import javax.swing.*; import java.util.ArrayList; import java.util.Collections; import java.util.Random; import java.awt.*; import java.awt.event.*; public class memory extends JFrame implements ActionListener { private JButton[] cards; private ImageIcon[] icons; private int[] iconIDs; private JButton firstButton; private ImageIcon firstIcon; private int numMatches; public memory() { setTitle("Memory Matching Game"); setSize(800, 600); setLayout(new BorderLayout()); JPanel boardPanel = new JPanel(new GridLayout(4, 4)); add(boardPanel, BorderLayout.CENTER); icons = new ImageIcon[8]; for (int i = 1; i <= 8; i++) { icons[i-1] = new ImageIcon("image" + i + ".png"); } iconIDs = new int[16]; for (int i = 0; i < 8; i++) { iconIDs[2*i] = i; iconIDs[2*i+1] = i; } Random rand = new Random(); for (int i = 0; i < 16; i++) { int index = rand.nextInt(16); int temp = iconIDs[i]; iconIDs[i] = iconIDs[index]; iconIDs[index] = temp; } cards = new JButton[16]; for (int i = 0; i < 16; i++) { cards[i] = new JButton(); cards[i].setPreferredSize(new Dimension(150, 150)); cards[i].addActionListener(this); boardPanel.add(cards[i]); } resetGame(); } private void resetGame() { numMatches = 0; firstButton = null; firstIcon = null; for (int i = 0; i < 16; i++) { cards[i].setEnabled(true); cards[i].setIcon(null); } for (int i = 0; i < 16; i++) { int id = iconIDs[i]; cards[i].putClientProperty("id", id); } } private void handleCardClick(JButton button) { int id = (int) button.getClientProperty("id"); ImageIcon icon = icons[id]; if (firstIcon == null) { // first card clicked firstIcon = icon; firstButton = button; button.setIcon(icon); button.setEnabled(false); } else { // second card clicked if (icon == firstIcon) { // matching cards numMatches++; button.setIcon(icon); button.setEnabled(false); firstButton = null; firstIcon = null; } else { // non-matching cards firstButton.setEnabled(true); firstButton.setIcon(null); firstButton = null; firstIcon = null; button.setIcon(null); button.setEnabled(false); } } if (numMatches == 8) { // game over int choice = JOptionPane.showConfirmDialog(this, "Congratulations, you won!\nDo you want to play again?", "Game Over", JOptionPane.YES_NO_OPTION); if (choice == JOptionPane.YES_OPTION) { resetGame(); } else { System.exit(0); } } } @Override public void actionPerformed(ActionEvent e) { JButton button = (JButton) e.getSource(); handleCardClick(button); } public static void main(String[] args) { memory game = new memory(); game.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } please tell me why my code wont execute but it has no errrors and how I might fix the issue.
import javax.swing.*; import java.util.ArrayList; import java.util.Collections; import java.util.Random; import java.awt.*; import java.awt.event.*; public class memory extends JFrame implements ActionListener { private JButton[] cards; private ImageIcon[] icons; private int[] iconIDs; private JButton firstButton; private ImageIcon firstIcon; private int numMatches; public memory() { setTitle("Memory Matching Game"); setSize(800, 600); setLayout(new BorderLayout()); JPanel boardPanel = new JPanel(new GridLayout(4, 4)); add(boardPanel, BorderLayout.CENTER); icons = new ImageIcon[8]; for (int i = 1; i <= 8; i++) { icons[i-1] = new ImageIcon("image" + i + ".png"); } iconIDs = new int[16]; for (int i = 0; i < 8; i++) { iconIDs[2*i] = i; iconIDs[2*i+1] = i; } Random rand = new Random(); for (int i = 0; i < 16; i++) { int index = rand.nextInt(16); int temp = iconIDs[i]; iconIDs[i] = iconIDs[index]; iconIDs[index] = temp; } cards = new JButton[16]; for (int i = 0; i < 16; i++) { cards[i] = new JButton(); cards[i].setPreferredSize(new Dimension(150, 150)); cards[i].addActionListener(this); boardPanel.add(cards[i]); } resetGame(); } private void resetGame() { numMatches = 0; firstButton = null; firstIcon = null; for (int i = 0; i < 16; i++) { cards[i].setEnabled(true); cards[i].setIcon(null); } for (int i = 0; i < 16; i++) { int id = iconIDs[i]; cards[i].putClientProperty("id", id); } } private void handleCardClick(JButton button) { int id = (int) button.getClientProperty("id"); ImageIcon icon = icons[id]; if (firstIcon == null) { // first card clicked firstIcon = icon; firstButton = button; button.setIcon(icon); button.setEnabled(false); } else { // second card clicked if (icon == firstIcon) { // matching cards numMatches++; button.setIcon(icon); button.setEnabled(false); firstButton = null; firstIcon = null; } else { // non-matching cards firstButton.setEnabled(true); firstButton.setIcon(null); firstButton = null; firstIcon = null; button.setIcon(null); button.setEnabled(false); } } if (numMatches == 8) { // game over int choice = JOptionPane.showConfirmDialog(this, "Congratulations, you won!