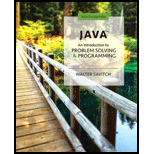
Suppose that you are in change of customer service for a certain business. As phone calls come in, the name of the caller is recorded and eventually a service representative returns the call and handles the request. Write a class ServiceRequests that keeps track of the names of callers. The class should have the following methods:
- addName (name)—adds a name to the list of names. Throws a ServiceBackUpException if there is no free space in the list
- removeName (name)—removes a name from the list Throws a NoServiceRequestException if the name is not in the list.
- getName (i)—returns the ith name in the 1st.
- getNumber—returns the current number of service requests.
Write a program that uses an object of type ServiceRequests to keep track of customers that have called. It should have a loop that, in each iteration, attempts to add a name, remove a name, or print all names. Use an array of size 10 as the list of names.
Graphics

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Database Concepts (8th Edition)
Modern Database Management
SURVEY OF OPERATING SYSTEMS
Concepts Of Programming Languages
Electric Circuits. (11th Edition)
- Can you please solve this. Thanksarrow_forwardcan you solve this pleasearrow_forwardIn the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forward
- In the previous homework scenario problem below: You have been hired by TechCo to create and manage their employee training portal. Your first task is to develop a program that will create and track different training sessions in the portal. Each training session has the following properties: • A session ID (e.g., "TECH101", "TECH205") • A session title (e.g., "Machine learning", "Advanced Java Programming") • A total duration in hours (e.g., 5.0, 8.0) • Current number of participants (e.g., 25) Each session must have at least a session ID and a total duration and must met the following requirements: • The maximum participant for each session is 30. • The total duration of a session must not exceed 10 hours. • The current number of participants should never exceed the maximum number of participants. Design an object-oriented solution to create a data definition class(DDC) and an implementation class for the session object. In the DDC, a session class must include: • Constructors to…arrow_forwardSend me the lexer and parserarrow_forwardHere is my code please draw a transition diagram and nfa on paper public class Lexer { private static final char EOF = 0; private static final int BUFFER_SIZE = 10; private Parser yyparser; // parent parser object private java.io.Reader reader; // input stream public int lineno; // line number public int column; // column // Double buffering implementation private char[] buffer1; private char[] buffer2; private boolean usingBuffer1; private int currentPos; private int bufferLength; private boolean endReached; // Keywords private static final String[] keywords = { "int", "print", "if", "else", "while", "void" }; public Lexer(java.io.Reader reader, Parser yyparser) throws Exception { this.reader = reader; this.yyparser = yyparser; this.lineno = 1; this.column = 0; // Initialize double buffering buffer1 = new char[BUFFER_SIZE]; buffer2 = new char[BUFFER_SIZE]; usingBuffer1 = true; currentPos = 0; bufferLength = 0; endReached = false; // Initial buffer fill fillBuffer(); } private…arrow_forward
- Create 2 charts using this data. One without using wind speed and one including max speed in mph. Write a Report and a short report explaining your visualizations and design decisions. Include the following: Lead Story: Identify the key story or insight based on your visualizations. Shaffer’s 4C Framework: Describe how you applied Shaffer’s 4C principles in the design of your charts. External Data Integration: Explain the second data and how you integrated it with the Halloween dataset. Compare the two datasets. Attach screenshots of the two charts (Bar graph or Line graph) The Shaffer 4 C’s of Data Visualization Clear - easily seen; sharply defined• who's the audience? what's the message? clarity more important than aestheticsClean - thorough; complete; unadulterated, labels, axis, gridlines, formatting, right chart type, colorchoice, etc.Concise - brief but comprehensive. not minimalist but not verboseCaptivating - to attract and hold by beauty or excellence does it capture…arrow_forwardHow can I resolve the following issue?arrow_forwardI need help to resolve, thank you.arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
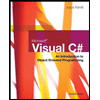
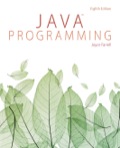
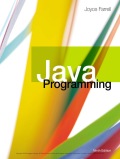
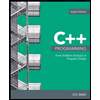
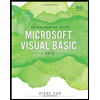