Example driver code to test functions with Container strov. container(a) front(a) backa) etc ): (e.g., at(a,i), construct.container(a), at, de- a
Example driver code to test functions with Container strov. container(a) front(a) backa) etc ): (e.g., at(a,i), construct.container(a), at, de- a
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:下午1:15 11月15日周一
* 92%
+: 0
YYY
Example driver code to test functions with Container a (e.g., at(a,i), construct.container(a), at, de-
stroy_container(a), front(a), back(a), etc.):
1 int main () {
2
Container a, b;
3
std::cout << "Container a, b;\n\n";
4
std::cout << "construct_container (a,5 ,0);";
construct_container (a, 5, 0) ;
std::cout << "\na = ";
for (int i = 0; i < size (a); i++)
std:: cout << at (a, i) << " ";
5
6
8
9
std::cout « "\nsize(a) is " << size (a);
std::cout << "\ncapacity (a) is " << capacity (a);
10
11
12
13
14
15
std::cout << "\n\n//add elements to a\n";
at (a, 0) = 10;
16
17
18
19
at (a, 3) = 5;
std::cout << "at(a,0) = 10;\n";
std::cout << "at (a,3) = 5;";
std::cout << "\na = ";
for (int i = 0; i < size (a); i++)
std:: cout << at (a, i) << " ";
20
21
int& cFront = front (a);
std::cout << "\n\nfront (a) = "
int& cBack = back (a);
std::cout << "\nback (a)
22
23
<< cFront;
24
<< cBack << "\n";
25
26
27
28
29
destroy_container (a);
std::cout « "\n//destroy container a\n";
std::cout <« "destroy container (a);";
30
31
std::cout << "\n\n0ptional exception handling:";
32
try {
33
std:: cout << "\nfront (a) = ":
std:: cout <« front (a);
34
35
}
36
37
catch (std :: out_of_range e) { std :: cerr << e.what (); }
38
39
40
try {
std:: cout << "\nat (a,9) = ":
std:: cout << at (a, 9);
41
42
43
}
catch (std: : string msg){std::cerr « "\n" << msg << std: : endl;}
44
45
std::cout <<
"\n\n-
46
// Create confidence tests for Container b as shown below
47
48 }
2021 © Scott Bishop | Assistant Professor of Computer Science, Santa Monica College
3

Transcribed Image Text:2. A struct Container with member variables size, capacity, and data.
1 struct Container{
2
int size
= 0;
3
int capacity = 0;
int *data
= nullptr;
4
5 };
1
3. Implement the Container Functions shown below.
1 // Construct a Container c with a
size s
and initial value val.
2 // Defaults are zero.
3 void construct_container( Container& c,
int s = 0, int val= 0 );
4
5 // Destroy Container c and return memory to the freestore (heap).
6 void destroy_container ( Container& c );
7
8 // Returns pointer to the first element in Container c.
9 int* data ( const Container& c );
10
11 // Returns the number of elements in Container c.
12 int size ( const Container& c);
13
14 // Returns a reference
15 // (optional) Throws std::string exception if out of bounds
16 int& at ( Container& c, int i);
17
to the element at location i in Container v.
18 // Returns a reference to
the last element in Container c.
19 // (optional) Throws std::out-of_range exception if Container is empty
20 int& back ( const Container& c );
21
22 // Returns the allocated storage for Container
C.
23 int capacity ( const Container& c );
24
25 // Erases the elements of Container
but does not change capacity.
26 void clear ( Container& c);
27
28 // If Container c is empty return true, else false.
29 bool e mpty ( const Container& c);
30
31 // Returns a reference to the first element in the Container.
32 // (optional) Throws exception if Container is empty.
33 int& front ( const
Container & c );
34
35 // Deletes the last element of Container .
36 void pop_back ( Container& c );
37
38 // Add element to the end of the Container c.
39 void push_back ( Container& c, int element);
40
41 // Search for a key in Container c,
42 //return index of key or -1 if not found
43 int find key (Container &c, int key);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
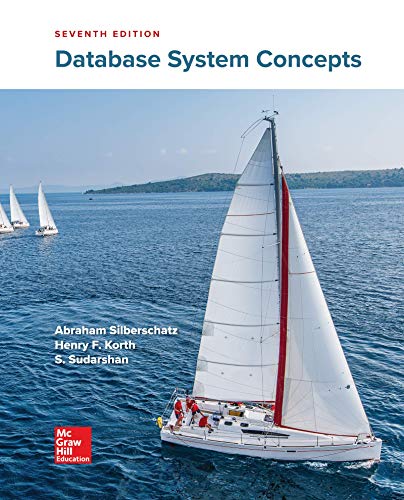
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
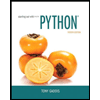
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
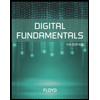
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
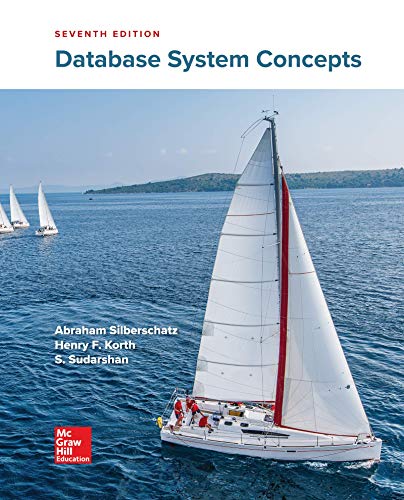
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
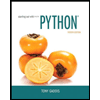
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
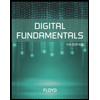
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
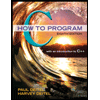
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
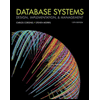
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
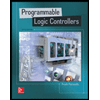
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education