\nDo you want to play again?", "Game Over", JOptionPane.YES_NO_OPTION); if (choice == JOptionPane.YES_OPTION) { resetGame(); } else { System.exit(0); } } } @Override public void actionPerformed(ActionEvent e) { JButton button = (JButton) e.getSource(); handleCardClick(button); } public static void main(String[] args) { memory game = new memory(); game.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } } please tell me why my code wont execute but it has no errrors and how I might fix the issue.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
import javax.swing.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Random;
import java.awt.*;
import java.awt.event.*;
public class memory extends JFrame implements ActionListener {
private JButton[] cards;
private ImageIcon[] icons;
private int[] iconIDs;
private JButton firstButton;
private ImageIcon firstIcon;
private int numMatches;
public memory() {
setTitle("Memory Matching Game");
setSize(800, 600);
setLayout(new BorderLayout());
JPanel boardPanel = new JPanel(new GridLayout(4, 4));
add(boardPanel, BorderLayout.CENTER);
icons = new ImageIcon[8];
for (int i = 1; i <= 8; i++) {
icons[i-1] = new ImageIcon("image" + i + ".png");
}
iconIDs = new int[16];
for (int i = 0; i < 8; i++) {
iconIDs[2*i] = i;
iconIDs[2*i+1] = i;
}
Random rand = new Random();
for (int i = 0; i < 16; i++) {
int index = rand.nextInt(16);
int temp = iconIDs[i];
iconIDs[i] = iconIDs[index];
iconIDs[index] = temp;
}
cards = new JButton[16];
for (int i = 0; i < 16; i++) {
cards[i] = new JButton();
cards[i].setPreferredSize(new Dimension(150, 150));
cards[i].addActionListener(this);
boardPanel.add(cards[i]);
}
resetGame();
}
private void resetGame() {
numMatches = 0;
firstButton = null;
firstIcon = null;
for (int i = 0; i < 16; i++) {
cards[i].setEnabled(true);
cards[i].setIcon(null);
}
for (int i = 0; i < 16; i++) {
int id = iconIDs[i];
cards[i].putClientProperty("id", id);
}
}
private void handleCardClick(JButton button) {
int id = (int) button.getClientProperty("id");
ImageIcon icon = icons[id];
if (firstIcon == null) {
// first card clicked
firstIcon = icon;
firstButton = button;
button.setIcon(icon);
button.setEnabled(false);
} else {
// second card clicked
if (icon == firstIcon) {
// matching cards
numMatches++;
button.setIcon(icon);
button.setEnabled(false);
firstButton = null;
firstIcon = null;
} else {
// non-matching cards
firstButton.setEnabled(true);
firstButton.setIcon(null);
firstButton = null;
firstIcon = null;
button.setIcon(null);
button.setEnabled(false);
}
}
if (numMatches == 8) {
// game over
int choice = JOptionPane.showConfirmDialog(this, "Congratulations, you won!\nDo you want to play again?", "Game Over", JOptionPane.YES_NO_OPTION);
if (choice == JOptionPane.YES_OPTION) {
resetGame();
} else {
System.exit(0);
}
}
}
@Override
public void actionPerformed(ActionEvent e) {
JButton button = (JButton) e.getSource();
handleCardClick(button);
}
public static void main(String[] args) {
memory game = new memory();
game.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
please tell me why my code wont execute but it has no errrors and how I might fix the issue.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
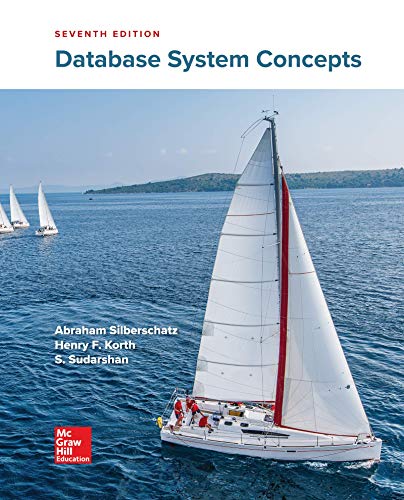
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
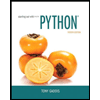
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
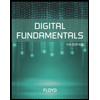
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
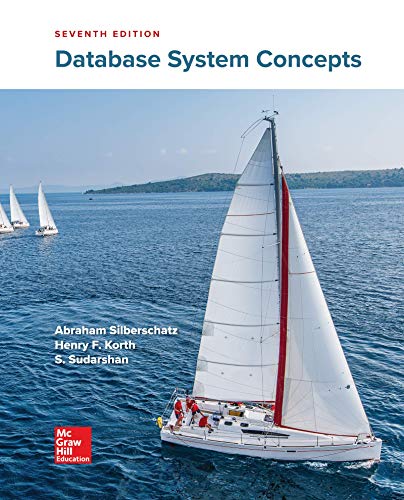
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
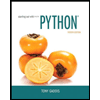
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
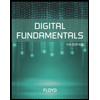
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
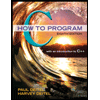
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
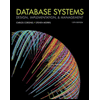
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
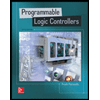
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education