package polynomials /* * Your tasks (indicated with 'Task X' below): Task 1: • Implement mutable internal state (=current value) for Variables. • Complete the 'set' and 'value' methods in class Variable, and the 'value' method in class Constant. Task 2: • Implement polynomial value computation using structural recursion. * Complete the 'value' methods in all the classes extending Polynomial. Hint: you may want to take a look at how the method 'asString' is implemented. + Task 3: + Implement programmer-defined operators to ease working with Polynomial objects. That is, complete the methods and '+' in class Polynomial so that you may use the operators and '+' to construct Product and Sum objects from Polynomial objects. For more on operators in Scala, see here http://docs.scala-lang.org/tutorials/tour/operators.html
package polynomials /* * Your tasks (indicated with 'Task X' below): Task 1: • Implement mutable internal state (=current value) for Variables. • Complete the 'set' and 'value' methods in class Variable, and the 'value' method in class Constant. Task 2: • Implement polynomial value computation using structural recursion. * Complete the 'value' methods in all the classes extending Polynomial. Hint: you may want to take a look at how the method 'asString' is implemented. + Task 3: + Implement programmer-defined operators to ease working with Polynomial objects. That is, complete the methods and '+' in class Polynomial so that you may use the operators and '+' to construct Product and Sum objects from Polynomial objects. For more on operators in Scala, see here http://docs.scala-lang.org/tutorials/tour/operators.html
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:1
2
3
4
5 package polynomials
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
// Scala 3 (DO NOT EDIT OR REMOVE THIS LINE!!!)
/*
* Your tasks (indicated with 'Task X' below):
*
* Task 1:
* Implement mutable internal state (=current value) for Variables.
Complete the 'set' and 'value' methods in class Variable,
* and the 'value' method in class Constant.
* Task 2:
* Implement polynomial value computation using structural recursion.
* Complete the 'value methods in all the classes extending Polynomial.
* Hint: you may want to take a look at how the method 'asString'
* is implemented.
* Task 3:
* Implement programmer-defined operators to ease working with Polynomial
* objects. That is, complete the methods '*' and '+' in class Polynomial
* so that you may use the operators '*' and '+' to construct Product and
* Sum objects from Polynomial objects. For more on operators in Scala,
see here
*
* http://docs.scala-lang.org/tutorials/tour/operators.html
*/
/** The abstract base class for polynomials. */
TRATSIRRO FER
SRINI
.
다
FEAT.
NOTEM NE
NA KABABA

Transcribed Image Text:35 abstract class Polynomial():
mm
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
70
71
def value: Int
// implemented by extending classes
def asString: String // implemented by extending classes
72 73 74
def +(right: Polynomial): Polynomial = ??? // Task 3
// sum of left (this) and right
def *(right: Polynomial): Polynomial = ??? // Task 3
// product of left (this) and right
end Polynomial
/** An extending class that implements polynomial variables. */
class Variable (name: String) extends Polynomial():
// Task 1
// ??? record current value of this variable somewhere here
def set(new_val: Int) = { ??? } // set the value of this variable
↑ value
|_def value = ???
↑asString
def asString = name
end Variable
/** An extending class that implements polynomial constants. */
class Constant (v: Int) extends Polynomial():
↑ value
def value = ??? // Task 1
↑ asString
def asString = v.toString
end Constant
65
66
67
*/
68 /** An extending class that implements polynomials that are the product of two polynomials. *
69 class Product(left: Polynomial, right: Polynomial) extends Polynomial():
↑ value
def value = ??? // Task 2
↑asString
def asString = "(" ++ left.asString ++ "*" ++ right.asString ++ ")"
// return current value of this variable
/** An extending class that implements polynomials that are the sum of two polynomials. */
class Sum(left: Polynomial, right: Polynomial) extends Polynomial():
↑ value
def value = ??? // Task 2
↑asString
def asString = "(" ++ left.asString ++ "+" ++ right.asString ++ ")"
end Sum
end Product
S
BEOG
REPARAR
CASINE GRATILI ALLA
MATATY
SUKIER BLE TABLES
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
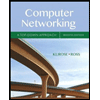
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
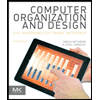
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
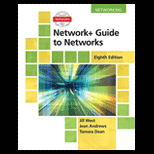
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
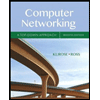
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
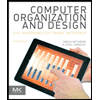
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
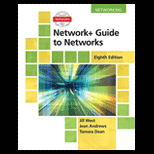
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
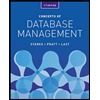
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
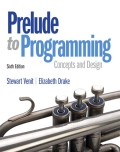
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
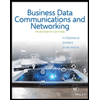
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY