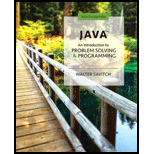
Explanation of Solution
Creating “Main.java”:
- Create a class named “Main”.
- Define the “main ()” method.
- Declare required variables.
- Do till the user enters “n” using “while” condition.
- Get the string from the user.
- Check if the length of the string is greater than or equal to 20.
- Create an object “e” for the class. Here the exception is thrown using default constructor.
- Get and print the message using the method “e.getMessage()”.
- Else,
- Print the number of characters.
- Ask whether the user wants to continue or not and store the response in a variable “response”.
- Check if the response is equal to “n”.
- Break the loop.
- Define the “main ()” method.
Creating “MessageTooLongException.java”:
- Create a class named “MessageTooLongException” that extends “Exception”.
- Define a default constructor that calls the parent class’s method using “super ()” by passing a message.
- Define a parameterized constructor that calls the parent class’s method using “super ()” by passing a message that is given as the argument.
Program:
MessageTooLongException.java:
//Define a class
public class MessageTooLongException extends Exception
{
//Default constructor
public MessageTooLongException()
{
//Call the parent class by passing the message
super("Message Too Long!");
}
//Parameterized constructor
public MessageTooLongException(String message)
{
//Call the parent class by passing the message
super(message);
}
}
Main.java:
//import the package
import java.util.Scanner;
//Main class
class Main
{
//Define main method
public static void main(String[] args)
{
//Create an object for the scanner class
Scanner sc = new Scanner(System.in);
//Declare required variables
String str, response = "y";
//Do till the user enters 'n'
while(response...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.arrow_forward2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forward
- hello please explain the architecture in the diagram below. thanks youarrow_forwardComplete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forward
- Hello please look at the attached picture. I need an detailed explanation of the architecturearrow_forwardInformation Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
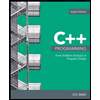
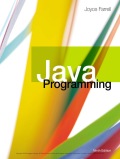
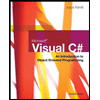
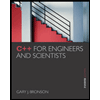
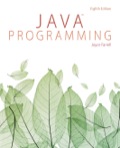