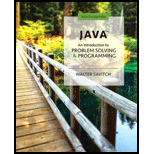
Explanation of Solution
Given program:
DoubleException.java:
//Define a class
public class DoubleException extends Exception
{
//Default constructor
public DoubleException()
{
//Call the method from parent class
super("Double exception thrown!");
}
//Parameterized constructor
public DoubleException(String message)
{
//Call the parent class's method
super(message + " " + message);
}
}
Main.java:
//Define the main class
class Main
{
//Define the main method
public static void main(String[] args)
{
//Try block
try
{
//Print the statement
System.out.println("try block entered:");
//Declare a variable
int number = 42;
//Check if the value is greater than 0
if(number > 0)
...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Suppose the exception class CrazyException is defined as follows: public class CrazyException extends Exception { public CrazyException() { super("Crazy exception thrown!"); System.out.println("Wow, Crazy exception thrown!"); } public CrazyException(String message) { super(message); System.out.println("Wow, crazy exception thrown with "+ "an argument!"); } public void crazyMethod() { System.out.println("Message is " + getMessage()); } } What output would be produced by the following unlikely code? CrazyException exceptionObject = new CrazyException(); System.out.println(exceptionObject.getMessage()); exceptionObject.crazyMethod();arrow_forwardJAVA EXCEPTION HANDLING:Define a new exception, called ExceptionLineTooLong, that prints out the error message "The strings is too long". Write a program that reads phrase and throws an exception of type ExceptionLineTooLong in the case where a string is longer than 80 characters. For example: Input Result The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped…arrow_forwardvoid foo () { try { throw new Exception1 (); print (" A "); throw new Exception2 (); print (" B "); } catch ( Exception1 e1 ) { print " handler1 "; } print (" C "); throw new Exception2 (); } void main () { try { try { foo (); print (" D "); } catch ( Exception1 e1 ) { print " handler2 "; } print (" E "); } catch ( Exception2 e2 ) { print " handler3 "; } } Write down the output of the program and justify why it is the output. Instead of the “replacement” semantics of exception handling used in modern languages, a very early design of exception handling introduced in the PL/I language uses a “binding” semantics. In particular, the design dynamically tracks a sequence of “catch” blocks that are currently active; a catch block is active whenever the corresponding try block is active. What will be the output of the following program if the language uses the “binding” semantics? Give the reasoning for your answer.arrow_forward
- What exception does this fragment of code raise? class Exception_Demo{ public static void main(String [] args) { try { int a = 10, b = 0; int c = a/b; System.out.println ("Result" + c); } } } a) NumberFormat b) NullPointer c) ArrayIndexOutOfBounds d) Arithmeticarrow_forwardJAVAarrow_forwardJAVA programming languagearrow_forward
- JAVA EXCEPTION HANDLING Complete the java code below according to the instructions given. Also use the test example below: Define the exceptions that are necessary to catch the possible errors that can occur in the class Matrix • ExceptionWrongMatrixValues that is thrown in the method read() if the data on the String does not correspond to numeric values, or if the data are not consistent with the form of a matrix (e.g., the rows have different length); • ExceptionWrongMatrixDimension that is thrown in the method read() if the data on the String do not correspond to the dimension of the matrix. The numbers are separated by space. A dot in a string means the next string of text is for the next row. For example: Test Result String input="1 2 3 . 1 e 3 ."; Matrix m = new Matrix(); m.read(input); ExceptionWrongMatrixValues String input="1 2 3 . 1 2 3 4 5 ."; Matrix m = new Matrix(); m.read(input); ExceptionWrongMatrixValues String input="12312345"; Matrix m = new Matrix();…arrow_forwardJAVA PROGRAM Probloem: Define a new exception, called ExceptionLineTooLong, that prints out the error message "The strings is too long". Write a program that reads phrase and throws an exception of type ExceptionLineTooLong in the case where a string is longer than 80 characters. First Example:Input:The quick brown fox jumped over the lazy dogs.Output:The quick brown fox jumped over the lazy dogs. Second Example:Input: The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown…arrow_forwardJava Foundations: Please provide a basic exception program. Do not use input, output. 1 Write the Java code for the following: Write the InvalidGradeExcepti on class that will be used below Write the Java program that reads an integer grade from a user and checks that it is valid (between 0 and 100) If it is invalid an InvalidGradeExcepti on will be thrown, stating what the invalid grade is. This will be caught in the main() method which will write a message about the invalid grade. If the grade is valid, it is ritten to the monitor.arrow_forward
- Subject: Object Oriented PrgrammingLanguage: Java ProgramTopic: Exception Define a new exception, called ExceptionLineTooLong, that prints out the error message "The strings is too long". Write a program that reads phrase and throws an exception of type ExceptionLineTooLong in the case where a string is longer than 80 characters. EXAMPLE: Input:The quick brown fox jumped over the lazy dogs. Output:The quick brown fox jumped over the laze dogs. ANOTHER EXAMPLE: Input:The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick brown fox jumped over the lazy dogs.The quick brown fox jumped over the lazy dogs. The quick…arrow_forwardDefined Exception Handling Exception Handling is used to overcome exceptions that take place during programs’ execution in a systematic manner. For instance, dividing a number by ‘0’ is a mathematical error (not defined). As you learned in class, you can use exception handling to overcome such operations. If you write a code without using exception handling, then the output of division by zero will be shown as infinity which cannot be further processed. Consider the following code: float Division(float num, float den) {return (num / den);} int main() { float numerator = 12.5; float denominator = 0; float result; result = Division(numerator, denominator); cout << "The quotient of 12.5/0 is " << result << endl; }arrow_forwardFind the error in each of the following code segmentsarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
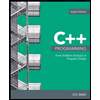