Here is my code please draw a transition diagram and nfa on paper public class Lexer { private static final char EOF = 0; private static final int BUFFER_SIZE = 10; private Parser yyparser; // parent parser object private java.io.Reader reader; // input stream public int lineno; // line number public int column; // column // Double buffering implementation private char[] buffer1; private char[] buffer2; private boolean usingBuffer1; private int currentPos; private int bufferLength; private boolean endReached; // Keywords private static final String[] keywords = { "int", "print", "if", "else", "while", "void" }; public Lexer(java.io.Reader reader, Parser yyparser) throws Exception { this.reader = reader; this.yyparser = yyparser; this.lineno = 1; this.column = 0; // Initialize double buffering buffer1 = new char[BUFFER_SIZE]; buffer2 = new char[BUFFER_SIZE]; usingBuffer1 = true; currentPos = 0; bufferLength = 0; endReached = false; // Initial buffer fill fillBuffer(); } private void fillBuffer() throws Exception { char[] currentBuffer = usingBuffer1 ? buffer1 : buffer2; bufferLength = 0; while (bufferLength < BUFFER_SIZE) { int data = reader.read(); if (data == -1) { endReached = true; break; } currentBuffer[bufferLength++] = (char)data; } currentPos = 0; } public char NextChar() throws Exception { if (currentPos >= bufferLength) { if (endReached) { return EOF; } usingBuffer1 = !usingBuffer1; fillBuffer(); if (bufferLength == 0) { return EOF; } } char c = usingBuffer1 ? buffer1[currentPos] : buffer2[currentPos]; currentPos++; column++; if (c == '\n') { lineno++; column = 0; } return c; } public void UngetChar() { if (currentPos > 0) { currentPos--; column--; if (column < 0) { lineno--; // We don't know the previous line's length, so we'll set it to 0 column = 0; } } } public int Fail() { return -1; } private boolean isDigit(char c) { return c >= '0' && c <= '9'; } private boolean isLetter(char c) { return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z'); } private boolean isWhitespace(char c) { return c == ' ' || c == '\t' || c == '\n' || c == '\r'; } private String readIdentifier(char firstChar) throws Exception { StringBuilder sb = new StringBuilder(); sb.append(firstChar); while (true) { char c = NextChar(); if (isLetter(c) || isDigit(c) || c == '_') { sb.append(c); } else { UngetChar(); break; } } return sb.toString(); } private String readNumber(char firstDigit) throws Exception { StringBuilder sb = new StringBuilder(); sb.append(firstDigit); boolean hasDecimal = false; while (true) { char c = NextChar(); if (isDigit(c)) { sb.append(c); } else if (c == '.' && !hasDecimal) { hasDecimal = true; sb.append(c); } else { UngetChar(); break; } } return sb.toString(); } // * If yylex reach to the end of file, return 0 // * If there is a lexical error found, return -1 // * If a proper lexeme is determined, return token public int yylex() throws Exception { while (true) { char c = NextChar(); // Handle EOF if (c == EOF) { return 0; } // Skip whitespace if (isWhitespace(c)) { continue; } // Handle identifiers and keywords if (isLetter(c)) { String lexeme = readIdentifier(c); // Check if it's a keyword for (String keyword : keywords) { if (lexeme.equals(keyword)) { yyparser.yylval = new ParserVal((Object)lexeme); switch (keyword) { case "int": return Parser.INT; case "print": return Parser.PRINT; case "if": return Parser.IF; case "else": return Parser.ELSE; case "while": return Parser.WHILE; case "void": return Parser.VOID; } } } // Not a keyword, so it's an identifier yyparser.yylval = new ParserVal((Object)lexeme); return Parser.ID; } // Handle numbers if (isDigit(c)) { String number = readNumber(c); yyparser.yylval = new ParserVal((Object)number); return Parser.NUM; } // Handle operators and symbols switch (c) { case '(': yyparser.yylval = new ParserVal((Object)"("); return Parser.LPAREN; case ')': yyparser.yylval = new ParserVal((Object)")"); return Parser.RPAREN; case ';': yyparser.yylval = new ParserVal((Object)";"); return Parser.SEMI; case ',': yyparser.yylval = new ParserVal((Object)","); return Parser.COMMA; case '+': case '-': case '*': case '/': yyparser.yylval = new ParserVal((Object)String.valueOf(c)); return Parser.OP; case '<': case '>': case '=': char next = NextChar(); if (next == '=') { yyparser.yylval = new ParserVal((Object)(c + "=")); } else { UngetChar(); yyparser.yylval = new ParserVal((Object)String.valueOf(c)); } return Parser.RELOP; case '{': yyparser.yylval = new ParserVal((Object)"{"); return Parser.BEGIN; case '}': yyparser.yylval = new ParserVal((Object)"}"); return Parser.END; } // If we get here, we found an invalid character return Fail(); } } }
Here is my code please draw a transition diagram and nfa on paper public class Lexer { private static final char EOF = 0; private static final int BUFFER_SIZE = 10; private Parser yyparser; // parent parser object private java.io.Reader reader; // input stream public int lineno; // line number public int column; // column // Double buffering implementation private char[] buffer1; private char[] buffer2; private boolean usingBuffer1; private int currentPos; private int bufferLength; private boolean endReached; // Keywords private static final String[] keywords = { "int", "print", "if", "else", "while", "void" }; public Lexer(java.io.Reader reader, Parser yyparser) throws Exception { this.reader = reader; this.yyparser = yyparser; this.lineno = 1; this.column = 0; // Initialize double buffering buffer1 = new char[BUFFER_SIZE]; buffer2 = new char[BUFFER_SIZE]; usingBuffer1 = true; currentPos = 0; bufferLength = 0; endReached = false; // Initial buffer fill fillBuffer(); } private void fillBuffer() throws Exception { char[] currentBuffer = usingBuffer1 ? buffer1 : buffer2; bufferLength = 0; while (bufferLength < BUFFER_SIZE) { int data = reader.read(); if (data == -1) { endReached = true; break; } currentBuffer[bufferLength++] = (char)data; } currentPos = 0; } public char NextChar() throws Exception { if (currentPos >= bufferLength) { if (endReached) { return EOF; } usingBuffer1 = !usingBuffer1; fillBuffer(); if (bufferLength == 0) { return EOF; } } char c = usingBuffer1 ? buffer1[currentPos] : buffer2[currentPos]; currentPos++; column++; if (c == '\n') { lineno++; column = 0; } return c; } public void UngetChar() { if (currentPos > 0) { currentPos--; column--; if (column < 0) { lineno--; // We don't know the previous line's length, so we'll set it to 0 column = 0; } } } public int Fail() { return -1; } private boolean isDigit(char c) { return c >= '0' && c <= '9'; } private boolean isLetter(char c) { return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z'); } private boolean isWhitespace(char c) { return c == ' ' || c == '\t' || c == '\n' || c == '\r'; } private String readIdentifier(char firstChar) throws Exception { StringBuilder sb = new StringBuilder(); sb.append(firstChar); while (true) { char c = NextChar(); if (isLetter(c) || isDigit(c) || c == '_') { sb.append(c); } else { UngetChar(); break; } } return sb.toString(); } private String readNumber(char firstDigit) throws Exception { StringBuilder sb = new StringBuilder(); sb.append(firstDigit); boolean hasDecimal = false; while (true) { char c = NextChar(); if (isDigit(c)) { sb.append(c); } else if (c == '.' && !hasDecimal) { hasDecimal = true; sb.append(c); } else { UngetChar(); break; } } return sb.toString(); } // * If yylex reach to the end of file, return 0 // * If there is a lexical error found, return -1 // * If a proper lexeme is determined, return token public int yylex() throws Exception { while (true) { char c = NextChar(); // Handle EOF if (c == EOF) { return 0; } // Skip whitespace if (isWhitespace(c)) { continue; } // Handle identifiers and keywords if (isLetter(c)) { String lexeme = readIdentifier(c); // Check if it's a keyword for (String keyword : keywords) { if (lexeme.equals(keyword)) { yyparser.yylval = new ParserVal((Object)lexeme); switch (keyword) { case "int": return Parser.INT; case "print": return Parser.PRINT; case "if": return Parser.IF; case "else": return Parser.ELSE; case "while": return Parser.WHILE; case "void": return Parser.VOID; } } } // Not a keyword, so it's an identifier yyparser.yylval = new ParserVal((Object)lexeme); return Parser.ID; } // Handle numbers if (isDigit(c)) { String number = readNumber(c); yyparser.yylval = new ParserVal((Object)number); return Parser.NUM; } // Handle operators and symbols switch (c) { case '(': yyparser.yylval = new ParserVal((Object)"("); return Parser.LPAREN; case ')': yyparser.yylval = new ParserVal((Object)")"); return Parser.RPAREN; case ';': yyparser.yylval = new ParserVal((Object)";"); return Parser.SEMI; case ',': yyparser.yylval = new ParserVal((Object)","); return Parser.COMMA; case '+': case '-': case '*': case '/': yyparser.yylval = new ParserVal((Object)String.valueOf(c)); return Parser.OP; case '<': case '>': case '=': char next = NextChar(); if (next == '=') { yyparser.yylval = new ParserVal((Object)(c + "=")); } else { UngetChar(); yyparser.yylval = new ParserVal((Object)String.valueOf(c)); } return Parser.RELOP; case '{': yyparser.yylval = new ParserVal((Object)"{"); return Parser.BEGIN; case '}': yyparser.yylval = new ParserVal((Object)"}"); return Parser.END; } // If we get here, we found an invalid character return Fail(); } } }
New Perspectives on HTML5, CSS3, and JavaScript
6th Edition
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Patrick M. Carey
Chapter14: Exploring Object-based Programming: Designing An Online Poker
Section14.1: Visual Overview: Custom Objects, Properties, And Methods
Problem 7QC
Related questions
Question
Here is my code please draw a transition diagram and nfa on paper
public class Lexer
{
private static final char EOF = 0;
private static final int BUFFER_SIZE = 10;
private Parser yyparser; // parent parser object
private java.io.Reader reader; // input stream
public int lineno; // line number
public int column; // column
// Double buffering implementation
private char[] buffer1;
private char[] buffer2;
private boolean usingBuffer1;
private int currentPos;
private int bufferLength;
private boolean endReached;
// Keywords
private static final String[] keywords = {
"int", "print", "if", "else", "while", "void"
};
public Lexer(java.io.Reader reader, Parser yyparser) throws Exception
{
this.reader = reader;
this.yyparser = yyparser;
this.lineno = 1;
this.column = 0;
// Initialize double buffering
buffer1 = new char[BUFFER_SIZE];
buffer2 = new char[BUFFER_SIZE];
usingBuffer1 = true;
currentPos = 0;
bufferLength = 0;
endReached = false;
// Initial buffer fill
fillBuffer();
}
private void fillBuffer() throws Exception {
char[] currentBuffer = usingBuffer1 ? buffer1 : buffer2;
bufferLength = 0;
while (bufferLength < BUFFER_SIZE) {
int data = reader.read();
if (data == -1) {
endReached = true;
break;
}
currentBuffer[bufferLength++] = (char)data;
}
currentPos = 0;
}
public char NextChar() throws Exception
{
if (currentPos >= bufferLength) {
if (endReached) {
return EOF;
}
usingBuffer1 = !usingBuffer1;
fillBuffer();
if (bufferLength == 0) {
return EOF;
}
}
char c = usingBuffer1 ? buffer1[currentPos] : buffer2[currentPos];
currentPos++;
column++;
if (c == '\n') {
lineno++;
column = 0;
}
return c;
}
