In this lab you will complete a program that reads students' name and score and displays students' name, score and letter grade that is calculated based on the above table. Each line of output corresponding to an honor student should be marked by a *. Given classes: • Class LabProgram contains the main method for testing the program. • Class Course represents a course, which contains an ArrayList of Student objects as a course roster. • Class Student represents a regular student, which has three fields: first name, last name, and score. • Class HonorStudent is derived from the class Student and represents an honor student. This class has a constructor and overrides methods getGrade and toString of its super class.
import java.util.Scanner;
import java.util.ArrayList;
public class LabProgram {
public static void main(String[] args) {
Course cop3804=new Course();
String fn, ln;
int score;
String studentType;
Scanner kb=new Scanner(System.in);
studentType=kb.next();
while (!studentType.equals("q")) {
fn = kb.next();
ln = kb.next();
score=kb.nextInt();
if (studentType.equals("R"))
cop3804.addStudent(new Student(fn, ln, score));
else
cop3804.addStudent(new HonorStudent(fn, ln, score));
studentType = kb.next();
}
cop3804.print();
}
}
import java.util.ArrayList;
public class Course {
private ArrayList<Student> roster; //collection of Student objects
public Course() {
roster = new ArrayList<Student>();
}
public void addStudent(Student s) {
roster.add(s);
}
public void print(){
/* displays the class roster and student grades */
/* Type your code here */
}
}
import java.text.DecimalFormat;
// Class representing a student
public class Student {
private String first;
private String last;
private int score;
public Student (String f, String l, int score) {
first=f;
last=l;
this.score=score;
}
public void setScore(int score) {
this.score=score;
}
public int getScore() {
return (score);
}
public char getGrade() {
/* returns student's grade */
/* Type your code here */
}
@Override
public String toString() {
return (String.format("%-10s %-10s %3d", first, last, score));
}
}
public class HonorStudent extends Student{
public HonorStudent (String f, String l, int score) {
/* HonorStudent constructor */
/* Type your code here */
}
@Override
public char getGrade() {
/* returns honor student's letter grade */
/* Type your code here */
}
@Override
public String toString() {
/* returns honor student's information followed by a "*" */
/* Hint. concatendate "*" to the end of the string returned */
/* by the toString function of the super class */
/* Type your code here */
}
}



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

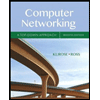
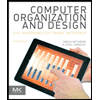
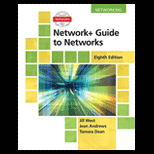
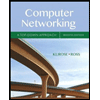
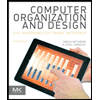
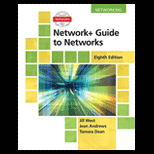
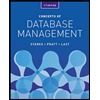
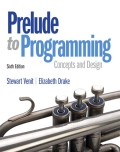
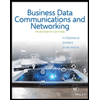