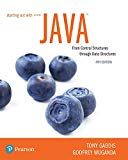
Explanation of Solution
Step 1: Define the method name “contains ()” which contains the node and search element as the parameters.
Step 2: Check if the value of node is equal to null. If it is equal, then return “false”.
Step 3: Check if the value of node is equal to the search element. If this condition is true, then return “true”.
Step 4: Again check if the search element is present in the left side of the tree by calling the function “contains ()” recursively and return “true” if the search element is present.
Step 5: Again check if the search element is present in the right side of the tree by calling the function “contains ()” recursively and return “true” if the search element is present.
Step 6: Finally, if the search element is not present, then return “false”.
A method “contains” to search a value of “x”:
//Function definition for "contains"
boolean contains(Node binarytree, int x)
{
//Check if the value of node is equal to null
if (binarytree == null)
//Return false
return false;
//Check if the value of node is equal to the search element
if (binarytree.value == x)
//Return true
return true;
//Check if the search element is present in the left sub-tree
if (contains(binarytree...

Want to see the full answer?
Check out a sample textbook solution
Chapter 21 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- Use the recursive strategy described in the chapter to implement a binary tree. Each node in this method is a binary tree. Thus, a binary tree includes references to its left and right subtrees in addition to the element stored at its root. You could also wish to make mention of its progenitor.arrow_forwardUse the recursive strategy described in the chapter to implement a binary tree. Each node in this method is a binary tree. Thus, a binary tree includes references to its left and right subtrees in addition to the element stored at its root.You could also wish to make mention of its progenitor.arrow_forward1. A Binary Search Tree (BST) is a binary tree where each node contains a value from a well-ordered set. (a) Draw a BST for each of the following set of data: i. 20, 30, 45, 31, 19, 15, 18, 13, 50, 21 i. М, О, R, T, С, F, E, A, S, N, Qarrow_forward
- Given a pre-order traversal of a binary search tree(BST) and a range,say [x,y], write a program that constructs a binary tree with the giventraversal and then removes all the nodes for which the values are inthe given range maintaining the BST nature of the tree with necessarychanges/modifications. You may assume that the ‘pre-order’ traversalgiven initially as input is a valid one and need not verify that.Input: The input should be a ‘pre-order’ traversal of a binary search treeand two values which are the non-negative bounds of the range.Output: The output should be a the ‘pre-order’ traversal of the modifiedbinary tree with no nodes in that range. Write using C programming.arrow_forwardWrite a function, countNegativeNodes(TreeNode* p), that returns the number of nodes in a binary tree that have negative numbers as their node value. Use RECURSION.arrow_forwardwrite a java/c++ code or an algorithm to solve the following problem. After that dry run and show output of algorithm using an example binary tree. Without dry run no marks will be awarded. Write a recursive function/algorithm that finds the minimum value node from a Binary tree. (Caution: This is a simple binary tree not binary search tree i.e; tree is not in any order. So minimum node may be anywhere in tree.)arrow_forward
- Create a function that takes an array that represent a Binary Tree and a value and return true if the value is in the tree and, false otherwise. Examples valueInTree (arr1, 5) true valueInTree (arr1, 9) → false valueInTree (arr2, 51) → falsearrow_forwardUsing an in-order traversal it is quite easy to output all the data in a binary search tree in order. Design an algorithm which when given a binary search tree and two numbers x and y outputs all the data items z in order with the property that xarrow_forwardJava code neededarrow_forward
- } 20. In a binary search tree, the immediate predecessor of a given node is the largest node in its left subtree. For example, the immediate predecessor of node 25 in the following tree is 23 while it is 15 for node 16. Nodes 21 and 39 do not have an immediate predecessor because none of them has a left child. 25 / 1 Write a non-recursive method immediate Predecessor, which takes a BSTNode parameter node (a reference to a node in a binary search tree) and returns a reference to its immediate predecessor in the tree. If node is null or it does not have an immediate predecessor, the method returns null. public class BSTNode 4 { private int m_info; private BSTNode m_left; private BSTNode m_right; public int getInfo() {return m_info; } public void setInfo (int value) {m_info = info; } public BSTNode getLeft () {return m_left; } public void setLeft (BSTNode left) (m_left = left; } public BSTNode getRight () {return m_right;} public void setRight (BSTNode right) {m_right = right;} } public…arrow_forwardGiven a binary tree, let an H-node be defined as a non-leaf node in the tree whose value is greater than or equal to its children nodes (1 or 2 children). Write a function called countHNodes(TreeNode* p), that returns the number of H-nodes in a binary tree (pointed by p). Use recursion in the function.arrow_forward1. Give a recursive algorithm in pseudocode to compute the diameter of a binary tree. Recall that diameter is defined as the number of nodes on the longest path between any two nodes in the tree. Nodes have left and right references, but nothing else. You must use the height function, defined as follows, in your solution. Your solution will return the diameter of the tree as an integer. function height (Node n) 1. if n = null 2. return -1 3. return 1 + max (height (n.left), height (n.right)) Write your solution below. function diameter (Node n)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
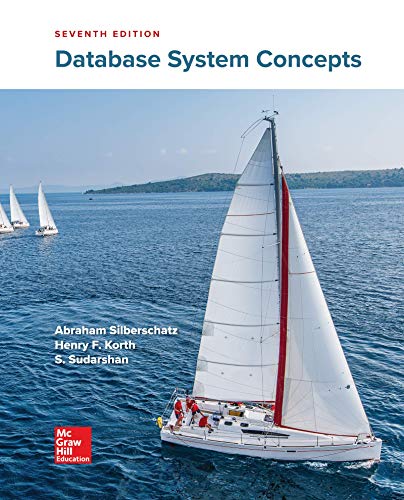
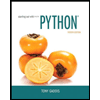
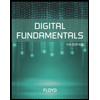
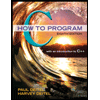
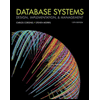
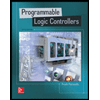