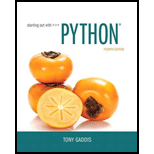
Concept explainers
List:
- • A list is an object that has sequence of elements.
- • The contents of the list can be changed at any point of time, so lists in Python are mutable.
- • Lists support various additional operations like “append()”, “insert()”, “remove()”, “del()”, etc.
- • Lists have a dynamic size.
- • Lists store elements of same type, i.e. lists are homogenous.
- • List elements are enclosed by square brackets.
Syntax:
The syntax to create a list is as follows:
List_name = [element1, element2,…, elementn]
Explanation:
Here,
- • “List_name” specifies the list name.
- • “element1, element2, and elementn” indicate the list elements.
Example
Consider the below example that creates a list and prints the list.
#Declare a list with numbers
list_ex = [1, 2, 3, 4]
#Print the list
print(list_ex)
Sample Output:
[1, 2, 3, 4]
Program explanation:
In the above code,
- • “list_ex” represents a list having integers as its elements.
- • Then, the list “list_ex” is printed using “print()” function.
Tuple:
- • Tuple is an object that has immutable sequence of elements.
- • The contents of the tuple cannot be altered once it is created. Therefore, tuples in Python are immutable.
- • Tuples have a static size.
- • The elements of tuples can be of various types, i.e. tuples are heterogeneous.
- • Elements in the tuple are enclosed by parenthesis.
Syntax:
In python, a tuple is created using the below format.
#Create a tuple
Tuple_name = (element1, element2, …, elementn)
Explanation:
- • “Tuple_name” specifies the tuple name.
- • “element1, element2, and elementn” indicate the tuple elements.
Example program:
Consider the below example that creates a tuple and prints the tuple.
#Declare a tuple with numbers, strings
tuple_ex = [1, 'Joe', 'Tom', 4]
#Print the tuple
print(tuple_ex)
Sample Output:
[1, 'Joe', 'Tom', 4]
Program explanation:
In the above code,
- • “tuple_ex” represents a tuple having integers and strings as its elements.
- • Then, the tuple “tuple_ex” is printed using “print()” function.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with Python (4th Edition)
- Need help with coding in this in python!arrow_forwardIn the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forward
- Why I need ?arrow_forwardHere are two diagrams. Make them very explicit, similar to Example Diagram 3 (the Architecture of MSCTNN). graph LR subgraph Teacher_Model_B [Teacher Model (Pretrained)] Input_Teacher_B[Input C (Complete Data)] --> Teacher_Encoder_B[Transformer Encoder T] Teacher_Encoder_B --> Teacher_Prediction_B[Teacher Prediction y_T] Teacher_Encoder_B --> Teacher_Features_B[Internal Features F_T] end subgraph Student_B_Model [Student Model B (Handles Missing Labels)] Input_Student_B[Input C (Complete Data)] --> Student_B_Encoder[Transformer Encoder E_B] Student_B_Encoder --> Student_B_Prediction[Student B Prediction y_B] end subgraph Knowledge_Distillation_B [Knowledge Distillation (Student B)] Teacher_Prediction_B -- Logits Distillation Loss (L_logits_B) --> Total_Loss_B Teacher_Features_B -- Feature Alignment Loss (L_feature_B) --> Total_Loss_B Partial_Labels_B[Partial Labels y_p] -- Prediction Loss (L_pred_B) --> Total_Loss_B Total_Loss_B -- Backpropagation -->…arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder --> Student_A_Features[Student A Features F_A] end subgraph Knowledge_Distillation_A [Knowledge Distillation (Student A)] Teacher_Prediction -- Logits Distillation Loss (L_logits_A) --> Total_Loss_A Teacher_Features -- Feature Alignment Loss (L_feature_A) --> Total_Loss_A Ground_Truth_A[Ground Truth y_gt] -- Prediction Loss (L_pred_A)…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
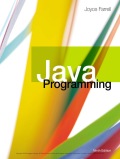
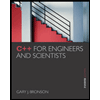
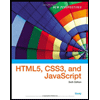
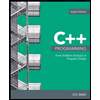
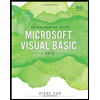
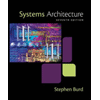