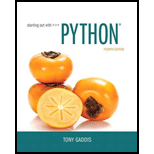
Concept explainers
List:
- • A list is an object that has sequence of elements.
- • The contents of the list can be changed at any point of time, so lists in Python are mutable.
- • Lists support various additional operations like “append()”, “insert()”, “remove()”, “del()”, etc.
- • Lists have dynamic size.
- • Lists store elements of same type, i.e. lists are homogenous.
- • List elements are enclosed by square brackets.
Syntax:
The syntax to create a list is as follows:
List_name = [element1, element2,…, elementn]
Explanation:
Here,
- • “List_name” specifies the list name.
- • “element1, element2, and elementn” indicate the list elements.
Example
Consider the below example that creates a list and prints the list.
#Declare a list with numbers
list_ex = [1, 2, 3, 4]
#Print the list
print(list_ex)
Sample Output:
[1, 2, 3, 4]
Program explanation:
In the above code,
- • “list_ex” represents a list having integers as its elements.
- • The list “list_ex” is printed using “print()” function.
Tuple:
- • A tuple is an object that has immutable sequence of elements.
- • The contents of the tuple cannot be altered once it is created, so tuples in Python are immutable.
- • Tuples have static size.
- • The elements of tuples can be of various types, i.e. tuples are heterogeneous.
- • Elements in the tuple are enclosed by parenthesis.
Syntax:
In python, a tuple is created using the below format:
#Create a tuple
Tuple_name = (element1, element2, …, elementn)
Explanation:
- • “Tuple_name” specifies the tuple name.
- • “element1, element2, and elementn” indicate the tuple elements.
Example program:
Consider the below example that creates a tuple and prints the tuple.
#Declare a tuple with numbers, strings
tuple_ex = [1, 'Joe', 'Tom', 4]
#Print the tuple
print(tuple_ex)
Sample Output:
[1, 'Joe', 'Tom', 4]
Program explanation:
In the above code,
- • “tuple_ex” represents a tuple having integers and strings as its elements.
- • Then, the tuple “tuple_ex” is printed using “print()” function.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with Python (4th Edition)
- Briefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forward
- I want to solve 13.2 using matlab please helparrow_forwarda) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
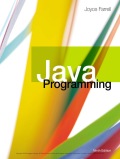
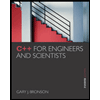
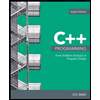
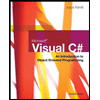
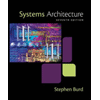
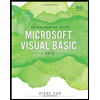