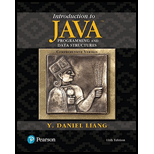
(Geometry: the bounding rectangle) A bounding rectangle is the minimum rectangle that encloses a set of points in a two-dimensional plane, as shown in Figure 10.24d. Write a method that returns a bounding rectangle for a set of points in a two-dimensional plane, as follows:
public static MyRectangle2D getRectangle(double[][] points)
The Rectang1e2D class is defined in

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (4th Edition)
Modern Database Management
Starting Out With Visual Basic (8th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- (Triangle class) Design a new Triangle class that extends the abstract GeometricObject class. Draw the UML diagram for the classes Triangle and GeometricObject and then implement the Triangle class. Write a test program that prompts the user to enter three sides of the triangle, a colour, and a Boolean value to indicate whether the triangle is filled. The program should create a Triangle object with these sides and set the colour and filled properties using the input. The program should display the area, perimeter, colour, and true or false to indicate whether it is filled or not.arrow_forward(Triangle class) Design a new Triangle class that extends the abstract Geometricobject class. Draw the UML diagram for the classes Triangle and Geometricobject then implement the Triangle class. Write a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filed. The program should create a Tri- angle object with the se sides, and set the color and filled properties using the input. The program should display the area, perimeter, color, and true or false to indicate whether it is filled or not.arrow_forward(The Account class) Account -id: int -balance: double -annualInterestRate : double -dateCreated : Date +Account( ) +Account(someId : int, someBalance : double) +getId() : int +setId(newId : int) : void +getBalance() : double +setBalance(newBalance : double) : void +getAnnualInterestRate( ) : double +setAnnualInterestRate(newRate : double) : void +getDateCreated( ) : Date +getMonthlyInterestRate( ) : double +getMonthlyInterest( ) : double +withdraw(amt : double) : void +deposit(amt : double) : void Design a class named Account that contains: ■ A private int data field named id for the account (default 0). ■ A private double data field named balance for the account (default 0). ■ A private double data field named annualInterestRate that stores the current interest rate (default 0). Assume all accounts have the same interest rate. Make it static. ■ A private Date data field named dateCreated that stores the date when the account was created. (private Date dateCreated)…arrow_forward
- (Java) Open up Eclipse and create a new class called ArrayListPractice.java Next, copy and paste the below program into your file and run the code. Your job is to take the given code, remove all the arrays and replace them with the identical ArrayLists. There should be no arrays in your program. You will need to call the ArrayList methods as defined in the lesson notes above. Note that you will not be able to do method overloading with ArrayLists so you should assign different names to your methods. Once you have made the changes, you should get identical output as the given version of the program. Submit your program when you are finished. /** * @author * CIS 36B * Activity 5.2 */import java.util.ArrayList;import java.util.Scanner;public class ArrayListPractice { public static void main(String[] args) { int scores[] = {95, 96, 97, 98, 99}; System.out.println("Integer exam scores:"); print(scores); System.out.println();…arrow_forward(Java) Open up Eclipse and create a new class called ArrayListPractice.java Next, copy and paste the below program into your file and run the code. Your job is to take the given code, remove all the arrays and replace them with the identical ArrayLists. There should be no arrays in your program. You will need to call the ArrayList methods as defined in the lesson notes above. Note that you will not be able to do method overloading with ArrayLists so you should assign different names to your methods. Once you have made the changes, you should get identical output as the given version of the program. Submit your program when you are finished. /** * @author * CIS 36B * Activity 5.2 */ import java.util.ArrayList; import java.util.Scanner; public class ArrayListPractice { public static void main(String[] args) { int scores[] = {95, 96, 97, 98, 99}; System.out.println("Integer test scores:"); print(scores); System.out.println();…arrow_forward(Java) Question 5 Explain the answer step-by-step and include verbal explanation. Thank you! Write an interface as follows: The interface is named ServiceReminder It has one method named timeForService that has no parameters and returns a boolean variable. Now, update the below class so that is inherits from ServiceReminder Note that the next service date should be 90 days from the last service public abstract class Car { private double gasGauge; private double currMileage; private String color; private String make; private String model; private int daysLastService; public Car(String color, String make, String model, int daysLastService) { this.color = color; this.make = make; this.model = model; gasGauge = 0.0; currMileage = 0.0; this.daysLastService = daysLastService; } @Override public String toString() { return "Make: " + make + "\nModel: " + model + "\nColor: " + color…arrow_forward
- (The ComparableSquare class) Define a class named ComparableSquare thatextends Square and implements Comparable. Implement the compareTo methodto compare the Squares on the basis of area. Write a test class to find the larger oftwo instances of ComparableSquareobjects.arrow_forward(Find the nonleaves) Java Define a new class named BSTWithNumberOfNonLeaves that extends BST with the following methods: /** Return the number of nonleaf nodes */public int getNumberofNonLeaves() // BEGIN REVEL SUBMISSION class BSTWithNumberOfNonLeaves<E> extends BST<E> { /** Create a default BST with a natural order comparator */ public BSTWithNumberOfNonLeaves() { super(); } /** Create a BST with a specified comparator */ public BSTWithNumberOfNonLeaves(java.util.Comparator<E> c) { super(c); } /** Create a binary tree from an array of objects */ public BSTWithNumberOfNonLeaves(E[] objects) { super(objects); } public int getNumberOfNonLeaves() { return getNumberOfNonLeaves(root); } /** Returns the number of non-leaf nodes */ private int getNumberOfNonLeaves(TreeNode<E> root) { // WRITE YOUR CODE HERE } } // END REVEL SUBMISSIONarrow_forwardProblem (Circle.java) Implement a class called Circle for representing a circle. The circle has two data members, a Point object representing the center of the circle and a float value representing the radius. Include appropriate constructors for your Circle class. Encapsulate it. Also include methods for finding the area and circumference of the circle. area = pi * radius * radius circumference = 2 * pi * radius. (You may use the static constant pi in the Math class) 5. Override the toString() and equals().arrow_forward
- Edit only the class definition. DO NOT CHANGE the code given under 'main' please. Steps: Additionally implement any Python Magic/Dunder methods such that instances of the class minimally:1. Support addition, subtraction, equality operations and the built-in abs function2. Are Iterable i.e., support for loops and star arguments for unpacking into function calls3. Support a string representation that displays the class name and coordinates stored by the instance: i.e. for an object initialized as: Vector(0, 3), the string representation should be ‘Vector(0, 3)’The code given under main tests for each of the program requirements and subsequently uses the turtle module to plot randomly generated points rotated. A screenshot for a sample run of the program is attached. Template.py: from math import hypot, pi from random import uniform import turtle as t class Vector2D: ... if __name__ == '__main__': # Test Vector class a, b = Vector2D(0, 3), Vector2D(0, -3) tests =…arrow_forward(Intro to Java) Explain the answers to the below questions. include a written answer to the question using step-by-step explanation 4. Write the linearSearch method as follows using the algorithm provided in class: The method is named linearSearch It takes on array of doubles as a parameter It takes a double value to locate in the array as a second parameter It returns the integer location (index) in the array where the value is located Or, it returns -1 if the value cannot be foundarrow_forward*In Java Problem Description and Given Info Write a public static method named countGreaterThanAverage that will take an int array as it's only argument. This method will return an int value. When called, and passed an array of int values, this method will compute and return the number of values in the argument array that are greater than the average of all the values in the argument array. Here are some examples: Example 1 Given: int[] myArray = {1, 2, 3, 4, 5}; countGreaterThanAverage(myArray) should return 2 Example 2 Given: int[] myArray = {13, 7, 6, 5, 99, 10, 4, 8}; countGreaterThanAverage(myArray) should return 1 Example 3 Given: int[] myArray = {1, 1, 1}; countGreaterThanAverage(myArray) should return 0 Helpful Info: You may want to write a main method to call and test your required method There should be no System.out.print or System.out.println statements in your countGreaterThanAverage method There should be no calls to any Scanner methods (like next, nextLine, or…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
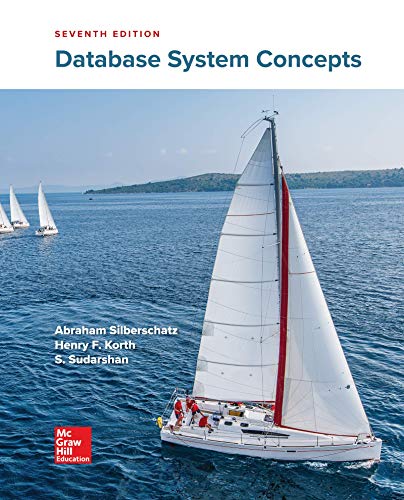
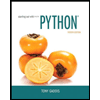
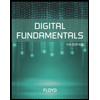
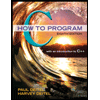
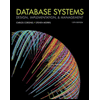
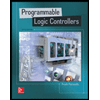