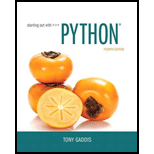
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7, Problem 4PE
Program Plan Intro
Program plan:
- • Declare a function named “NumberAnalysis()”.
- ○ Declare an empty list “ournumbers” to hold the input numbers.
- ○ Use a “for” loop to iterate up to the range of “20” and get “20” input numbers from the user and insert the numbers to the empty list “ournumbers” using “append()”.
- ○ Using the built-in functions “min()” to find the lowest number in the list “ournumbers” and display it using “print()” function.
- ○ Using the built-in functions “max()” to find the highest number in the list “ournumbers” and display it using “print()” function.
- ○ Using the built-in functions “sum()” to find the total of the numbers in the list “ournumbers” and display it using “print()” function.
- ○ To find the average of numbers in the list, the built-in function “sum()” is used to find the total of the numbers in the list “ournumbers” and sum of the numbers value is divided by the “len(ournumbers)”; result is displayed using “print()” function.
- • In the “main()” function:
- ○ Call the function “NumberAnalysis()”.
- • Finally, call the “main()” to execute the program.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
We are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7).
A code breaker manages to factories 247 = 13 x 19
Determine Alice's secret key.
To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.
Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.
Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnn
Chapter 7 Solutions
Starting Out with Python (4th Edition)
Ch. 7.2 - What will the following code display? numbers =...Ch. 7.2 - Prob. 2CPCh. 7.2 - Prob. 3CPCh. 7.2 - Prob. 4CPCh. 7.2 - Prob. 5CPCh. 7.2 - Prob. 6CPCh. 7.2 - Prob. 7CPCh. 7.2 - Prob. 8CPCh. 7.3 - Prob. 9CPCh. 7.3 - Prob. 10CP
Ch. 7.3 - Prob. 11CPCh. 7.3 - Prob. 12CPCh. 7.3 - Prob. 13CPCh. 7.4 - What will the following code display? names =...Ch. 7.5 - Prob. 15CPCh. 7.5 - Prob. 16CPCh. 7.5 - Prob. 17CPCh. 7.5 - Prob. 18CPCh. 7.8 - Prob. 19CPCh. 7.8 - Prob. 20CPCh. 7.8 - Write a set of nested loops that display the...Ch. 7.9 - Prob. 22CPCh. 7.9 - Prob. 23CPCh. 7.9 - Prob. 24CPCh. 7.9 - Prob. 25CPCh. 7.10 - Prob. 26CPCh. 7.10 - Prob. 27CPCh. 7.10 - Prob. 28CPCh. 7.10 - Prob. 29CPCh. 7.10 - Prob. 30CPCh. 7.10 - To create a bar chart with the bar function, what...Ch. 7.10 - Assume the following statement calls the bar...Ch. 7.10 - Prob. 33CPCh. 7 - This term refers to an individual item in a list....Ch. 7 - This is a number that identifies an item in a...Ch. 7 - Prob. 3MCCh. 7 - This is the last index in a list. a. 1 b. 99 c. 0...Ch. 7 - This will happen if you try to use an index that...Ch. 7 - This function returns the length of a list. a....Ch. 7 - When the operator's left operand is a list and...Ch. 7 - This list method adds an item to the end of an...Ch. 7 - This removes an item at a specific index in a...Ch. 7 - Prob. 10MCCh. 7 - If you call the index method to locate an item in...Ch. 7 - Prob. 12MCCh. 7 - This file object method returns a list containing...Ch. 7 - Which of the following statement creates a tuple?...Ch. 7 - Prob. 1TFCh. 7 - Prob. 2TFCh. 7 - Prob. 3TFCh. 7 - Prob. 4TFCh. 7 - A file object's writelines method automatically...Ch. 7 - You can use the + operator to concatenate two...Ch. 7 - Prob. 7TFCh. 7 - You can remove an element from a tuple by calling...Ch. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - What will the following code display? values = [2,...Ch. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - Prob. 4AWCh. 7 - Write a function that accepts a list as an...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Total Sales Design a program that asks the user to...Ch. 7 - Prob. 2PECh. 7 - Rainfall Statistics Design a program that lets the...Ch. 7 - Prob. 4PECh. 7 - Prob. 5PECh. 7 - Larger Than n In a program, write a function that...Ch. 7 - Drivers License Exam The local driver s license...Ch. 7 - Name Search If you have downloaded the source code...Ch. 7 - Prob. 9PECh. 7 - World Series Champions If you have downloaded the...Ch. 7 - Prob. 11PECh. 7 - Prob. 12PECh. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Expense Pie Chart Create a text file that contains...Ch. 7 - 1994 Weekly Gas Graph In the student sample...
Knowledge Booster
Similar questions
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
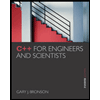
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
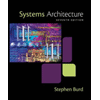
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
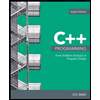
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning