Draw a UML class diagram for the following code: class Node: def __init__(self, value): self.value = value self.next = None class Stack: def __init__(self): self.top = None def isEmpty(self): return self.top is None def push(self, item): new_node = Node(item) new_node.next = self.top self.top = new_node def pop(self): if self.isEmpty(): raise Exception("Stack is empty") popped_item = self.top.value self.top = self.top.next return popped_item def popAll(self): items = [] while not self.isEmpty(): items.append(self.pop()) return items[::-1] def peek(self): if self.isEmpty(): raise Exception("Stack is empty") return self.top.value # Verification stack = Stack() print("Is stack empty?", stack.isEmpty()) stack.push(10) stack.push(20) stack.push(30) print("Top item:", stack.peek()) print("Popped item:", stack.pop()) print("Popped items:", stack.popAll()) print("Is stack empty?", stack.isEmpty())
Draw a UML class diagram for the following code:
class Node:
def __init__(self, value):
self.value = value
self.next = None
class Stack:
def __init__(self):
self.top = None
def isEmpty(self):
return self.top is None
def push(self, item):
new_node = Node(item)
new_node.next = self.top
self.top = new_node
def pop(self):
if self.isEmpty():
raise Exception("Stack is empty")
popped_item = self.top.value
self.top = self.top.next
return popped_item
def popAll(self):
items = []
while not self.isEmpty():
items.append(self.pop())
return items[::-1]
def peek(self):
if self.isEmpty():
raise Exception("Stack is empty")
return self.top.value
# Verification
stack = Stack()
print("Is stack empty?", stack.isEmpty())
stack.push(10)
stack.push(20)
stack.push(30)
print("Top item:", stack.peek())
print("Popped item:", stack.pop())
print("Popped items:", stack.popAll())
print("Is stack empty?", stack.isEmpty())

Step by step
Solved in 3 steps with 1 images

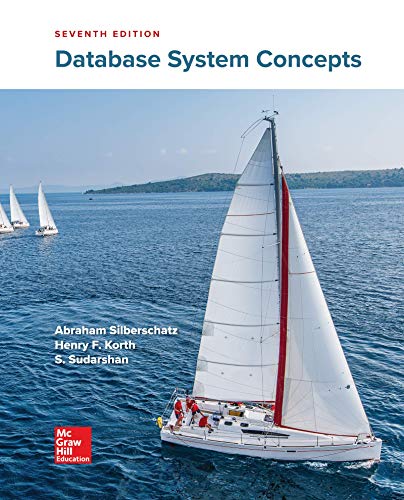
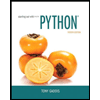
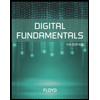
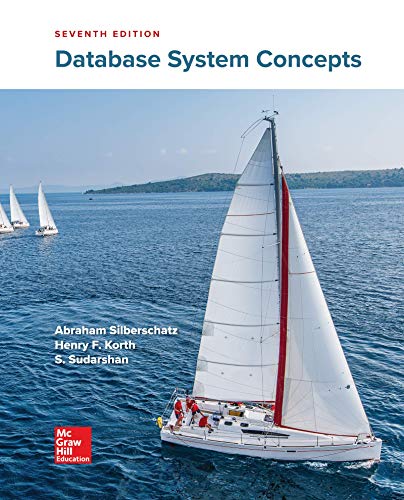
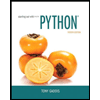
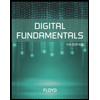
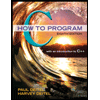
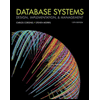
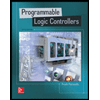