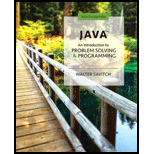
Concept explainers
Explanation of Solution
Creating “TimeOfDay.java”:
- Import required packages.
- Define the “TimeOfDay” class.
- Declare required variables.
- Define the constructor and instantiate the variables.
- Give function definition “setTimeTo ()” to set the time.
- Declare required variables.
- Create an object for the “Scanner” class.
- Split the string using a delimiter “:”.
- Inside the “try” block,
- Get the hour and store it in a variable.
- Catch the exception “Exception”.
- Throw the exception “InvalidFormattingException” with a message.
- Check if the hour value is valid.
- Throw “InvalidHourException” with a message.
- Get the remaining string and cut off the last two characters.
- Check if the string’s length is less than 3.
- Throw “InvalidFormattingException” with a message.
- Get the minutes and store it in a variable.
- Get “am” or “pm” and store it in a variable.
- Inside “try” block,
- Convert the minute string to integer.
- Catch the exception “Exception”.
- Throw “InvalidFormattingException” with a message.
- Check if minute is less than 0 or greater than 59.
- Throw “InvalidMinuteException” with a message.
- Check whether the string is not equal to “am” and “pm”.
- Throw “InvalidFormattingException” with a message.
- Check if the string is equal to “am”.
- Assign “true” to “isAM”.
- Else,
- Assign “false” to “isAM”.
- Function to produce the resultant string.
- Append all the value to a string.
- Define the “main ()” method.
- Create an object for the “Scanner” class.
- Create an object for the class “TimeOfDay”.
- Declare a variable “time”.
- Inside “try” block.
- Get the time from the user.
- Call the function “setTimeTo ()” by passing the time.
- Catch the exception “InvalidFormattingException”.
- Print the message using “getMessage ()” function.
- Catch the exception “InvalidHourException”.
- Print the message using “getMessage ()” function.
- Catch the exception “InvalidMinuteException”.
- Print the message using “getMessage ()” function.
- Print the time.
Creating “InvalidFormattingException.java”:
- Define a class “InvalidFormattingException” that extends “Exception”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Creating “InvalidHourException.java”:
- Define a class “InvalidHourException” that extends “InvalidFormattingException”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Creating “InvlaidMinuteException.java”:
- Define a class “InvalidMinuteException” that extends “InvalidFormattingException”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Program:
TimeOfDay.java:
//Import required packages
import java.util.*;
import java.util.Scanner;
//Define the main class
class TimeOfDay
{
//Declare required variables
private int hour, minute;
private boolean isAM;
//Constructor
public TimeOfDay()
{
//Instantiate the values
hour = 0;
minute = 0;
isAM = false;
}
//Function definition to set the time
public void setTimeTo(String aTime) throws InvalidFormattingException, InvalidHourException, InvalidMinuteException
{
//Declare required variables
int hourFound;
int minuteFound;
String indicatorFound;
//Create an object for the scanner class
Scanner reader = new Scanner(aTime);
//Split using the delimiter
reader.useDelimiter(":");
//Try block
try
{
//Assign the hour
hourFound = reader.nextInt();
}
//Catch the exception
catch (Exception e)
{
//Throw the exception with a message
throw new InvalidFormattingException("Hour not an integer");
}
//Check the condition
if(hourFound<1 || hourFound>12)
//Throw the exception with a message
throw new InvalidHourException("Hour not in the range of 1 to 12");
//Get the remaining string
String restOfString = reader.next();
reader = new Scanner(restOfString);
//Remove the last two characters
if(restOfString.length()<3)
//Throw the exception
throw new InvalidFormattingException("Bad format");
//Get the substring
String minuteString = restOfString.substring(0, restOfString.length()-2);
//Get the substring
String amString = restOfString.substring(restOfString.length()-2);
//Try block
try
{
//Convert the minute to integer
minuteFound = Integer.parseInt(minuteString);
}
//Catch the exception
catch (Exception e)
{
//Throw the exception
throw new InvalidFormattingException("Minute not an integer");
}
//Check the condition
if(minuteFound<0 || minuteFound>59)
//Throw the exception with a message
throw new InvalidMinuteException("Minute not in the range of 0 to 59");
//Check condition
if(!amString...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Please don't take this post down. It is not a homework assignment and follows all guidelines. Code in Java and post the finished result. Write a RetailItem class that holds data pertaining to a retail item. Write an exception class that can be instantiated and thrown when a negative number is given for the price. Write another exception class that can be instantiated and thrown when a negative number is given for the units on hand. Demonstrate the exception classes in a program. Please use pseudocode to easily explain each line of code and also include a simple algorithm and write in your pseudocode where it is. Also include a simple UML diagram.. RetailItem.Java public class RetailItem{ private String description; // Item description private int unitsOnHand; // Number of units on hand private double price; // Unit price /** This constructor initializes the item's description with an empty string, units on hand to 0, and price to 0.0. */…arrow_forwardDefine an exception class called CoreBreachException. The class should have a default constructor. If an exception is thrown using zero-argument constructor, getMessage should return "Cor Breach Evacuation Ship!" The class should also define a constructor having a single parameter of type String. If an exception is thrown using constructor, getMessage should return the value that was used as an argument to constructor.arrow_forwardDefine an exception class called tornadoException. The class should have two constructors including the default constructor. If the exception is thrown with the default constructor, the method what should return "Tornado: Take cover immediately!". The other constructor has a single parameter, say m, of the int type. If the exception is thrown with this constructor, the method what should return "Tornado: m miles away; and approaching!"arrow_forward
- Write a program that calls a method that throws an exception of type ArithmeticExcepton at arandom iteration in a for loop. Catch the exception in the method and pass the iteration countwhen the exception occurred to the calling method by using an object of an exception class youdefinein java codearrow_forwardWrite an exception class that is appropriate for indicating that a time enteredby a user is not valid. The time will be in the format hour:minute followed by“am” or “pm.”arrow_forwardin c # i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. Next, create a Book class that contains fields for title, author, price, and number of pages. Include properties for each field. Throw a BookException if a client program tries to construct a Book object for which the price is more than 10 cents per page. Finally, using the Book class, create an array of five Books. Prompt the user for values for each Book. To handle any exceptions that are thrown because of improper or invalid data entered by the user, set the Book’s price to the maximum of 10 cents per page. At the end of the program, display all the entered, and…arrow_forward
- in C# i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. For example, an error message: For Goodnight Moon, ratio is invalid. ...Price is $12.99 for 25 pages. Next, create a Book class that contains fields for title, author, price, and number of pages. Include properties for each field. Throw a BookException if a client program tries to construct a Book object for which the price is more than 10 cents per page. Finally, using the Book class, create an array of five Books. Prompt the user for values for each Book. To handle any exceptions that are thrown because of improper or invalid data entered by the user, set the Book’s…arrow_forwardjavaarrow_forwardThis is a java program question dont copy from any other source or internet for gods sake write the program and give me the output also write the code in your own imagination dont copy it from other source pleasearrow_forward
- in c # i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. my errors are Test Case IncompleteComplete program walkthrough, test 1 InputBook1Author110.99200Book2Author219.99100Book3Author225.00600Book4Author35.0020Book5Author48.00120OutputEnter information for book #1:Title: Author: Price: Number of pages: Unhandled Exception:System.DivideByZeroException: Value was either too large or too small for a Decimal. at System.Decimal+DecCalc.VarDecDiv (System.Decimal& d1, System.Decimal& d2) [0x0003e] in <7b90a8780ac4414295b539b19eea7eea>:0 at System.Decimal.Divide (System.Decimal d1, System.Decimal d2) [0x00000] in…arrow_forwardin C# i need to Write the program BookExceptionDemo for the Peterman Publishing Company. Create a BookException class that is instantiated when a Book’s price exceeds 10 cents per page and whose constructor requires three arguments for title, price, and number of pages. Create an error message that is passed to the Exception class constructor for the Message property when a Book does not meet the price-to-pages ratio. my errors are Test Case IncompleteComplete program walkthrough, test 1 InputBook1Author110.99200Book2Author219.99100Book3Author225.00600Book4Author35.0020Book5Author48.00120OutputBook1 by Author1 Price $10.99 200 pages.Book2 by Author2 Price $19.99 100 pages.Book3 by Author2 Price $25.00 600 pages.Book4 by Author3 Price $5.00 20 pages.Book5 by Author4 Price $8.00 120 pages. ResultsFor Book2, ratio is invalid....Price is $19.99 for 100 pages.For Book4, ratio is invalid....Price is $5.00 for 20 pages.Book1 by Author1 Price $10.99 200 pages.Book2 by Author2 Price $10.00 100…arrow_forwardWrite a program that shows a constructor passing information about constructor failure to an exception handler. Define class SomeException, which throws an Exception in the constructor. Your program should try to create an object of type SomeException and catch the exception that is thrown from the constructor.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
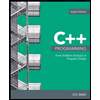
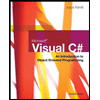