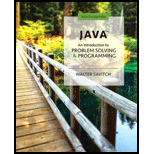
Concept explainers
Explanation of Solution
Changing statements inside “if” block:
The output of the
Changed program of Listing 9.2:
The modified statement is highlighted below.
//Import required package
import java.util.Scanner;
//Define a class
public class Main
{
//Define the main method
public static void main(String[] args)
{
Scanner keyboard = new Scanner(System.in);
//Try block
try
{
//Get the number of donuts
System.out.println("Enter number of donuts:");
int donutCount = keyboard.nextInt();
//Get the number of glasses of milk
System.out.println("Enter number of glasses of milk:");
int milkCount = keyboard.nextInt();
//Check if milkCount is less than 1
if(milkCount < 1)
{
//Create an exception
Exception e = new Exception("Exception: No milk!");
//Throw the exception
throw e;
}
//Calculate donuts per glass
double donutsPerGlass = donutCount / (double)milkCount;
//Print the donuts count
System...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- JAVA EXCEPTION HANDLING:Define a new exception, called ExceptionLineTooLong, that prints out the error message "The strings is too long". Write a program that reads phrase and throws an exception of type ExceptionLineTooLong in the case where a string is longer than 80 characters. For example: Input Result The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped…arrow_forwardwrite a code in javaarrow_forwardWrite a Month class that holds information about the month. Write exception classes for the following error conditions:• A number less than 1 or greater than 12 is given for the month number.• An invalid string is given for the name of the month.Modify the Month class so that it throws the appropriate exception when either of these errors occurs. Demonstrate the classes in a program.arrow_forward
- Subject: Object Oriented PrgrammingLanguage: Java ProgramTopic: Exception Define a new exception, called ExceptionLineTooLong, that prints out the error message "The strings is too long". Write a program that reads phrase and throws an exception of type ExceptionLineTooLong in the case where a string is longer than 80 characters. EXAMPLE: Input:The quick brown fox jumped over the lazy dogs. Output:The quick brown fox jumped over the laze dogs. ANOTHER EXAMPLE: Input:The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick…arrow_forwardwrite a code in javaarrow_forwardIn C++ You have a class called Fraction, which uses the heap. The constructor for the class is declared as: Fraction::Fraction(); Implement an exception handling design in the parameterized constructor to catch zeros in the denominator. Show the new exception class you will need to put in the header (specification) file. Show the definition for your parameterized constructor that throws the exception if the denominator is zero. Finally, show how to use the try-catch statement in the driver program when instatiating a new paramerized Fraction object. You do not need to show entire programs, only the requested lines of source codearrow_forward
- How can the standard error message be shown when an exception is thrown?arrow_forwardJAVA PROGRAM Probloem: Define a new exception, called ExceptionLineTooLong, that prints out the error message "The strings is too long". Write a program that reads phrase and throws an exception of type ExceptionLineTooLong in the case where a string is longer than 80 characters. First Example:Input:The quick brown fox jumped over the lazy dogs.Output:The quick brown fox jumped over the lazy dogs. Second Example:Input: The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown…arrow_forwardHow can the default error message be shown whenever an exception is thrown?arrow_forward
- Question 3 } public class WeekDay { static void daysFromSunday(int day) { String dayName = ""; try { } } if (day > 7) { throw new Exception("Invalid Day! Too high!"); } else if(day < 1){ throw new Exception("Invalid Day! Too low!"); } switch(day){ case 1: dayName="Sun"; break; case 2: dayName="Mon"; break; case 3: dayName="Tue"; break; case 4: dayName="Wed"; break; } ▬▬ } case 5: dayName="Thu"; Dreak; case 5: dayName="Thu"; break; case 6: dayName="Fri"; break; default: dayName="Sat"; System.out.println(dayName); } catch (Exception excpt) { System.out.println("Oops"); System.out.println(excpt.getMessage()); }finally{ System.out.println("Done!"); public static void main(String[] args) { daysFromSunday(10); daysFromSunday(3); daysFromSunday(0); OOops Invalid Day! Too high! Tue Oops Invalid Day! Too low! OOops Invalid Day! Too high! Done! Tue Done! Oops Invalid Day! Too low! Done! O Oops Invalid Day! Too high! Done! Oops Invalid Day! Too high! Done! Oops Invalid Day! Too low! Done! O Oops…arrow_forwardwrite a propram has a exception class named “Exception” that must inheritfrom the C++ runtime_errorclass.This class will capture the error case for a variable price when it is negative.For example, if the user tries to create with a variable price -1, it will generate an exception and this class should capture the reason and the error value, then print:Error: Invalid price. Ignored.arrow_forwarda) Write a method in java that searches a numeric array for a specified value. The method should return the subscript of the element containing the value if it is found in the array. If the value is not found , the method should throw an exceptionof the Exception class with the error message “Element not found”. b) Write an exception class that can be thrown when a negative number is passed to a method.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
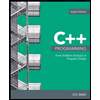