/** Represents a car. */ public class Car // TODO: Inherit from Vehicle { // Do NOT add any instance variables public Car(double purchasePrice) { // TODO: Complete } // TODO: Override the getValue method
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
/**
Represents a car.
*/
public class Car // TODO: Inherit from Vehicle
{
// Do NOT add any instance variables
public Car(double purchasePrice)
{
// TODO: Complete
}
// TODO: Override the getValue method
![## Java Programming Example: Car and Vehicle Classes
### CarTester.java
This is a Java program that tests vehicle functionality.
```java
public class CarTester
{
public static void main(String[] args)
{
Vehicle myCar = new Car(20000);
System.out.println(myCar.getValue());
System.out.println("Expected: 20000.0");
myCar.move(10000);
System.out.println(myCar.getValue());
System.out.println("Expected: 17500.0");
myCar.move(30000);
System.out.println(myCar.getValue());
System.out.println("Expected: 10000.0");
myCar.move(40000);
System.out.println(myCar.getValue());
System.out.println("Expected: 0.0");
myCar.move(10000);
System.out.println(myCar.getValue());
System.out.println("Expected: 0.0");
}
}
```
### Vehicle.java
This class represents a vehicle's basic properties and behaviors.
```java
/**
* Represents a vehicle of any type.
*/
public class Vehicle
{
private double value;
private double mileage;
public Vehicle(double aValue)
{
value = aValue;
mileage = 0;
}
public void move(double milesMoved)
{
mileage = mileage + milesMoved;
}
public double getValue()
{
return value;
}
public double getMileage()
{
return mileage;
}
}
```
### Explanation
In this example, there are two Java files:
#### 1. CarTester.java
- **Purpose**: Tests the functionality of a `Vehicle` (or `Car`) object.
- **Functionality**:
- Creates a new `Car` with an initial value of 20,000.
- Prints the car's value and performs a series of movements, reducing the car's value each time.
- Expects the car's value to be displayed and checked against expected values after each movement.
#### 2. Vehicle.java
- **Purpose**: Defines a generic vehicle with attributes such as `value` and `mileage`.
- **Attributes**:
- `value` (double): Initial worth of the vehicle.
- `mileage` (double): Distance the vehicle has traveled.
- **Methods**:
- `move(double milesMoved)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1580620b-ec3d-48da-8d5f-e0e1d6dd1757%2F38a8a228-fbb4-4fba-bbee-f650561d02ae%2Fit6v15_processed.png&w=3840&q=75)

Description:
In the constructor of Car class, call the superclass constructor with the purchasePrice as the argument.
- In the getValue function, do the following:
- Initialize a variable.
- Set the value of the variable to super.getValue() - ((super.getMileage() / 10000) * 2500).
- If the value of the variable is less than 0, set its value to 0.0.
- Return the value of the variable.
Implementation of the Car class is given in the next step.
Step by step
Solved in 3 steps with 1 images

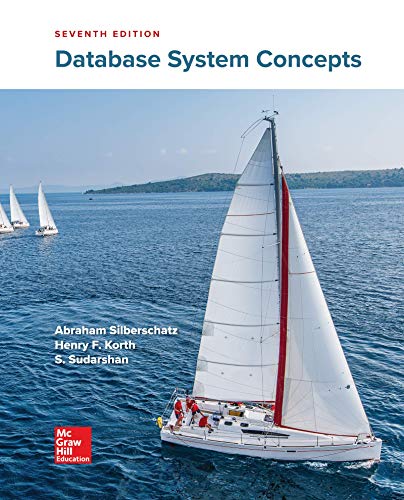
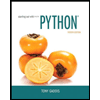
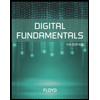
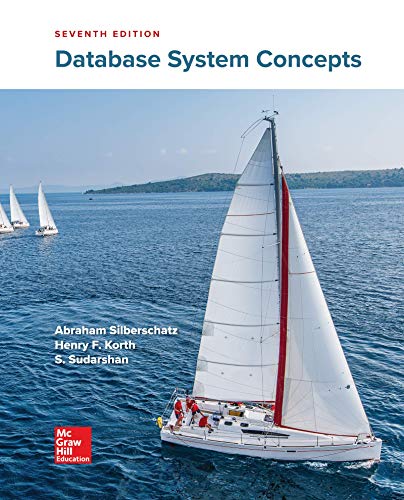
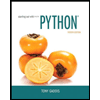
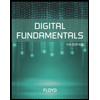
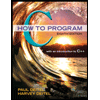
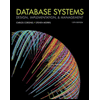
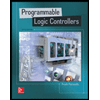