public void UngetChar() {
if (currentPos > 0) {
currentPos--;
column--;
if (column < 0) {
lineno--;
// We don't know the previous line's length, so we'll set it to 0
column = 0;
}
}
}
public int Fail()
{
return -1;
}
private boolean isDigit(char c) {
return c >= '0' && c <= '9';
}
private boolean isLetter(char c) {
return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z');
}
private boolean isWhitespace(char c) {
return c == ' ' || c == '\t' || c == '\n' || c == '\r';
}
private String readIdentifier(char firstChar) throws Exception {
StringBuilder sb = new StringBuilder();
sb.append(firstChar);
while (true) {
char c = NextChar();
if (isLetter(c) || isDigit(c) || c == '_') {
sb.append(c);
} else {
UngetChar();
break;
}
}
return sb.toString();
}
private String readNumber(char firstDigit) throws Exception {
StringBuilder sb = new StringBuilder();
sb.append(firstDigit);
boolean hasDecimal = false;
while (true) {
char c = NextChar();
if (isDigit(c)) {
sb.append(c);
} else if (c == '.' && !hasDecimal) {
hasDecimal = true;
sb.append(c);
} else {
UngetChar();
break;
}
}
return sb.toString();
}
// * If yylex reach to the end of file, return 0
// * If there is a lexical error found, return -1
// * If a proper lexeme is determined, return token
public int yylex() throws Exception
{
while (true) {
char c = NextChar();
// Handle EOF
if (c == EOF) {
return 0;
}
// Skip whitespace
if (isWhitespace(c)) {
continue;
}
// Handle identifiers and keywords
if (isLetter(c)) {
String lexeme = readIdentifier(c);
// Check if it's a keyword
for (String keyword : keywords) {
if (lexeme.equals(keyword)) {
yyparser.yylval = new ParserVal((Object)lexeme);
switch (keyword) {
case "int": return Parser.INT;
case "print": return Parser.PRINT;
case "if": return Parser.IF;
case "else": return Parser.ELSE;
case "while": return Parser.WHILE;
case "void": return Parser.VOID;
}
}
}
// Not a keyword, so it's an identifier
yyparser.yylval = new ParserVal((Object)lexeme);
return Parser.ID;
}
// Handle numbers
if (isDigit(c)) {
String number = readNumber(c);
yyparser.yylval = new ParserVal((Object)number);
return Parser.NUM;
}
// Handle operators and symbols
switch (c) {
case '(':
yyparser.yylval = new ParserVal((Object)"(");
return Parser.LPAREN;
case ')':
yyparser.yylval = new ParserVal((Object)")");
return Parser.RPAREN;
case ';':
yyparser.yylval = new ParserVal((Object)";");
return Parser.SEMI;
case ',':
yyparser.yylval = new ParserVal((Object)",");
return Parser.COMMA;
case '+':
case '-':
case '*':
case '/':
yyparser.yylval = new ParserVal((Object)String.valueOf(c));
return Parser.OP;
case '<':
case '>':
case '=':
char next = NextChar();
if (next == '=') {
yyparser.yylval = new ParserVal((Object)(c + "="));
} else {
UngetChar();
yyparser.yylval = new ParserVal((Object)String.valueOf(c));
}
return Parser.RELOP;
case '{':
yyparser.yylval = new ParserVal((Object)"{");
return Parser.BEGIN;
case '}':
yyparser.yylval = new ParserVal((Object)"}");
return Parser.END;
}
// If we get here, we found an invalid character
return Fail();
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
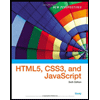
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
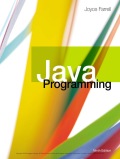
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
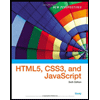
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
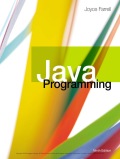
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
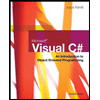
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
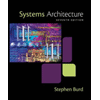
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
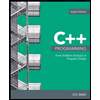
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning