import statistics def find Min (scoreArray: list) -> float: return min (scoreArray) def findMax (score Array: list) -> float: return max (scoreArray) def getMean (scoreArray: list) -> float: return statistics.mean (scoreArray) def getMedian (scoreArray:list) -> float: return statistics.median (scoreArray) def getScores (): pass def saveScores (scores: list): pass def testFunctions () : ''Test for functions written above only remove comments for what you want to test''' test Scores = getScores () #Testing findMin print (f"Testing findmin: find Min should return 74 and returns (find Min (testScores)}") #Testing findMax print (f"Testing findMax: find Max should return 98 and returns (find Max (testScores)}") #Testing getMean print (f"Testing getMean: getMean should return a 85.25 and return {getMean (testScores)}") #Testing getMedian print (f"Testing getMedian: getMedian should return a 86.5 and returns {getMedian (testScores)}") testScores.pop (0) print (f"Testing getMedian: getMedian should return a 89 and returns (getMedian (testScores)}") saveScores (testScores) if name ' _main__': test Functions () Go back to our 1D array assignment where you found the max, min, mean, and median of a list. You are going to write 2 new functions to that program, getScores and saveScores. Function getScores does not require a parameter and will have you open a text file of scores called scores.txt, read in each score and append it to a list. You must return the list of scores created. Requirements for the function are listed below 1. The file must open in read only mode and close when finished 2. Contents of the file must be read in one at a time, converted to an integer, and appended to a list 3. The list must be returned from the function 4. Use only basic python structures, no list comprehensions or functions from other libraries Function saveScores will require a single list of integers as a parameter. It will save the list's contents to a text file named updatedScores.txt. It will write each score in the file as a single entry in each line of text. The updatedScores.txt should follow the same format at the scores.txt file
import statistics def find Min (scoreArray: list) -> float: return min (scoreArray) def findMax (score Array: list) -> float: return max (scoreArray) def getMean (scoreArray: list) -> float: return statistics.mean (scoreArray) def getMedian (scoreArray:list) -> float: return statistics.median (scoreArray) def getScores (): pass def saveScores (scores: list): pass def testFunctions () : ''Test for functions written above only remove comments for what you want to test''' test Scores = getScores () #Testing findMin print (f"Testing findmin: find Min should return 74 and returns (find Min (testScores)}") #Testing findMax print (f"Testing findMax: find Max should return 98 and returns (find Max (testScores)}") #Testing getMean print (f"Testing getMean: getMean should return a 85.25 and return {getMean (testScores)}") #Testing getMedian print (f"Testing getMedian: getMedian should return a 86.5 and returns {getMedian (testScores)}") testScores.pop (0) print (f"Testing getMedian: getMedian should return a 89 and returns (getMedian (testScores)}") saveScores (testScores) if name ' _main__': test Functions () Go back to our 1D array assignment where you found the max, min, mean, and median of a list. You are going to write 2 new functions to that program, getScores and saveScores. Function getScores does not require a parameter and will have you open a text file of scores called scores.txt, read in each score and append it to a list. You must return the list of scores created. Requirements for the function are listed below 1. The file must open in read only mode and close when finished 2. Contents of the file must be read in one at a time, converted to an integer, and appended to a list 3. The list must be returned from the function 4. Use only basic python structures, no list comprehensions or functions from other libraries Function saveScores will require a single list of integers as a parameter. It will save the list's contents to a text file named updatedScores.txt. It will write each score in the file as a single entry in each line of text. The updatedScores.txt should follow the same format at the scores.txt file
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 28SA
Related questions
Question
How do I answer this python coding question here? Code provided

Transcribed Image Text:import statistics
def find Min (scoreArray: list) -> float:
return min (scoreArray)
def findMax (score Array: list) -> float:
return max (scoreArray)
def getMean (scoreArray: list) -> float:
return statistics.mean (scoreArray)
def getMedian (scoreArray:list) -> float:
return statistics.median (scoreArray)
def getScores ():
pass
def saveScores (scores: list):
pass
def testFunctions () :
''Test for functions written above only remove comments for what you want to test'''
test Scores = getScores ()
#Testing findMin
print (f"Testing findmin: find Min should return 74 and returns (find Min (testScores)}")
#Testing findMax
print (f"Testing findMax: find Max should return 98 and returns (find Max (testScores)}")
#Testing getMean
print (f"Testing getMean: getMean should return a 85.25 and return {getMean (testScores)}")
#Testing getMedian
print (f"Testing getMedian: getMedian should return a 86.5 and returns {getMedian (testScores)}")
testScores.pop (0)
print (f"Testing getMedian: getMedian should return a 89 and returns (getMedian (testScores)}")
saveScores (testScores)
if
name
'
_main__':
test Functions ()

Transcribed Image Text:Go back to our 1D array assignment where you found the max, min, mean, and median of a list.
You are going to write 2 new functions to that program, getScores and saveScores.
Function getScores does not require a parameter and will have you open a text file of scores
called scores.txt, read in each score and append it to a list. You must return the list of scores
created. Requirements for the function are listed below
1. The file must open in read only mode and close when finished
2. Contents of the file must be read in one at a time, converted to an integer, and appended to
a list
3. The list must be returned from the function
4. Use only basic python structures, no list comprehensions or functions from other libraries
Function saveScores will require a single list of integers as a parameter. It will save the list's
contents to a text file named updatedScores.txt. It will write each score in the file as a single
entry in each line of text. The updatedScores.txt should follow the same format at the
scores.txt file
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Recommended textbooks for you
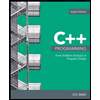
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
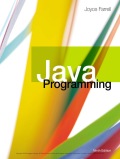
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
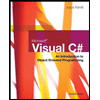
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
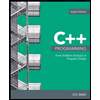
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
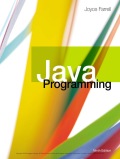
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
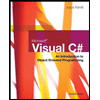
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
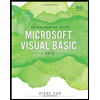
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
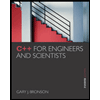
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage