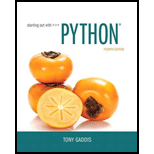
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7.2, Problem 2CP
Program Plan Intro
Python built-in functions:
- • In Python, “list()” is a built-in function that can change a type of object to a list.
- • In Python, “range()” is a built-in function used to produce a sequence of integers that can be iterated over. For instance, “range(4)” produces a list of 4 integers starting at index 0. The “range()” function follows 0-based indexing.
- • The statement “list(range(arguments))” modifies the “range()” function’s object to a list.
Syntax:
In Python, to create a list of integers, the built-in functions “list()” and “range()” are expressed as follows:
#Create a list of integers
list(range(arguments))
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please do not use any AI tools to solve this question.
I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor.
No AI-generated responses, please.
Obtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).
I cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ?
Cisco Packet Tracer
1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.
Chapter 7 Solutions
Starting Out with Python (4th Edition)
Ch. 7.2 - What will the following code display? numbers =...Ch. 7.2 - Prob. 2CPCh. 7.2 - Prob. 3CPCh. 7.2 - Prob. 4CPCh. 7.2 - Prob. 5CPCh. 7.2 - Prob. 6CPCh. 7.2 - Prob. 7CPCh. 7.2 - Prob. 8CPCh. 7.3 - Prob. 9CPCh. 7.3 - Prob. 10CP
Ch. 7.3 - Prob. 11CPCh. 7.3 - Prob. 12CPCh. 7.3 - Prob. 13CPCh. 7.4 - What will the following code display? names =...Ch. 7.5 - Prob. 15CPCh. 7.5 - Prob. 16CPCh. 7.5 - Prob. 17CPCh. 7.5 - Prob. 18CPCh. 7.8 - Prob. 19CPCh. 7.8 - Prob. 20CPCh. 7.8 - Write a set of nested loops that display the...Ch. 7.9 - Prob. 22CPCh. 7.9 - Prob. 23CPCh. 7.9 - Prob. 24CPCh. 7.9 - Prob. 25CPCh. 7.10 - Prob. 26CPCh. 7.10 - Prob. 27CPCh. 7.10 - Prob. 28CPCh. 7.10 - Prob. 29CPCh. 7.10 - Prob. 30CPCh. 7.10 - To create a bar chart with the bar function, what...Ch. 7.10 - Assume the following statement calls the bar...Ch. 7.10 - Prob. 33CPCh. 7 - This term refers to an individual item in a list....Ch. 7 - This is a number that identifies an item in a...Ch. 7 - Prob. 3MCCh. 7 - This is the last index in a list. a. 1 b. 99 c. 0...Ch. 7 - This will happen if you try to use an index that...Ch. 7 - This function returns the length of a list. a....Ch. 7 - When the operator's left operand is a list and...Ch. 7 - This list method adds an item to the end of an...Ch. 7 - This removes an item at a specific index in a...Ch. 7 - Prob. 10MCCh. 7 - If you call the index method to locate an item in...Ch. 7 - Prob. 12MCCh. 7 - This file object method returns a list containing...Ch. 7 - Which of the following statement creates a tuple?...Ch. 7 - Prob. 1TFCh. 7 - Prob. 2TFCh. 7 - Prob. 3TFCh. 7 - Prob. 4TFCh. 7 - A file object's writelines method automatically...Ch. 7 - You can use the + operator to concatenate two...Ch. 7 - Prob. 7TFCh. 7 - You can remove an element from a tuple by calling...Ch. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - What will the following code display? values = [2,...Ch. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - Prob. 4AWCh. 7 - Write a function that accepts a list as an...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Total Sales Design a program that asks the user to...Ch. 7 - Prob. 2PECh. 7 - Rainfall Statistics Design a program that lets the...Ch. 7 - Prob. 4PECh. 7 - Prob. 5PECh. 7 - Larger Than n In a program, write a function that...Ch. 7 - Drivers License Exam The local driver s license...Ch. 7 - Name Search If you have downloaded the source code...Ch. 7 - Prob. 9PECh. 7 - World Series Champions If you have downloaded the...Ch. 7 - Prob. 11PECh. 7 - Prob. 12PECh. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Expense Pie Chart Create a text file that contains...Ch. 7 - 1994 Weekly Gas Graph In the student sample...
Knowledge Booster
Similar questions
- using r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forwardusing r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forward
- using r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardA. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forward
- Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forwardGiven the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forward
- Considering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forwardGiven the FSA below, answer the following questions. b 1 3 a a b b с 2 A. Write a RegEx that is equivalent to this FSA as it is; B. Write a RegEx that is equivalent to this FSA removing the states and edges corresponding to the letter c. Note: To both items feel free to use any method you want, including analyzing which words are accepted by the FSA, to generate your RegEx.arrow_forward3) Finite State Automata Given the FSA below, answer the following questions. a b a b 0 1 2 b b 3 A. Give three 4-letter words that can be accepted by this FSA; B. Give three 4-letter words that cannot be accepted by this FSA; C. How could you describe the words accepted by this FSA? D. Is this FSA deterministic or non-deterministic?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
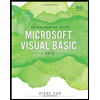
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
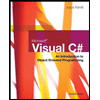
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
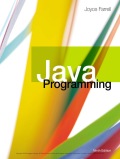
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
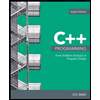
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage