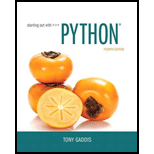
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 7.3, Problem 12CP
Program Plan Intro
List slicing:
- • Slicing is a concept of selecting a range of elements from a list.
- • In Python, an expression that selects a subsection of a list is known as a slice.
- • A slice is a span of elements that are taken from a list.
- • Normally, the slice expression has a start index and an end index for slicing.
- • In case if the start and end indices are not provided, the python employs 0 as the start index and length of the list as the end index.
Syntax:
In Python, the list slice statement without the start index and end index can be expressed as follows:
listname[:]
Explanation:
In the list slice syntax,
- • As the “start” index is not provided, python uses 0 as the start index by default.
- • As the “end” index is not provided, Python employs the length of the list as the “end” index for the slice.
- • The list slice expression returns a copy of the entire list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In the past, encryption and decryption were mostly done by substitution and permutation of letters in a text message. study those classic cryptographic schemes Then, develop an automatic cipher using Javascript The cipher should be able to perform the following tasks:
generate keys
encrypt a given plaintext message with a key selected from the list of keys generated
decrypt a given ciphertext message with a known cipher key
List reasons why teachers should and shouldn’t be replaced by computers? State your response in a descriptive context. Provide five references from the with internet with your answers.
The attached picture shows the RTCAPI memory mapped register which has the address 0x180.
1) Program the MCU's registers so that
a) SIRC is the clock source
b) the clock is divided by 16K
c) the clock is enabled
d) load is enabled
Chapter 7 Solutions
Starting Out with Python (4th Edition)
Ch. 7.2 - What will the following code display? numbers =...Ch. 7.2 - Prob. 2CPCh. 7.2 - Prob. 3CPCh. 7.2 - Prob. 4CPCh. 7.2 - Prob. 5CPCh. 7.2 - Prob. 6CPCh. 7.2 - Prob. 7CPCh. 7.2 - Prob. 8CPCh. 7.3 - Prob. 9CPCh. 7.3 - Prob. 10CP
Ch. 7.3 - Prob. 11CPCh. 7.3 - Prob. 12CPCh. 7.3 - Prob. 13CPCh. 7.4 - What will the following code display? names =...Ch. 7.5 - Prob. 15CPCh. 7.5 - Prob. 16CPCh. 7.5 - Prob. 17CPCh. 7.5 - Prob. 18CPCh. 7.8 - Prob. 19CPCh. 7.8 - Prob. 20CPCh. 7.8 - Write a set of nested loops that display the...Ch. 7.9 - Prob. 22CPCh. 7.9 - Prob. 23CPCh. 7.9 - Prob. 24CPCh. 7.9 - Prob. 25CPCh. 7.10 - Prob. 26CPCh. 7.10 - Prob. 27CPCh. 7.10 - Prob. 28CPCh. 7.10 - Prob. 29CPCh. 7.10 - Prob. 30CPCh. 7.10 - To create a bar chart with the bar function, what...Ch. 7.10 - Assume the following statement calls the bar...Ch. 7.10 - Prob. 33CPCh. 7 - This term refers to an individual item in a list....Ch. 7 - This is a number that identifies an item in a...Ch. 7 - Prob. 3MCCh. 7 - This is the last index in a list. a. 1 b. 99 c. 0...Ch. 7 - This will happen if you try to use an index that...Ch. 7 - This function returns the length of a list. a....Ch. 7 - When the operator's left operand is a list and...Ch. 7 - This list method adds an item to the end of an...Ch. 7 - This removes an item at a specific index in a...Ch. 7 - Prob. 10MCCh. 7 - If you call the index method to locate an item in...Ch. 7 - Prob. 12MCCh. 7 - This file object method returns a list containing...Ch. 7 - Which of the following statement creates a tuple?...Ch. 7 - Prob. 1TFCh. 7 - Prob. 2TFCh. 7 - Prob. 3TFCh. 7 - Prob. 4TFCh. 7 - A file object's writelines method automatically...Ch. 7 - You can use the + operator to concatenate two...Ch. 7 - Prob. 7TFCh. 7 - You can remove an element from a tuple by calling...Ch. 7 - Prob. 1SACh. 7 - Prob. 2SACh. 7 - What will the following code display? values = [2,...Ch. 7 - Prob. 4SACh. 7 - Prob. 5SACh. 7 - Prob. 6SACh. 7 - Prob. 1AWCh. 7 - Prob. 2AWCh. 7 - Prob. 3AWCh. 7 - Prob. 4AWCh. 7 - Write a function that accepts a list as an...Ch. 7 - Prob. 6AWCh. 7 - Prob. 7AWCh. 7 - Prob. 8AWCh. 7 - Total Sales Design a program that asks the user to...Ch. 7 - Prob. 2PECh. 7 - Rainfall Statistics Design a program that lets the...Ch. 7 - Prob. 4PECh. 7 - Prob. 5PECh. 7 - Larger Than n In a program, write a function that...Ch. 7 - Drivers License Exam The local driver s license...Ch. 7 - Name Search If you have downloaded the source code...Ch. 7 - Prob. 9PECh. 7 - World Series Champions If you have downloaded the...Ch. 7 - Prob. 11PECh. 7 - Prob. 12PECh. 7 - Magic 8 Ball Write a program that simulates a...Ch. 7 - Expense Pie Chart Create a text file that contains...Ch. 7 - 1994 Weekly Gas Graph In the student sample...
Knowledge Booster
Similar questions
- What is the ALU result if the 4-bit ALU Control signal is 0100? What happens if the ALU Control signal is 0101?arrow_forwardIn the past, encryption and decryption were mostly done by substitution and permutation of letters in a text message. study those classic cryptographic schemes Then, develop an automatic cipher using a programming language of your choice. The cipher should be able to perform the following tasks: generate keys encrypt a given plaintext message with a key selected from the list of keys generated decrypt a given ciphertext message with a known cipher keyarrow_forwardCase Study Instructions: Offshore Wind Energy in the North Sea For this case study, students will analyze the institutional challenges and social rules surrounding offshore wind energy development in the Dutch North Sea, as explored in the case study from the textbook (pages 44-46). Additional resources in this week’s learning module will provide further perspectives on the impact of wind energy on different stakeholders. Students should refer to the syllabus for specific guidelines regarding length, format, and content requirements. Reflection Questions to Consider: What are the key institutional challenges in implementing offshore wind energy in the North Sea? How do formal and informal social rules shape the use of this shared space? What conflicts arise between different stakeholders (e.g., fishermen, naval transport, military, and wind energy developers)? How can policymakers balance economic, environmental, and social considerations when allocating space for wind energy?…arrow_forward
- Alphabetic Telephone Number TranslatorMany companies use telephone numbers like 555-GET-FOOD so the number is easier for their customers to remember. On a standard telephone, the alphabetic letters are mapped to numbers in the following fashion: A, B, and C = 2 D, E, and F = 3 G, H, and I = 4 J, K, and L = 5 M, N, and O = 6 P, Q, R, and S = 7 T, U, and V = 8 W, X, Y, and Z = 9 Write a program that asks the user to enter a 10-character telephone number in the format XXX-XXX-XXXX. The application should display the telephone number with any alphabetic characters that appeared in the original translated to their numeric equivalent. For example, if the user enters 555-GET-FOOD, the application should display 555-438-3663.arrow_forwardI would like help to resolve the following casearrow_forward7. Character AnalysisIf you have downloaded the source code you will find a file named text.txt in the Chapter 08 folder. Write a program that reads the file’s contents and determines the following: The number of uppercase letters in the file The number of lowercase letters in the file The number of digits in the file The number of whitespace characters in the filearrow_forward
- Provide the full blue terminal commands & output and other things that are usefularrow_forwardHomework You have the row vector (A) that has values from (-100 to 100), write MATLAB codes to: (1) Interchange the first 10 elements with last ten elements. (2) Replace the elements at indices (93,9,17,50) of A with 99. (3) Return the second element and the element before the last of A as a column vector (C1). (4) Extract the first five elements and the last five elements of A and append them as a row vector (R). (5) Set the elements of A with odd indices to zeros(0).arrow_forwardif the error in a closed-loop Servo motor system is zero, why does the motor Contimac ranning instead of stoppingarrow_forward
- When the FCC added Color Television to the Industry Standards, they went with the system developed in the 1940s by Peter Goldman for CBS. Question 15 options: True False Part of the reason that many critics disliked 1950s gameshows was the fact that gameshows offered one of the few opportunities to see unscripted interactions with "real" (average/non-famous) people on television. Question 16 options: True False The Andy Griffith Show is an example of the "rural revival" shows that become enormously popular on 1960s American television. Question 19 options: True False During the Network Era, the hours before primetime each day were exclusively devoted to locally-produced programming, not programming dictated by an affiliate station's parent network. Question 20 options: True Falsearrow_forwardAlthough color television was not added to the industry standard until 1956, CBS had been broadcasting selected special events in color as early as 1950. Question 1 options: True False Two key factors in creating the Network Era of American television were the FCC licensing freeze and ______________. Question 4 options: The Quiz Show Scandals Habitual Viewing Operation Frontal Lobes Drop-In Viewing Least Objectionable Programming was designed to embrace the public service-oriented vision of using television to elevate mass culture and enrich viewers. Question 6 options: True False By the end of the 1950s, all three remaining networks (NBC, CBS, & ABC) were broadcasting their entire nightly programming schedule in full color. Question 9 options: True Falsearrow_forward7. See the code below and solve the following. public class Test { public static void main(String[] args) { int result = 0; } result = fn(2,3); System.out.println("The result is: + result); // fn(x, 1) = x // fn(x, y) = fn(x, y-1) + 2, when y>1 public static int fn(int x, int y) { if (x <= 1) return x; else return fn(x, y-1) + 2; } } 7-1. This program has a bug that leads to infinite recursion. Modify fn(int x, int y) method to fix the problem. (2 point) 7-2. Manually trace the recursive call, fn(2,3) and show the output (step by step). (2 point) 7-3. Can you identify the Base Case in recursive method fn(int x, int y)? (1 point)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
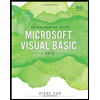
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
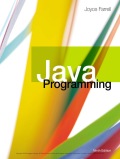
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
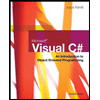
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
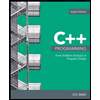
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage