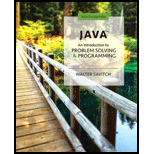
Write a class LapTimer that can be used to time the laps in a race. The class should have the following private attributes:
- running — a boolean indication of whether the timer is running
- startTime — the time when the timer started
- lapStart — the timer’s value when the current lap started
- lapTime — the elapsed time for the last lap
- totalTime — the total time from the start of the race through the last completed lap
- lapsCompleted — the number of laps completed so far
- lapsInRace — the number of laps in the race
The class should have the following methods:
- LapTimer (n) — a constructor for a race having n laps.
- start — starts the timer. Throws an exception if the race has already started.
- markLap — marks the end of the current lap and the start of a new lap. Throws an exception if the race is finished.
- getLapTime — returns the time of the last lap. Throws an exception if the first lap has not yet been completed.
- getTotalTime — returns the total time from the start of the race through the last completed lap. Throws an exception if the first lap has not yet been completed.
- getLapsRemaining — returns the number of laps yet to be completed, including the current one.
Express all times in seconds.
To get the current time in milliseconds from some baseline date, invoke
Calendar.getInstance ().getTimeInMillis ()
This invocation returns a primitive value of type long. By taking the difference between the returned values of two invocations at two different times, you will know the elapsed time in milliseconds between the invocations. Note that the class Calendar is in the package java.util.

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
SURVEY OF OPERATING SYSTEMS
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Modern Database Management
Electric Circuits. (11th Edition)
- Every attribute and method declared in a class has an access modifier such as private, public and protected. Differentiate each of them.arrow_forwardThe monkey class should look similar to the dog class.arrow_forwardUsing JAVASCRIPT write an object prototype for a Person that has a name and age, has a printInfo method, and also has a method that increments the persons age by 1 each time the method is called. Create two people using the 'new' keyword, and print both of their infos and increment one persons age by 3 years. Use an arrow function for both methods */ // Create our Person Prototype // Use an arrow to create the printInfo method // Create another arrow function for the addAge method that takes a single parameter // Adding to the agearrow_forward
- The Point2D should store an x and y coordinate pair, and will be used to build a new class via class composition. A Point2D has a x and a y, while a LineSegment has a start point and an end point (both of which are represented as Point2Ds). class Invariants The start and end points of a line segment should never be null Initialize these to the origin instead. Data A LineSegment has a start point This is a Point2D object All data will be private A LineSegment also has an end point. Also a Point2D object Methods Create getters and setters for your start and end points public Point2D getStartPoint() { public void setStartPoint(Point2D start) { Create a toString() function to build a string composed of the startPoint’s toString() and endPoint’s toString() Should look like “Line start(0,0) and end(1,1)” Create an equals method that determines if two LineSegments are equal public boolean equals(Object other) { if(other == null || !(other instanceof LineSegment)) return…arrow_forwardCode the StudentAccount class according to the class diagramMethods explanation:pay(double): doubleThis method is called when a student pays an amount towards outstanding fees. The balance isreduced by the amount received. The updated balance is returned by the method.addFees(double): doubleThis method is called to increase the balance by the amount received as a parameter. The updatedbalance is returned by the method.refund(): voidThis method is called when any monies owned to the student is paid out. This method can onlyrefund a student if the balance is negative. It displays the amount to be refunded and set thebalance to zero. (Use Math.abs(double) in your output message). If there is no refund, display anappropriate message.Test your StudentAccount class by creating objects and calling the methods addFees(), pay() andrefund(). Use toString() to display the object’s data after each method calledarrow_forwardObject adapters and class adapters each provide a unique function. These concepts are also significant due to the significance that you attach to them.arrow_forward
- Library Information System Design and Testing Library Item Class Design and TestingDesign a class that holds the Library Item Information, with item name, author, publisher. Write appropriate accessor and mutator methods. Also, write a tester program(Console and GUI Program) that creates three instances/objects of the Library Items class. Extending Library Item Class Library and Book Classes: Extend the Library Item class in (1) with a Book class with data attributes for a book’s title, author, publisher and an additional attributes as number of pages, and a Boolean data attribute indicating whether there is both hard copy as well as eBook version of the book. Demonstrate Book Class in a Tester Program (Console and GUI Program) with an object of Book class.arrow_forwardA group or collection of objects with common properties is called as: Casting Method Attributes Classarrow_forwardjava programming language You are part of a team writing classes for the different game objects in a video game. You need to write classes for the two human objects warrior and politician. A warrior has the attributes name (of type String) and speed (of type int). Speed is a measure of how fast the warrior can run and fight. A politician has the attributes name (of type String) and diplomacy (of type int). Diplomacy is the ability to outwit an adversary without using force. From this description identify a superclass as well as two subclasses. Each of these three classes need to have a default constructor, a constructor with parameters for all the instance variables in that class (as well as any instance variables inherited from a superclass) accessor (get) and mutator (set) methods for all instance variables and a toString method. The toString method needs to return a string representation of the object. Also write a main method for each class in which that class is tested – create…arrow_forward
- The attributes field of objects is a place where data may be saved. The class is solely responsible for its own properties.arrow_forwardImplement the following class Employee. This class is composed by 4 attributes and 3 methods. 1) Create a class Employee containing: _init_( ) method to initialize the different attributes . Get EmployeeName method Get EmployeeSSN method . Get EmployeeDepartment method 2) Define the method main to create 4 employees and apply the different methods Employee # Name: # Social Security Number: # Department # Salary + + Get Employee Name() Get Employee Social Security Number() + Get Employee Department() 3arrow_forwardjava languageCreate a class with following attributes: The car's make The car's model The car's year Now let's identify the class's methods. Specifically, the actions are: Constructor of Car class Set and get the car's make Set and get the car's model Set and get the car's year Now create few instance of the Car in a Test class. Use getter and setter to set the instance variables. Create a class with following attributes: The car's make The car's model The car's year Now let's identify the class's methods. Specifically, the actions are: Constructor of Car class Set and get the car's make Set and get the car's model Set and get the car's year Now create few instance of the Car in a Test class. Use getter and setter to set the instance variables.arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
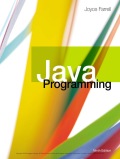
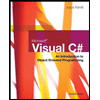