6. In the Driver.java class, modify the main method. In main(), you must create a menu loop that does the following: • Displays the menu by calling the displayMenu method. This method is in the Driver.java class. o Prompts the user for input • Takes the appropriate action based on the value that the user entered IMPORTANT: You do not need to complete all of the methods included in the menu for this milestone. Simple placeholder print statements for these methods have been included in the starter code so that you can test your menu functionality. 7. Next, you will need to create a monkey ArrayList in the Driver.java class. Refer to the dog ArrayList, which is included right before main(), as an example. Creating this ArrayList is necessary for the intakeNewMonkey() method, which you will implement in the next step. Though it is not required, it may be helpful to pre-populate your ArrayList with a few test monkey objects in the initializeMonkeyList() method. 8. Finally, you will implement the intakeNewMonkey() method in the Driver.java class. Your completed method should do the following: o Prompt the user for input. o Set data for all attributes based on user input. • Add the newly instantiated monkey to an ArrayList.
6. In the Driver.java class, modify the main method. In main(), you must create a menu loop that does the following: • Displays the menu by calling the displayMenu method. This method is in the Driver.java class. o Prompts the user for input • Takes the appropriate action based on the value that the user entered IMPORTANT: You do not need to complete all of the methods included in the menu for this milestone. Simple placeholder print statements for these methods have been included in the starter code so that you can test your menu functionality. 7. Next, you will need to create a monkey ArrayList in the Driver.java class. Refer to the dog ArrayList, which is included right before main(), as an example. Creating this ArrayList is necessary for the intakeNewMonkey() method, which you will implement in the next step. Though it is not required, it may be helpful to pre-populate your ArrayList with a few test monkey objects in the initializeMonkeyList() method. 8. Finally, you will implement the intakeNewMonkey() method in the Driver.java class. Your completed method should do the following: o Prompt the user for input. o Set data for all attributes based on user input. • Add the newly instantiated monkey to an ArrayList.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Need help with menu loop please

Transcribed Image Text:6. In the Driver.java class, modify the main method. In `main()`, you must **create a menu loop** that does the following:
- Displays the menu by calling the `displayMenu` method. This method is in the Driver.java class.
- Prompts the user for input.
- Takes the appropriate action based on the value that the user entered.
**IMPORTANT**: You do not need to complete all of the methods included in the menu for this milestone. Simple placeholder print statements for these methods have been included in the starter code so that you can test your menu functionality.
7. Next, you will need to **create a monkey `ArrayList`** in the Driver.java class. Refer to the dog `ArrayList`, which is included right before `main()`, as an example. Creating this `ArrayList` is necessary for the `intakeNewMonkey()` method, which you will implement in the next step. Though it is not required, it may be helpful to pre-populate your `ArrayList` with a few test monkey objects in the `initializeMonkeyList()` method.
8. Finally, you will **implement the `intakeNewMonkey()` method** in the Driver.java class. Your completed method should do the following:
- Prompt the user for input.
- Set data for all attributes based on user input.
- Add the newly instantiated monkey to an `ArrayList`.
![```java
import java.util.ArrayList;
public class Driver {
private static final String inServiceCountry = null;
private static ArrayList<Dog> dogList = new ArrayList<Dog>();
// monkey array list
private static ArrayList<Monkey> monkeyList = new ArrayList<Monkey>();
// Instance variables (if needed)
public static void main(String[] args) {
initializeDogList();
initializeMonkeyList();
// Add a loop that displays the menu, accepts the users input
// and takes the appropriate action.
// For the project submission you must also include input validation
// and appropriate feedback to the user.
// Hint: create a Scanner and pass it to the necessary
// methods
// Hint: Menu options 4, 5, and 6 should all connect to the printAnimals() method.
}
}
```
### Explanation
- **Import Statement**: The code begins with an import statement for `ArrayList`, which is a part of the Java Collections Framework and is used to create resizable arrays.
- **Class Definition**: The public class `Driver` is defined, which contains static variables and methods.
- **Static Variables**:
- `inServiceCountry`: A static final string, which is initialized to `null`. This might be used later to define a country related to service operations.
- `dogList`: An `ArrayList` specifically for storing `Dog` objects.
- `monkeyList`: An `ArrayList` for storing `Monkey` objects. This is remarked as a monkey array list in comments.
- **Main Method**:
- `initializeDogList()`: A method call to initialize the dog list, though the method itself isn't defined here.
- `initializeMonkeyList()`: Similarly, this method call initializes the monkey list.
- **Comments for Further Implementation**:
- There are instructions to add a loop for a menu that accepts user input and performs appropriate actions based on the input.
- Input validation and user feedback are highlighted as necessary features for project submission.
- A `Scanner` should be created for input handling.
- Suggestions are made that menu options 4, 5, and 6 should link to a method named `printAnimals()`, though the method is not shown.
This code serves as a starting point for building an application that likely manages lists of animals with options for](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffa5f2882-5062-4aed-aa87-941121be8da3%2Fe77e8fca-ba2e-4c86-bbf4-4d5b9d479eb6%2Fwczkcwj_processed.png&w=3840&q=75)
Transcribed Image Text:```java
import java.util.ArrayList;
public class Driver {
private static final String inServiceCountry = null;
private static ArrayList<Dog> dogList = new ArrayList<Dog>();
// monkey array list
private static ArrayList<Monkey> monkeyList = new ArrayList<Monkey>();
// Instance variables (if needed)
public static void main(String[] args) {
initializeDogList();
initializeMonkeyList();
// Add a loop that displays the menu, accepts the users input
// and takes the appropriate action.
// For the project submission you must also include input validation
// and appropriate feedback to the user.
// Hint: create a Scanner and pass it to the necessary
// methods
// Hint: Menu options 4, 5, and 6 should all connect to the printAnimals() method.
}
}
```
### Explanation
- **Import Statement**: The code begins with an import statement for `ArrayList`, which is a part of the Java Collections Framework and is used to create resizable arrays.
- **Class Definition**: The public class `Driver` is defined, which contains static variables and methods.
- **Static Variables**:
- `inServiceCountry`: A static final string, which is initialized to `null`. This might be used later to define a country related to service operations.
- `dogList`: An `ArrayList` specifically for storing `Dog` objects.
- `monkeyList`: An `ArrayList` for storing `Monkey` objects. This is remarked as a monkey array list in comments.
- **Main Method**:
- `initializeDogList()`: A method call to initialize the dog list, though the method itself isn't defined here.
- `initializeMonkeyList()`: Similarly, this method call initializes the monkey list.
- **Comments for Further Implementation**:
- There are instructions to add a loop for a menu that accepts user input and performs appropriate actions based on the input.
- Input validation and user feedback are highlighted as necessary features for project submission.
- A `Scanner` should be created for input handling.
- Suggestions are made that menu options 4, 5, and 6 should link to a method named `printAnimals()`, though the method is not shown.
This code serves as a starting point for building an application that likely manages lists of animals with options for
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
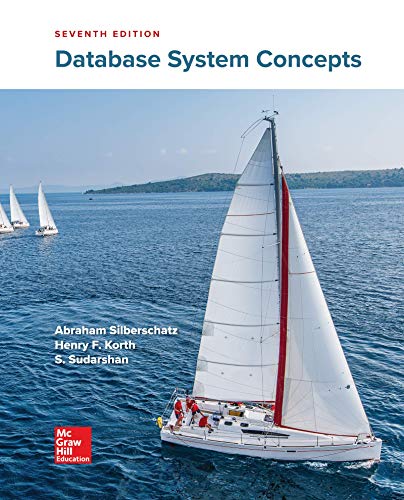
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
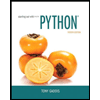
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
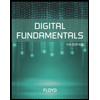
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
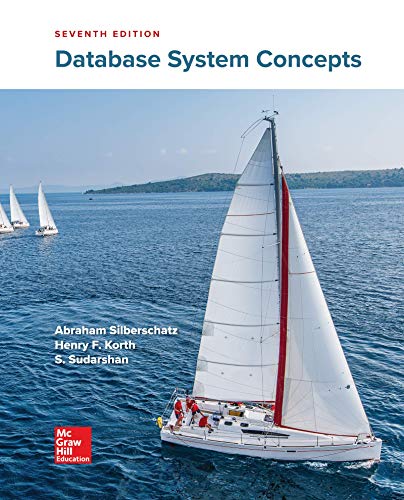
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
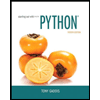
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
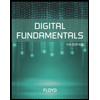
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
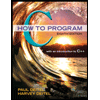
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
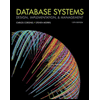
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
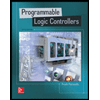
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education