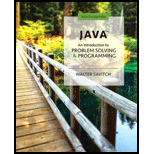
Explanation of Solution
Creating a class “RationalAssert.java”:
- Import required package.
- Define the class “RationalAssert”.
- Declare the private variables “the_numerator”, and “the_denominator”.
- Define parameterized constructor.
- Assign “numerator” to “the_numerator”.
- Assign “denominator” to “the_denominator”.
- Create an assertion with the condition “assert the_denominator != 0”.
- Give mutator methods to set numerator and denominator.
- Give accessor methods to get the value of numerator and denominator.
- Give function definition for “value ()”.
- Return the value produced by “the_numerator / the_denominator”.
- Given function “toString ()”.
- Return the string “the_numerator / the_denominator”.
- Define the “main ()” method.
- Create an object for “Scanner” class.
- Declare the variables “n”, and “d”.
- Get the numerator and denominator from the user.
- Call the constructor by passing “n”, and “d”.
- Call the function “value”.
- Call the function to get the numerator and denominator.
- Print new line.
Program:
Rational.java:
import java.util.Scanner;
//Define a class
public class RationalAssert
{
//Declare required variables
private int the_numerator;
private int the_denominator;
//Parameterized constructor
public RationalAssert(int numerator, int denominator)
{
//Assign the value of numerator
the_numerator = numerator;
//Assign the value of denominator
the_denominator = denominator;
//Declare an assertion
assert the_denominator != 0;
}
//Function to set the value of numerator
public void setNumerator(int numerator)
{
//Set the value
the_numerator = numerator;
}
//Function to set the denominator
public void setDenominator(int denominator)
{
//Declare an assertion
assert the_denominator != 0;
//Assign the value
the_denominator = denominator;
}
�...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- language is c++ sample output included with user input in boldarrow_forward82. Write a class definition for a class named Fraction that contains two integer fields named numerator and denominator. Include in the class a prototype for a method (function) named add that takes two Fraction objects as input arguments and returns a Fraction object as output. You do not need to provide an implementation of the add method.arrow_forwardc++arrow_forward
- Construct a java program to create a class Person that contains ID number and name. ID and name are initialized by a constructor. Create a new class Employee derived from Person. Employee class takes one additional entry salary and initializes all three variables through a constructor by reusing the definition of parent class constructor. Use an Exception handling mechanism to validate the values of the variables like ID will accept only integers, name accepts only string and salary accepts only double values. If users try to give some other inputs to these variables your program must show an exception. Define a new class Test which creates the objects of the classes for invoking the respective constructors.arrow_forwardSuggest a simple scheme for creating a new class SafeMember that would allow us to export a reference to a Member. The classes outside the system should be unaware of this additional class, and access the reference like a reference to a Member object. However, the reference would not allow the integrity of the data to be compromised. Remember that when designing the “Return Book with fines” use case, Member object is returned to the UserInterface to be able to display the member information such as owed fine. But this also means that the UserInterface can access all the Member methods. Your implementation of SafeMember class should prevent this.arrow_forwardProblem C • -3: method consonants() had more than one loop • -3: method initials () had more than one loop • -3: at least one method used nested loopsarrow_forward
- problem statement: Write a java classStringCheckto do the below given checks on accepted string or strings. Each function should be coded in separate methods of the class. Give appropriate instance variables to implement the method. The user should be able to enter the string through the main function in Main class. Use a constructor to initialize the String entered by the user. Use the Main class to test all the methods in StringCheckclass. The programs should have the below given checklist: 1)Define classStringCheck with required instance variables 2)The StringCheck class should have six methods to do the below functions: *Check if the user entered string is a simple sentence? (Starts with capital letter, Ends with period, space between words). *Check if the user entered string is a question? (Check if the string starts with ac apital letter ends with a question mark, space between words) *Check if the user entered string have numbers in it? *Check if string is a valid email *Check…arrow_forwardUse the definition of the class productType to answer the questions a,b,c,d, and e pictured below. *class productType is also pictured below *Everything is in C++arrow_forwardWrite c# equivalent statements for the following: There is a book library. Define classes respectively for a library. The library must contain a name and a list of books (use array). The books only contain author name as information. In the class, which describes the library, create methods to add a book to the library, to search for a book by a predefined author, and to delete a book from the library. Write a test class, which creates an object of type library, adds several books to it. Implement a test functionality, which finds all books authored by Stephen King and deletes them. Finally, display information for each of the remaining books.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
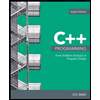