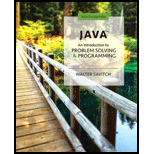
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 9.3, Problem 24STQ
Explanation of Solution
“throws” clause:
- • This clause is used to declare the exception. This provides information to the programmer that the
program might produce an exception so it is better to give the exception handling code which maintains the normal flow of the program. - • It is usually given in the method header.
Syntax:
Method_Name throws ExceptionName
{
//statements
}
Adding “throws” clause to the given method:
//throws clause is added to the Method header
public void ...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 9.1 - Prob. 1STQCh. 9.1 - What output would the code in the previous...Ch. 9.1 - Prob. 3STQCh. 9.1 - Prob. 4STQCh. 9.1 - Prob. 5STQCh. 9.1 - Prob. 6STQCh. 9.1 - Prob. 7STQCh. 9.1 - Prob. 8STQCh. 9.1 - In the code given in Self-Test Question 1,...Ch. 9.1 - In the code given in Self-Test Question 1,...
Ch. 9.1 - Prob. 11STQCh. 9.1 - Prob. 12STQCh. 9.1 - Prob. 13STQCh. 9.1 - Prob. 14STQCh. 9.2 - Prob. 15STQCh. 9.2 - Prob. 16STQCh. 9.2 - Prob. 17STQCh. 9.2 - Prob. 18STQCh. 9.2 - Prob. 19STQCh. 9.2 - Prob. 20STQCh. 9.2 - Suppose that, in Self-Test Question 19, we change...Ch. 9.2 - Prob. 22STQCh. 9.2 - Prob. 23STQCh. 9.3 - Prob. 24STQCh. 9.3 - Prob. 25STQCh. 9.3 - Prob. 26STQCh. 9.3 - Prob. 27STQCh. 9.3 - Prob. 28STQCh. 9.3 - Repeat Self-Test Question 27, but change the value...Ch. 9.3 - Prob. 30STQCh. 9.3 - Prob. 31STQCh. 9.3 - Prob. 32STQCh. 9.3 - Consider the following program: a. What output...Ch. 9.3 - Write an accessor method called getPrecision that...Ch. 9.3 - Prob. 35STQCh. 9.4 - Prob. 36STQCh. 9.4 - Prob. 37STQCh. 9.4 - Prob. 38STQCh. 9 - Write a program that allows students to schedule...Ch. 9 - Prob. 2ECh. 9 - Prob. 3ECh. 9 - Prob. 4ECh. 9 - Prob. 5ECh. 9 - Write code that reads a string from the keyboard...Ch. 9 - Create a class Rational that represents a rational...Ch. 9 - Prob. 9ECh. 9 - Suppose that you are going to create an object...Ch. 9 - Revise the class RoomCounter described in the...Ch. 9 - Prob. 12ECh. 9 - Write a class LapTimer that can be used to time...Ch. 9 - Prob. 1PCh. 9 - Prob. 2PCh. 9 - Prob. 3PCh. 9 - Write a program that uses the class calculator in...Ch. 9 - Prob. 3PPCh. 9 - Prob. 7PPCh. 9 - Suppose that you are in change of customer service...Ch. 9 - Write an application that implements a trip-time...
Knowledge Booster
Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
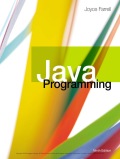
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
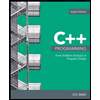
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
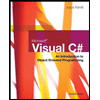
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
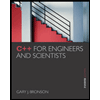
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
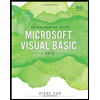
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning