2. UNIX Shell and History Feature [20 points] This question consists of designing a C program to serve as a shell interface that accepts user commands and then executes each command in a separate process. A shell interface gives the user a prompt, after which the next command is entered. The example below illustrates the prompt osh> and the user's next command: cat prog.c. The UNIX/Linux cat command displays the contents of the file prog.s on the terminal using the UNIX/Linux cat command and your program needs to do the same. osh> cat prog.c www The above can be achieved by running your shell interface as a parent process. Every time a command is entered, you create a child process by using fork(), which then executes the user's command using one of the system calls in the exec() family (as described in Chapter 3). A C program that provides the general operations of a command-line shell can be seen below. #include #include #define MAX LINE 80 /* The maximum length command */ { int main(void) char *args (MAX LINE/2 + 1]; /* command line arguments */ int should run = 1; /* flag to determine when to exit program */ while (should run) { printf("osh>"); fflush(stdout); /** *After reading user input, the steps are: * (1) fork a child process using fork() *(2) the child process will invoke execvp() * (3) parent will invoke wait() unless command included & } return 0; } Figure 3.32 Outline of simple shell. The main () function presents the prompt osh-> and outlines the steps to be taken after input from the user has been read. The main ( ) function continually loops as long as should run equals 1; when the user enters exit at the prompt, your program will set should run to 0 and terminate. This question is organized into two parts: First Part: [10 points] Creating the child process and executing the command in the child Your shell interface needs to handle the following two cases. 1) Parent waits while the child process executes. In this case, the parent process first reads what the user enters on the command line (in this case, cat prog.c), and then creates a separate child process that executes the command. Unless otherwise specified, the parent process waits for the child to exit before continuing. This is similar in functionality to the new process creation illustrated in Lab 3. 2) Parent executes in the background or concurrently while the child process executes (similar to UNIX/Linux) To distinguish this case from the first one, add an ampersand (&) at the end of the command. Thus, if we rewrite the above command as osh> cat progic & the parent and child processes will run concurrently. Second Part: [10 points] Modifying the shell to allow a history feature In this part your shell interface program should provide a history feature that allows the user to access the most recently entered commands. The user will be able to access up to 5 commands by using the feature. The commands will be consecutively numbered starting at 1, and the numbering will continue past 5. For example, if the user has entered 35 commands, the 5 most recent commands will be numbered 31 to 35. The user will be able to list the command history by entering the command osh history As an example, assume that the history consists of the commands (from most to least recent): 1s -1, top, ps, who, date. The command history should output: 5 ls -1 4 top 3 ps 2 who 1 date Your program should support two techniques for retrieving commands from the command history:
2. UNIX Shell and History Feature [20 points] This question consists of designing a C program to serve as a shell interface that accepts user commands and then executes each command in a separate process. A shell interface gives the user a prompt, after which the next command is entered. The example below illustrates the prompt osh> and the user's next command: cat prog.c. The UNIX/Linux cat command displays the contents of the file prog.s on the terminal using the UNIX/Linux cat command and your program needs to do the same. osh> cat prog.c www The above can be achieved by running your shell interface as a parent process. Every time a command is entered, you create a child process by using fork(), which then executes the user's command using one of the system calls in the exec() family (as described in Chapter 3). A C program that provides the general operations of a command-line shell can be seen below. #include #include #define MAX LINE 80 /* The maximum length command */ { int main(void) char *args (MAX LINE/2 + 1]; /* command line arguments */ int should run = 1; /* flag to determine when to exit program */ while (should run) { printf("osh>"); fflush(stdout); /** *After reading user input, the steps are: * (1) fork a child process using fork() *(2) the child process will invoke execvp() * (3) parent will invoke wait() unless command included & } return 0; } Figure 3.32 Outline of simple shell. The main () function presents the prompt osh-> and outlines the steps to be taken after input from the user has been read. The main ( ) function continually loops as long as should run equals 1; when the user enters exit at the prompt, your program will set should run to 0 and terminate. This question is organized into two parts: First Part: [10 points] Creating the child process and executing the command in the child Your shell interface needs to handle the following two cases. 1) Parent waits while the child process executes. In this case, the parent process first reads what the user enters on the command line (in this case, cat prog.c), and then creates a separate child process that executes the command. Unless otherwise specified, the parent process waits for the child to exit before continuing. This is similar in functionality to the new process creation illustrated in Lab 3. 2) Parent executes in the background or concurrently while the child process executes (similar to UNIX/Linux) To distinguish this case from the first one, add an ampersand (&) at the end of the command. Thus, if we rewrite the above command as osh> cat progic & the parent and child processes will run concurrently. Second Part: [10 points] Modifying the shell to allow a history feature In this part your shell interface program should provide a history feature that allows the user to access the most recently entered commands. The user will be able to access up to 5 commands by using the feature. The commands will be consecutively numbered starting at 1, and the numbering will continue past 5. For example, if the user has entered 35 commands, the 5 most recent commands will be numbered 31 to 35. The user will be able to list the command history by entering the command osh history As an example, assume that the history consists of the commands (from most to least recent): 1s -1, top, ps, who, date. The command history should output: 5 ls -1 4 top 3 ps 2 who 1 date Your program should support two techniques for retrieving commands from the command history:
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section8.4: File Streams As Function Arguments
Problem 1E
Related questions
Question
- Whentheuserenters!!,themostrecentcommandinthehistoryisexecuted.In the example above, if the user entered the command:
Osh> !!
The ‘ls -l’ command should be executed and echoed on user’s screen. The command should also be placed in the history buffer as the next command.
- Whentheuserentersasingle!followedbyanintegerN,theNthcommandin the history is executed. In the example above, if the user entered the command:
Osh> ! 3
The ‘ps’ command should be executed and echoed on the user’s screen. The command should also be placed in the history buffer as the next command.
Error handling:
The program should also manage basic error handling. For example, if there are no commands in the history, entering !! should result in a message “No commands in history.” Also, if there is no command corresponding to the number entered with the single !, the program should output "No such command in history."
![2. UNIX Shell and History Feature [20 points]
This question consists of designing a C program to serve as a shell interface that
accepts user commands and then executes each command in a separate process. A
shell interface gives the user a prompt, after which the next command is entered.
The example below illustrates the prompt osh> and the user's next command:
cat prog.c. The UNIX/Linux cat command displays the contents of the file
prog.s on the terminal using the UNIX/Linux cat command and your program
needs to do the same.
osh> cat prog.c
www
The above can be achieved by running your shell interface as a parent process.
Every time a command is entered, you create a child process by using fork(),
which then executes the user's command using one of the system calls in the
exec() family (as described in Chapter 3). A C program that provides the general
operations of a command-line shell can be seen below.
#include <stdio.h>
#include <unistd.h>
#define MAX LINE 80 /* The maximum length command */
{
int main(void)
char *args (MAX LINE/2 + 1]; /* command line arguments */
int should run = 1; /* flag to determine when to exit program */
while (should run) {
printf("osh>");
fflush(stdout);
/**
*After reading user input, the steps are:
* (1) fork a child process using fork()
*(2) the child process will invoke execvp()
* (3) parent will invoke wait() unless command included &
}
return 0;
}
Figure 3.32 Outline of simple shell.
The main () function presents the prompt osh-> and outlines the steps to be
taken after input from the user has been read. The main ( ) function continually
loops as long
as should run equals 1; when the user enters exit at the prompt, your program
will set should run to 0 and terminate.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F92e5c125-0162-44af-aecb-b4cf9027a9d3%2F923532eb-84e4-4da0-b8e4-20b6802255d4%2Fah3ip7ue_processed.png&w=3840&q=75)
Transcribed Image Text:2. UNIX Shell and History Feature [20 points]
This question consists of designing a C program to serve as a shell interface that
accepts user commands and then executes each command in a separate process. A
shell interface gives the user a prompt, after which the next command is entered.
The example below illustrates the prompt osh> and the user's next command:
cat prog.c. The UNIX/Linux cat command displays the contents of the file
prog.s on the terminal using the UNIX/Linux cat command and your program
needs to do the same.
osh> cat prog.c
www
The above can be achieved by running your shell interface as a parent process.
Every time a command is entered, you create a child process by using fork(),
which then executes the user's command using one of the system calls in the
exec() family (as described in Chapter 3). A C program that provides the general
operations of a command-line shell can be seen below.
#include <stdio.h>
#include <unistd.h>
#define MAX LINE 80 /* The maximum length command */
{
int main(void)
char *args (MAX LINE/2 + 1]; /* command line arguments */
int should run = 1; /* flag to determine when to exit program */
while (should run) {
printf("osh>");
fflush(stdout);
/**
*After reading user input, the steps are:
* (1) fork a child process using fork()
*(2) the child process will invoke execvp()
* (3) parent will invoke wait() unless command included &
}
return 0;
}
Figure 3.32 Outline of simple shell.
The main () function presents the prompt osh-> and outlines the steps to be
taken after input from the user has been read. The main ( ) function continually
loops as long
as should run equals 1; when the user enters exit at the prompt, your program
will set should run to 0 and terminate.
![This question is organized into two parts:
First Part: [10 points] Creating the child process and executing the command
in the child
Your shell interface needs to handle the following two cases.
1) Parent waits while the child process executes.
In this case, the parent process first reads what the user enters on the command line
(in this case, cat prog.c), and then creates a separate child process that
executes the command. Unless otherwise specified, the parent process waits for the
child to exit before continuing. This is similar in functionality to the new process
creation illustrated in Lab 3.
2) Parent executes in the background or concurrently while the child
process executes (similar to UNIX/Linux)
To distinguish this case from the first one, add an ampersand (&) at the end of the
command. Thus, if we rewrite the above command as osh> cat progic & the
parent and child processes will run concurrently.
Second Part: [10 points] Modifying the shell to allow a history feature
In this part your shell interface program should provide a history feature that allows
the user to access the most recently entered commands. The user will be able to
access up to 5 commands by using the feature. The commands will be consecutively
numbered starting at 1, and the numbering will continue past 5. For example, if the
user has entered 35 commands, the 5 most recent commands will be numbered 31
to 35. The user will be able to list the command history by entering the command
osh history
As an example, assume that the history consists of the commands (from most to
least recent): 1s -1, top, ps, who, date. The command history should
output:
5 ls -1
4 top
3 ps
2 who
1 date
Your program should support two techniques for retrieving commands from the
command history:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F92e5c125-0162-44af-aecb-b4cf9027a9d3%2F923532eb-84e4-4da0-b8e4-20b6802255d4%2Fn8ye4a_processed.png&w=3840&q=75)
Transcribed Image Text:This question is organized into two parts:
First Part: [10 points] Creating the child process and executing the command
in the child
Your shell interface needs to handle the following two cases.
1) Parent waits while the child process executes.
In this case, the parent process first reads what the user enters on the command line
(in this case, cat prog.c), and then creates a separate child process that
executes the command. Unless otherwise specified, the parent process waits for the
child to exit before continuing. This is similar in functionality to the new process
creation illustrated in Lab 3.
2) Parent executes in the background or concurrently while the child
process executes (similar to UNIX/Linux)
To distinguish this case from the first one, add an ampersand (&) at the end of the
command. Thus, if we rewrite the above command as osh> cat progic & the
parent and child processes will run concurrently.
Second Part: [10 points] Modifying the shell to allow a history feature
In this part your shell interface program should provide a history feature that allows
the user to access the most recently entered commands. The user will be able to
access up to 5 commands by using the feature. The commands will be consecutively
numbered starting at 1, and the numbering will continue past 5. For example, if the
user has entered 35 commands, the 5 most recent commands will be numbered 31
to 35. The user will be able to list the command history by entering the command
osh history
As an example, assume that the history consists of the commands (from most to
least recent): 1s -1, top, ps, who, date. The command history should
output:
5 ls -1
4 top
3 ps
2 who
1 date
Your program should support two techniques for retrieving commands from the
command history:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
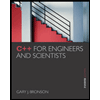
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
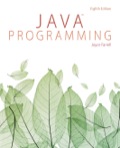
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
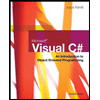
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
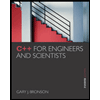
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
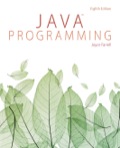
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
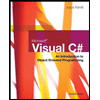
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
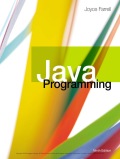
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
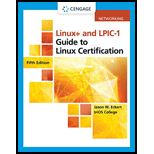
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:
9781337569798
Author:
ECKERT
Publisher:
CENGAGE L