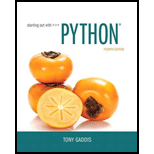
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7, Problem 5PE
Program Plan Intro
Charge account validation
Program Plan:
- • Declare a function named “readfile()”.
- ○ The function accepts a file handler “inputfileobject” as a parameter.
- ○ Create an empty list “chargeaccountlist” to hold the account number from the input file.
- ○ Use “for” to iterate through the file and fetch the charge account numbers from each line of the file.
- ○ “append()” function is used to add the fetched charge account numbers from the file to the list “chargeaccountlist”.
- ○ The function finally returns the list “chargeaccountlist”.
- • Declare a function named “checknumber()”.
- ○ The function accepts a list “chargeaccountlist” as a parameter.
- ○ Prompt the user to enter a seven digit charge account number and store in a variable “n”.
- ○ Use an “if” statement to check whether “n” is in the list “chargeaccountlist”. If the statement returns true, print the user entered charge account number is valid; otherwise, print invalid.
- • In the “main()” function,
- ○ Open the file “charge_accounts.txt” and assign to “inputfileobject”.
- ○ Call the function “readfile()” with “inputfileobject” as a parameter and assign the function return to the “chargeaccountslist”.
- ○ Call the function “checknumber()” with “chargeaccountslist” as a parameter.
- • Finally, call the “main()” to execute the program.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
solve this questions for me .
a) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?
Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as the
Chapter 7 Solutions
Starting Out with Python (4th Edition)
Ch. 7.2 - What will the following code display? numbers =...Ch. 7.2 - Prob. 2CPCh. 7.2 - Prob. 3CPCh. 7.2 - Prob. 4CPCh. 7.2 - Prob. 5CPCh. 7.2 - Prob. 6CPCh. 7.2 - Prob. 7CPCh. 7.2 - Prob. 8CPCh. 7.3 - Prob. 9CPCh. 7.3 - Prob. 10CP
Ch. 7.3 - Prob. 11CPCh. 7.3 - Prob. 12CPCh. 7.3 - Prob. 13CPCh. 7.4 - What will the following code display? names =...Ch. 7.5 - Prob. 15CPCh. 7.5 - Prob. 16CPCh. 7.5 - Prob. 17CPCh. 7.5 - Prob. 18CPCh. 7.8 - Prob. 19CPCh. 7.8 - Prob. 20CPCh. 7.8 - Write a set of nested loops that display the...Ch. 7.9 - Prob. 22CPCh. 7.9 - Prob. 23CPCh. 7.9 - Prob. 24CPCh. 7.9 - Prob. 25CPCh. 7.10 - Prob. 26CPCh. 7.10 - Prob. 27CPCh. 7.10 - Prob. 28CPCh. 7.10 - Prob. 29CPCh. 7.10 - Prob. 30CPCh. 7.10 - To create a bar chart with the bar function, what...Ch. 7.10 - Assume the following statement calls the bar...Ch. 7.10 - Prob. 33CPCh. 7 - This term refers to an individual item in a list....Ch. 7 - This is a number that identifies an item in a...Ch. 7 - Prob. 3MCCh. 7 - This is the last index in a list. a. 1 b. 99 c. 0...Ch. 7 - This will happen if you try to use an index that...Ch. 7 - This function returns the length of a list. a....Ch. 7 - When the operator's left operand is a list and...Ch. 7 - This list method adds an item to the end of an...Ch. 7 - This removes an item at a specific index in a...Ch. 7 - Prob. 10MCCh. 7 - If you call the index method to locate an item in...Ch. 7 - Prob. 12MCCh. 7 - This file object method returns a list containing...Ch. 7 - Which of the following statement creates a tuple?...Ch. 7 - Prob. 1TFCh. 7 - Prob. 2TFCh. 7 - Prob. 3TFCh. 7 - Prob. 4TFCh. 7 - A file object's writelines method automatically...Ch. 7 - You can use the + operator to concatenate two...Ch. 7 - Prob. 7TFCh. 7 - You can remove an element from a tuple by calling...Ch. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - What will the following code display? values = [2,...Ch. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - Prob. 4AWCh. 7 - Write a function that accepts a list as an...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Total Sales Design a program that asks the user to...Ch. 7 - Prob. 2PECh. 7 - Rainfall Statistics Design a program that lets the...Ch. 7 - Prob. 4PECh. 7 - Prob. 5PECh. 7 - Larger Than n In a program, write a function that...Ch. 7 - Drivers License Exam The local driver s license...Ch. 7 - Name Search If you have downloaded the source code...Ch. 7 - Prob. 9PECh. 7 - World Series Champions If you have downloaded the...Ch. 7 - Prob. 11PECh. 7 - Prob. 12PECh. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Expense Pie Chart Create a text file that contains...Ch. 7 - 1994 Weekly Gas Graph In the student sample...
Knowledge Booster
Similar questions
- whats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forward
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
- What are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
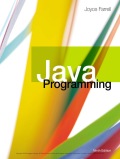
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
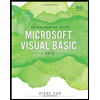
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
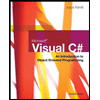
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
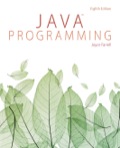
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
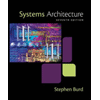
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning