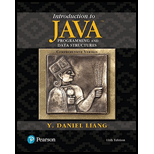
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 10.11, Problem 10.11.4CP
Program Plan Intro
- Define the required public class.
- Define the main function in the class using public static void main(String[] args).
- Create string object using StringBuilder sb = new StringBuilder();method.
- Delete the required substring using sb.delete(x,y); method.
- Print the string using System.out.println() .
- Close the main function.
- Define the main function in the class using public static void main(String[] args).
- Close the public class definition.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
True/False 7. The split method breaks a string into a list of substrings, and j oin does the opposite.
public static String pancakeScramble(String text) This nifty little problem is taken from the excellent Wolfram Challenges problem site where you can also see examples of what the result should be for various arguments. Given a text string, construct a new string by reversing its first two characters, then reversing the first three characters of that, and so on, until the last round where you reverse your entire current string.This problem is an exercise in Java string manipulation. For some mysterious reason, the Java String type does not come with a reverse method. The canonical way to reverse a Java string str is to first convert it to mutable StringBuilder, reverse its contents, and convert the result back to an immutable string, that is,str = new StringBuilder(str).reverse().toString();
Here's the tester it must pass:
@Test public void testPancakeScramble() throws IOException {// Explicit test casesassertEquals("", P2J3.pancakeScramble(""));assertEquals("alu",…
What happens if you try to retrieve a character in a string with an incorrect index?
Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 10.2 - Prob. 10.2.1CPCh. 10.3 - Is the BMI class defined in Listing 10.4...Ch. 10.4 - Prob. 10.4.1CPCh. 10.4 - Prob. 10.4.2CPCh. 10.4 - Prob. 10.4.3CPCh. 10.4 - Prob. 10.4.4CPCh. 10.7 - Prob. 10.7.1CPCh. 10.7 - Prob. 10.7.2CPCh. 10.7 - How do you convert an integer into a string? How...Ch. 10.7 - Show the output of the following code: public...
Ch. 10.7 - Prob. 10.7.5CPCh. 10.8 - What are autoboxing and autounboxing? Are the...Ch. 10.8 - Show the output of the following code. public...Ch. 10.9 - What is the output of the following code? public...Ch. 10.10 - Suppose s1, s2, s3, and s4 are four strings, given...Ch. 10.10 - To create the string Welcome to Java, you may use...Ch. 10.10 - What is the output of the following code? String...Ch. 10.10 - Let s1 be Welcome and s2 be welcome Write the...Ch. 10.10 - Prob. 10.10.5CPCh. 10.10 - Prob. 10.10.6CPCh. 10.10 - Prob. 10.10.7CPCh. 10.10 - Prob. 10.10.8CPCh. 10.10 - What is wrong in the following program? 1public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.11 - Prob. 10.11.1CPCh. 10.11 - Prob. 10.11.2CPCh. 10.11 - Prob. 10.11.3CPCh. 10.11 - Prob. 10.11.4CPCh. 10.11 - Prob. 10.11.5CPCh. 10.11 - Suppose s1 and s2 are given as fot tows:...Ch. 10.11 - Show the output of the following program: public...Ch. 10 - (The Time class) Design a class named Time. The...Ch. 10 - (The BMI class) Add the following new constructor...Ch. 10 - (The MyInteger class) Design a class named...Ch. 10 - Prob. 10.4PECh. 10 - (Display the prime factors) Write a program that...Ch. 10 - (Display the prime numbers) Write a program that...Ch. 10 - Prob. 10.7PECh. 10 - Prob. 10.8PECh. 10 - (The Course class) Revise the Course class as...Ch. 10 - Prob. 10.10PECh. 10 - Prob. 10.11PECh. 10 - (Geometry: the Triangle2D class) Define the...Ch. 10 - (Geometry: the MyRectangle 2D class) Define the...Ch. 10 - (The MyDate class) Design a class named MyDate....Ch. 10 - (Geometry: the bounding rectangle) A bounding...Ch. 10 - (Divisible by 2 or 3) Find the first 10 numbers...Ch. 10 - (Square numbers) Find the first 10 square numbers...Ch. 10 - (Mersenne prime)A prime number is called a...Ch. 10 - (Approximate e) Programming Exercise 5.26...Ch. 10 - (Divisible by 5 or 6) Find the first 10 numbers...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the Character class) The Character...Ch. 10 - (New string split method) The split method in the...Ch. 10 - (Implement the StringBuilder class) The...Ch. 10 - (Implement the StringBuilder class) The...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- language using - Javaarrow_forwardWhen working with String objects, they sometimes have extra spaces or otherformatting characters at the beginning or at the end of the string. The Trimand TrimEnd methods will remove spaces or other characters from either endof a string. You can specify either a single character to trim or an array ofcharacters. If you specify an array of characters, if any of the characters in thearray are found, they will be trimmed from the string.Write C# code that trims spaces from the beginning and end of a set of string values:arrow_forwardTask 2 Get Binary 1: Implement the get_bin_1 function as directed in the comment above the function. If the directions are unclear, run the code, and look at the expected answer for a few inputs. In order to be able to compare your solution to the answer, you cannot simply print out the binary directly to the console. Instead, you have been provided with a very rudimentary stringbuilder implementation that allows you to build a string one character at a time, without the ability to erase. There are comments at the top of the code explaining how to use the stringbuilder, however you could also see how it is being used in task_2_check. Note: if you create a stringbuilder and append ‘a’, ‘b’, then ‘c’, the resulting string will be “abc” when printed. You are free to read through the stringbuilder implementation, but it is quite complicated in order to make it easy to use. As specified in the comment, you may not use division (/) or mod (%) to implement this function (as the check…arrow_forward
- def q5(sentence): Assumes sentence is a string. Returns a list of all the words in sentence, where a word is a token separated by white space, (you can use sentence.split()), and then for each word, make it lowercase and remove any character that is not alpha-numeric (a-z or 8-9). For example, I q5("The Plague" (French: "La Peste"), 1947, by Albert CamUs.') should return ['the', 'plague', 'french', 'la', 'peste', '1947', 'by', 'albert', 'camus'] q5('Red@Dragon....ca is great!') should return ['reddragonca', 'is', 'great'] passarrow_forwardFix the error(s) in the following line of code.decRoyalTitle = strRoyalTitle(Text.StringLength)arrow_forwardQ1: Write a program that asks the user to enter their first name and their last name,separated by a space. Break the input string up into two strings, one containing thefirst name and one containing the last name. You can do that by using the indexOf()subroutine to find the position of the space, and then using substring() to extracteach of the two names. Also output the number of characters in each name andoutput the user’s initials. (The initials are the first letter of the first name togetherwith the first letter of the last name.) A sample run of the program should looksomething like this:Please enter your first name and last name, separated bya space.? Mary SmithYour first name is Mary, which has 4 charactersYour last name is Smith, which has 5 charactersYour initials are MSarrow_forward
- Write a program that reads in a line consisting of a student’s name, Social Security number, user ID, and password. The program outputs the string in which all the digits of the Social Security number and all the characters in the password are replaced by x. (The Social Security number is in the form 000-00-0000, and the user ID and the password do not contain any spaces.) Your program should not use the operator [] to access a string element. Use the appropriate functions described in Table 7-1 below. Expression Effect strVar.at(index) Returns the element at the position specified by index strVar[index] Returns the element at the position specified by index strVar.append(n, ch) Appends n copies of ch to strVar, where ch is a char variable or a char constant strVar.append(str) Appends str to strVar strVar.clear() Deletes all the characters in strVar strVar.compare(str) Returns 1 if strVar > str returns 0 if strVar == str; returns −1 if strVar < str…arrow_forwardWhy does the StringWriter class use a StringBuffer to perform its work?arrow_forwardWhat happens if you try to multiply a string by a string, "a" * "b"?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
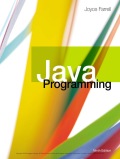
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT