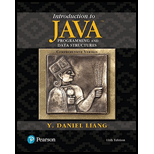
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 10, Problem 10.9PE
(The Course class) Revise the Course class as follows:
- Revise the getStudents() method to return an array whose length is the same as the number of students in the course, (Hint: create a new array and copy students to it.)
- The array size is fixed in Listing 10.6. Revise the addStudent method to automatically increase the array size if there is no room to add more students. This is done by creating a new larger array and copying the contents of the current array to it.
- Implement the dropStudent method.
- Add a new method named clear() that removes all students from the course.
Write a test
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Shift Right k Cells (Use Python)
Consider an array named source. Write a method/function named shifRight( source, k) that shifts all the elements of the source array to the right by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly.
Example:
source=[10,20,30,40,50,60]
shiftRight(source,3)
After calling shiftRight(source,3), printing the array should give the output as:
[ 0,0,0,10,20,30 ]
A bit new to methods and need a little bit of help.
Swap Method
Your task is to write a public static method named swap that takes a single parameter which is an array of doubles. This method should swap the first and last element in the array and then return the adjusted double array.
You can assume that all arrays passed into the method are a length of 2 or more.
For example, the test data provided when passed into the swap method should go from:
{8.34, 7.221, 10.643, 93.2}
to
Method Details:
public static void rotate(int[] array, boolean leftRotation, int positions)
Rotates the provided array left if leftRotation is true; right otherwise. The number of positions to rotate is determined by positions. For example, rotating the array 10, 20, 7, 8 two positions to the left will update the array to 7, 8, 10, 20. Only arrays with 2 or more elements will be rotated. Hint: adding private methods that rotate an array one position to the left and one position to the right can help.
Parameters:
array -
leftRotation -
positions -
Throws:java.lang.IllegalArgumentException - When a null array parameter is provided
Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 10.2 - Prob. 10.2.1CPCh. 10.3 - Is the BMI class defined in Listing 10.4...Ch. 10.4 - Prob. 10.4.1CPCh. 10.4 - Prob. 10.4.2CPCh. 10.4 - Prob. 10.4.3CPCh. 10.4 - Prob. 10.4.4CPCh. 10.7 - Prob. 10.7.1CPCh. 10.7 - Prob. 10.7.2CPCh. 10.7 - How do you convert an integer into a string? How...Ch. 10.7 - Show the output of the following code: public...
Ch. 10.7 - Prob. 10.7.5CPCh. 10.8 - What are autoboxing and autounboxing? Are the...Ch. 10.8 - Show the output of the following code. public...Ch. 10.9 - What is the output of the following code? public...Ch. 10.10 - Suppose s1, s2, s3, and s4 are four strings, given...Ch. 10.10 - To create the string Welcome to Java, you may use...Ch. 10.10 - What is the output of the following code? String...Ch. 10.10 - Let s1 be Welcome and s2 be welcome Write the...Ch. 10.10 - Prob. 10.10.5CPCh. 10.10 - Prob. 10.10.6CPCh. 10.10 - Prob. 10.10.7CPCh. 10.10 - Prob. 10.10.8CPCh. 10.10 - What is wrong in the following program? 1public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.11 - Prob. 10.11.1CPCh. 10.11 - Prob. 10.11.2CPCh. 10.11 - Prob. 10.11.3CPCh. 10.11 - Prob. 10.11.4CPCh. 10.11 - Prob. 10.11.5CPCh. 10.11 - Suppose s1 and s2 are given as fot tows:...Ch. 10.11 - Show the output of the following program: public...Ch. 10 - (The Time class) Design a class named Time. The...Ch. 10 - (The BMI class) Add the following new constructor...Ch. 10 - (The MyInteger class) Design a class named...Ch. 10 - Prob. 10.4PECh. 10 - (Display the prime factors) Write a program that...Ch. 10 - (Display the prime numbers) Write a program that...Ch. 10 - Prob. 10.7PECh. 10 - Prob. 10.8PECh. 10 - (The Course class) Revise the Course class as...Ch. 10 - Prob. 10.10PECh. 10 - Prob. 10.11PECh. 10 - (Geometry: the Triangle2D class) Define the...Ch. 10 - (Geometry: the MyRectangle 2D class) Define the...Ch. 10 - (The MyDate class) Design a class named MyDate....Ch. 10 - (Geometry: the bounding rectangle) A bounding...Ch. 10 - (Divisible by 2 or 3) Find the first 10 numbers...Ch. 10 - (Square numbers) Find the first 10 square numbers...Ch. 10 - (Mersenne prime)A prime number is called a...Ch. 10 - (Approximate e) Programming Exercise 5.26...Ch. 10 - (Divisible by 5 or 6) Find the first 10 numbers...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the Character class) The Character...Ch. 10 - (New string split method) The split method in the...Ch. 10 - (Implement the StringBuilder class) The...Ch. 10 - (Implement the StringBuilder class) The...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
The ____________ is always transparent.
Web Development and Design Foundations with HTML5 (8th Edition)
Assume a telephone signal travels through a cable at two-thirds the speed of light. How long does it take the s...
Electric Circuits. (11th Edition)
Determine the slope of the simply supported beam at A. E = 200 GPa and I = 39.9(106) m4. F126
Mechanics of Materials (10th Edition)
In many programming languages, the _____ key word creates an object in memory. a. Create b. New c. Instantiate ...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
In multiple inheritance, the derived class should always __________ a function that has the same name in more t...
Starting Out with C++ from Control Structures to Objects (9th Edition)
True or False: The superclass constructor always executes before the subclass constructor.
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Exercise (Array and Method): Write a method called arrayToString(), which takes an int array and return a String in the form of {a1, a2, ..., an}. Take note that there is no comma after the last element. The method's signature is as follows: public static String arrayToString(int[] array) Also write a test driver to test this method (you should test on empty array, one-element array, and n-element array). Notes: This is similar to the built-in function Arrays.toString(). You could study its source code. Exercise (Array and Method): Write a boolean method called contains(), which takes an array of int and an int; and returns true if the array contains the given int. The method's signature is as follows: public static boolean contains(int[] array, int key) Also write a test driver to test this method. Exercise (Array and Method): Write a method called search(), which takes an array of int and an int; and returns the array index if the array contains the given int; or -1 otherwise. The…arrow_forward2. Rotate Left k cells Use Python Consider an array named source. Write a method/function named rotateLeft( source, k) that rotates all the elements of the source array to the left by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] rotateLeft(source,3) After calling rotateLeft(source,3), printing the array should give the output as: [ 40, 50, 60, 10, 20, 30]arrow_forward3. Remove an element from an array Consider an array named source. Write a method/function named remove( source, size, idx) that removes the element in index idx of the source array. You must execute the method by passing an array, its size and the idx( that is the index of the element to be removed). After calling the method, print the array to show whether the element of that particular index has been removed properly. Example: source=[10,20,30,40,50,0,0] remove(source,5,2) After calling remove(source,5,2) , printing the array should give the output as: [ 10,20,40,50,0,0,0] [Use Python and Don't use Built-in function like "pop"]arrow_forward
- 3. Remove an element from an array Consider an array named source. Write a method/function named remove( source, size, idx) that removes the element in index idx of the source array. You must execute the method by passing an array, its size and the idx( that is the index of the element to be removed). After calling the method, print the array to show whether the element of that particular index has been removed properly. Example: source=[10,20,30,40,50,0,0] remove(source,5,2) After calling remove(source,5,2) , printing the array should give the output as: [ 10,20,40,50,0,0,0] Use Python languagearrow_forwardQuestion: 1. Write a method that has an int parameters. Creates an array of integers of that size. Reads in values for the array from the user. Returns the newly created array. 2 Write a method that given an array of integers.returns the index of the smallest integer.arrow_forwardPosted it 5th time if u answer wrong this time I’ll do downvote and bad review tooarrow_forward
- Create a class containing the main method. In the main method, create an integer array and initialize it with the numbers: 1,3,5,7,9,11,13,15,17,19 Pass the array as an argument to a method. Use a loop to add 1 to each element of the array and return the array to the main method. In the main method, use a loop to add the array elements and display the result. Note: In the loops, use the array field that holds the length of the array and do not use a number for array length.arrow_forwardJava: Write a complete method to create an array of random integers. Method Specification The method takes in three integer parameters: the array size, the lower bound, and the upper bound. The method also takes in a boolean parameter. The method creates and returns an integer array of the specified size that contains random numbers between the lower and upper bound., using these rules: The lower bound is always inclusive. If the boolean parameter is true, the upper bound is also inclusive. If the boolean parameter is false, the upper bound is not inclusive (meaning it is exclusive). If any of the parameters are invalid, null is returned. Driver/Tester Program I have provided you with a driver/tester program that you can use to test your code before submitting. Paste your code into the placeholder at the top of the file. Review the Homework FAQ page, which contains instructions and a video of how to use the provided file. If you aren't sure how to use the tester or have any…arrow_forwardJava: Write a complete method to create an array of random integers. Method Specification The method takes in three integer parameters: the array size, the lower bound, and the upper bound. The method also takes in a boolean parameter. The method creates and returns an integer array of the specified size that contains random numbers between the lower and upper bound., using these rules: The lower bound is always inclusive. If the boolean parameter is true, the upper bound is also inclusive. If the boolean parameter is false, the upper bound is not inclusive (meaning it is exclusive). If any of the parameters are invalid, null is returned. Driver/Tester Program I have provided you with a driver/tester program that you can use to test your code before submitting. Paste your code into the placeholder at the top of the file. I strongly recommend running this program before you submit your homework. The program is designed to help you catch common errors and fix them before submitting.…arrow_forward
- InsertNum(int num){} Write the method body of above method such that: 1. It checks first if the array is sorted or not. If array is sorted than it is self intelligent to place that user entered num at desired place in an array i.e if array is {2,3,5,7} and the number entered is 6 than it will place the number after 5 and increment the size and moves 7 to the right. 2. In case of unsorted array it will place the number at the end of array in constant time. (BigO(1)) Write code in C++ and dont use pointers.arrow_forwardExercise 2: Write array methods that carry out the following tasks for an array of integers by creating and completing the "ArrayMethods" class below. Add documentation comments for each method. Provide a test program called Labs_yourID.java" that tests methods of ArrayMethods class. In your test program, use random class to generate array values. public class ArrayMethods prívate int[ ] values: //declare instant variables public ArrayMethods (int[] initialValues) (values = initialValues:) //constructor public void shiftRight() () public boolean ad jacentDuplicate() () ... • Method shiftRight: that shifts all elements by one to the right and move the last element into the first position. For example, 1 49 16 25 would be transformed into 25 1 4 19 16. • Method ad jacentDuplicate: returns true if the array has two adjacent duplicate elements.arrow_forwardDNA Max Write code to find which of the strands representing DNA in an array String[] strands representing strands of DNA has the most occurrences of the nucleotide represented by parameter nuc. Complete the definition of the class DnaMax and method definition maxStrand shown below. If more than one strand has the same maximal number of the specified nucleotide you should return the longest strand with the maximal number. All DNA strands have different lengths in this problem so the maximal strand will be unique when length is accounted for. Return this uniquely maximal strand. Each String representing a DNA strand will contain only cytosine, guanine, thymine, and adenine, represented by the characters 'c', 'g', 't', and 'a', respectively. If no strand in the array contains the specified nucleotide return the empty string "". public class DnaMax { public String maxStrand(String[] strands, String nuc) { // fill in code here } } Constraints strands will contain no more than 50 elements,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
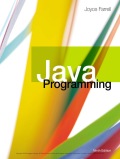
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
9.1: What is an Array? - Processing Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=NptnmWvkbTw;License: Standard Youtube License