PREPARE A UML DIAGRAM FOR THE CODE public class Customer { private List orderList = new ArrayList<>(); boolean isPremium; boolean isDue = true; String paymentMethod = "card"; int ID = 0; int numCD = 0; int numDVD = 0; int numBook = 0; public Customer(int ID, int numCD, int numDVD, int numBook){ this.ID = ID; this.numCD = numCD; this.numDVD = numDVD; this.numBook = numBook; } public ProductOrder addOrder(ProductOrder newOrder){ this.orderList.add(newOrder); return newOrder; } public void checkout(){ for (ProductOrder order : orderList) { numCD += order.getCD(); numDVD += order.getDVD(); numBook += order.getBook(); } orderList.clear(); } public void printInventory(){ System.out.println("numDVDs : " + this.numDVD); System.out.println("numCDs : " + this.numCD); System.out.println("numBooks : " + this.numBook); } public int getBook(){ return numBook; } public void setBook(int numBook){ this.numBook = numBook; } public int getCD(){ return numCD; } public void setCD(int numCD){ this.numCD = numCD; } public int getDVD(){ return numDVD; } public void setDVD(int numDVD){ this.numDVD = numDVD; } public int getProductTotal(){ return getBook()*10 + getCD()*15 + getDVD()*20 + (this.isPremium?10:0); } public int getID(){ return ID; } }
PREPARE A UML DIAGRAM FOR THE CODE
public class Customer {
private List<ProductOrder> orderList = new ArrayList<>();
boolean isPremium;
boolean isDue = true;
String paymentMethod = "card";
int ID = 0;
int numCD = 0;
int numDVD = 0;
int numBook = 0;
public Customer(int ID, int numCD, int numDVD, int numBook){
this.ID = ID;
this.numCD = numCD;
this.numDVD = numDVD;
this.numBook = numBook;
}
public ProductOrder addOrder(ProductOrder newOrder){
this.orderList.add(newOrder);
return newOrder;
}
public void checkout(){
for (ProductOrder order : orderList) {
numCD += order.getCD();
numDVD += order.getDVD();
numBook += order.getBook();
}
orderList.clear();
}
public void printInventory(){
System.out.println("numDVDs : " + this.numDVD);
System.out.println("numCDs : " + this.numCD);
System.out.println("numBooks : " + this.numBook);
}
public int getBook(){
return numBook;
}
public void setBook(int numBook){
this.numBook = numBook;
}
public int getCD(){
return numCD;
}
public void setCD(int numCD){
this.numCD = numCD;
}
public int getDVD(){
return numDVD;
}
public void setDVD(int numDVD){
this.numDVD = numDVD;
}
public int getProductTotal(){
return getBook()*10 + getCD()*15 + getDVD()*20 + (this.isPremium?10:0);
}
public int getID(){
return ID;
}
}

Step by step
Solved in 2 steps with 1 images

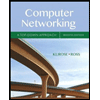
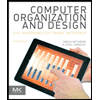
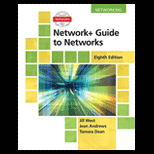
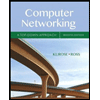
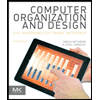
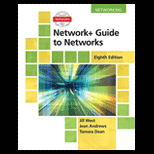
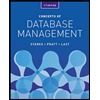
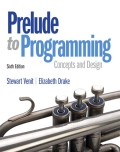
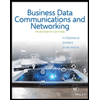