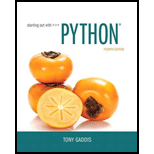
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 7, Problem 14PE
Expense Pie Chart
Create a text file that contains your expenses for last month in the following categories:
▪ Rent
▪ Gas
▪ Food
▪ Clothing
▪ Car payment
▪ Misc
Write a Python
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A cylinder of diameter 10 cm rotates concentrically inside another hollow
cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their
axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to
rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poise
Make the following game user friendly with GUI, with some simple graphics
The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units.
Cell Class:
public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…
Please original work
What are four of the goals of information lifecycle management think they are most important to data warehousing,
Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each.
Please cite in text references and add weblinks
Chapter 7 Solutions
Starting Out with Python (4th Edition)
Ch. 7.2 - What will the following code display? numbers =...Ch. 7.2 - Prob. 2CPCh. 7.2 - Prob. 3CPCh. 7.2 - Prob. 4CPCh. 7.2 - Prob. 5CPCh. 7.2 - Prob. 6CPCh. 7.2 - Prob. 7CPCh. 7.2 - Prob. 8CPCh. 7.3 - Prob. 9CPCh. 7.3 - Prob. 10CP
Ch. 7.3 - Prob. 11CPCh. 7.3 - Prob. 12CPCh. 7.3 - Prob. 13CPCh. 7.4 - What will the following code display? names =...Ch. 7.5 - Prob. 15CPCh. 7.5 - Prob. 16CPCh. 7.5 - Prob. 17CPCh. 7.5 - Prob. 18CPCh. 7.8 - Prob. 19CPCh. 7.8 - Prob. 20CPCh. 7.8 - Write a set of nested loops that display the...Ch. 7.9 - Prob. 22CPCh. 7.9 - Prob. 23CPCh. 7.9 - Prob. 24CPCh. 7.9 - Prob. 25CPCh. 7.10 - Prob. 26CPCh. 7.10 - Prob. 27CPCh. 7.10 - Prob. 28CPCh. 7.10 - Prob. 29CPCh. 7.10 - Prob. 30CPCh. 7.10 - To create a bar chart with the bar function, what...Ch. 7.10 - Assume the following statement calls the bar...Ch. 7.10 - Prob. 33CPCh. 7 - This term refers to an individual item in a list....Ch. 7 - This is a number that identifies an item in a...Ch. 7 - Prob. 3MCCh. 7 - This is the last index in a list. a. 1 b. 99 c. 0...Ch. 7 - This will happen if you try to use an index that...Ch. 7 - This function returns the length of a list. a....Ch. 7 - When the operator's left operand is a list and...Ch. 7 - This list method adds an item to the end of an...Ch. 7 - This removes an item at a specific index in a...Ch. 7 - Prob. 10MCCh. 7 - If you call the index method to locate an item in...Ch. 7 - Prob. 12MCCh. 7 - This file object method returns a list containing...Ch. 7 - Which of the following statement creates a tuple?...Ch. 7 - Prob. 1TFCh. 7 - Prob. 2TFCh. 7 - Prob. 3TFCh. 7 - Prob. 4TFCh. 7 - A file object's writelines method automatically...Ch. 7 - You can use the + operator to concatenate two...Ch. 7 - Prob. 7TFCh. 7 - You can remove an element from a tuple by calling...Ch. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - What will the following code display? values = [2,...Ch. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - Prob. 4AWCh. 7 - Write a function that accepts a list as an...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Total Sales Design a program that asks the user to...Ch. 7 - Prob. 2PECh. 7 - Rainfall Statistics Design a program that lets the...Ch. 7 - Prob. 4PECh. 7 - Prob. 5PECh. 7 - Larger Than n In a program, write a function that...Ch. 7 - Drivers License Exam The local driver s license...Ch. 7 - Name Search If you have downloaded the source code...Ch. 7 - Prob. 9PECh. 7 - World Series Champions If you have downloaded the...Ch. 7 - Prob. 11PECh. 7 - Prob. 12PECh. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Expense Pie Chart Create a text file that contains...Ch. 7 - 1994 Weekly Gas Graph In the student sample...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Design a class to represent a credit card. Think about the attributes of a credit card; that is, what data is o...
Java: An Introduction to Problem Solving and Programming (8th Edition)
Explain how an SEM micrograph is made. Check the Internet or the library to find the answer.
Degarmo's Materials And Processes In Manufacturing
Define each of the following terms: supertype subtype specialization entity cluster completeness constraint enh...
Modern Database Management
True or False: You must have a return statement in a value-returning method.
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
If a subclass extends a superclass with an abstract method, what must you do in the subclass?
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
What populates the Smalltalk world?
Concepts Of Programming Languages
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forwardIn this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forward
- You are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forwardDistributed Systems: Consistency Models fer to page 45 for problems on data consistency. structions: Compare different consistency models (e.g., strong, eventual, causal) for distributed databases. Evaluate the trade-offs between availability and consistency in a given use case. Propose the most appropriate model for the scenario and explain your reasoning. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440AZF/view?usp=sharing]arrow_forwardOperating Systems: Deadlock Detection fer to page 25 for problems on deadlock concepts. structions: • Given a system resource allocation graph, determine if a deadlock exists. If a deadlock exists, identify the processes and resources involved. Suggest strategies to prevent or resolve the deadlock and explain their trade-offs. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forward
- Artificial Intelligence: Heuristic Evaluation fer to page 55 for problems on Al search algorithms. tructions: Given a search problem, propose and evaluate a heuristic function. Compare its performance to other heuristics based on search cost and solution quality. Justify why the chosen heuristic is admissible and/or consistent. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 75 for graph-related problems. Instructions: • Implement a greedy graph coloring algorithm for the given graph. • Demonstrate the steps to assign colors while minimizing the chromatic number. • Analyze the time complexity and limitations of the approach. Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 150 for problems on socket programming. Instructions: • Develop a client-server application using sockets to exchange messages. • Implement both TCP and UDP communication and highlight their differences. • Test the program under different network conditions and analyze results. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 80 for problems on white-box testing. Instructions: • Perform control flow testing for the given program, drawing the control flow graph (CFG). • Design test cases to achieve statement, branch, and path coverage. • Justify the adequacy of your test cases using the CFG. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
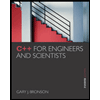
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
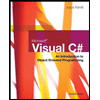
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
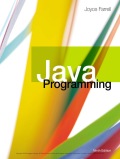
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
C - File I/O; Author: Tutorials Point (India) Ltd.;https://www.youtube.com/watch?v=cEfuwpbGi1k;License: Standard YouTube License, CC-BY
file handling functions in c | fprintf, fscanf, fread, fwrite |; Author: Education 4u;https://www.youtube.com/watch?v=aqeXS1bJihA;License: Standard Youtube License