C++ help. In the class definition, initialize the data members, string type and integer age, with the default values "Unstated" and 0, respectively. Ex: If the input is mouse 13, then the output is: Type: Unstated, Age: 0 Type: mouse, Age: 13 Note: The class's print function is called first after the default constructor, then again after the inputs are passed to the setters.''' #include <iostream>#include <string>using namespace std; class Animal { public: void SetType(string animalType); void SetAge(int animalAge); void Print(); private: /* Your code goes here */ }; void Animal::SetType(string animalType) { type = animalType;} void Animal::SetAge(int animalAge) { age = animalAge;} void Animal::Print() { cout << "Type: " << type << ", Age: " << age << endl;} int main() { string newType; int newAge; Animal myAnimal; myAnimal.Print(); cin >> newType; cin >> newAge; myAnimal.SetType(newType); myAnimal.SetAge(newAge); myAnimal.Print(); return 0;}'''
C++ help.
In the class definition, initialize the data members, string type and integer age, with the default values "Unstated" and 0, respectively.
Ex: If the input is mouse 13, then the output is:
Type: Unstated, Age: 0 Type: mouse, Age: 13
Note: The class's print function is called first after the default constructor, then again after the inputs are passed to the setters.
'''
#include <iostream>
#include <string>
using namespace std;
class Animal {
public:
void SetType(string animalType);
void SetAge(int animalAge);
void Print();
private:
/* Your code goes here */
};
void Animal::SetType(string animalType) {
type = animalType;
}
void Animal::SetAge(int animalAge) {
age = animalAge;
}
void Animal::Print() {
cout << "Type: " << type << ", Age: " << age << endl;
}
int main() {
string newType;
int newAge;
Animal myAnimal;
myAnimal.Print();
cin >> newType;
cin >> newAge;
myAnimal.SetType(newType);
myAnimal.SetAge(newAge);
myAnimal.Print();
return 0;
}
'''
Unlock instant AI solutions
Tap the button
to generate a solution
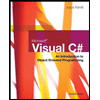
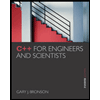
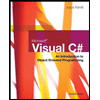
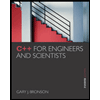
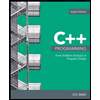
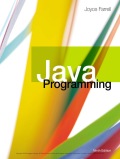
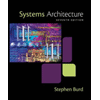