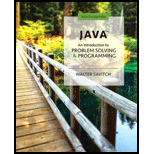
Concept explainers
Explanation of Solution
Modified code:
“Account.java”:
//Class Account
public class Account
{
//Private variable
private double balance;
//Define a constructor
public Account()
{
//Initialize the amount value
balance = 0;
}
//Parameterized constructor
public Account(double initialDeposit)
{
//Assign the value
balance = initialDeposit;
}
//Function to get balance
public double getBalance()
{
//Return the amount
return balance;
}
//Function to deposit the amount
public double deposit(double amount) throws NegativeAmountException
{
//Check if amount is greater than 0
if (amount > 0)
//Add the value to the balance
balance += amount;
//Else
Else
//return -1;
//Throw an exception
throw new NegativeAmountException();
//Return the balance
return balance;
}
//Function definition to withdraw the amount
public double withdraw(double amount) throws InsufficentBalanceException, NegativeAmountException
{
//Check if amount is greater than balance
if (amount > balance)
//throw an exception
throw new InsufficentBalanceException();
//Check if amount is less than 0
else if (amount < 0)
//Throw an exception
throw new NegativeAmountException();
//Else
else
//Calculate the balance
balance -= amount;
//Return the balance amount
return balance;
}
//Main method
public static void main(String[] args)
{
//Create an object for the class
Account a = new Account(10);
//Try block
try
{
//Deposit the value
a...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
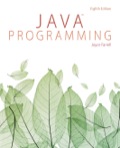
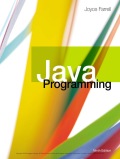
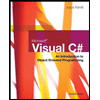
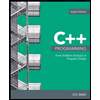
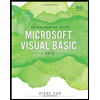