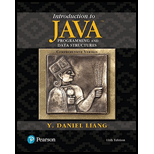
Concept explainers
(The Time class) Design a class named Time. The class contains:
- The data fields hour, minute, and second that represent a time.
- A no-arg constructor that creates a Time object for the current time. (The values of the data fields will represent the current time.)
- A constructor that constructs a Time object with a specified elapsed time since midnight, January 1, 1970, in milliseconds. (The values of the data fields will represent this time.)
- A constructor that constructs a Time object with the specified hour, minute, and second.
- Three getter methods for the data fields hour, minute, and second, respectively.
- A method named setTime(long elapseTime) that sets a new time for the object using the elapsed time. For example, if the elapsed time is 555550000 milliseconds, the hour is 10, the minute is 19, and the second is 10.
Draw the UML diagram for the class then implement the class. Write a test
(Hint: The first two constructors will extract the hour, minute, and second from the elapsed time. For the no-arg constructor, the current time can be obtained using System.currentTimeMillis(), as shown in Listing 2.7, ShowCuncntTime.java. Assume the time is in GMT.)

Program to print the current time with required data fields
Program Plan:
- Define the required public class.
- Define the main function in the class using public static void main(String[] args).
- Create the object to the class and pass the arguments.
- Print the data field using System.out.println() .
- Close the main function.
- Define the main function in the class using public static void main(String[] args).
- Close the public class definition.
- Define the required class.
- Define the integers
- Declare the public function.
- Define the setter method.
- Pass the arguments to the public function.
- Define the getter method.
- Define the void function.
- Compute the data fields.
- Close the main function.
- Close the public class definition.
Program Description:
The following java program has the class named Time and shows the current time with the data fields hour, minute and second respectively.
Explanation of Solution
Program:
//Class definition
public class Time {
//Define main function
public static void main(String[] args) {
//Create object to the class and assign the arguments
MyTime time1 = new MyTime();
//Print the data field
System.out.println(time1.getHour() + ":" +
time1.getMinute() + ":" + time1.getSecond());
//Create object to the class and assign the arguments
MyTime time2 = new MyTime(555550000);
//Print the data field
System.out.println(time2.getHour() + ":" +
time2.getMinute() + ":" + time2.getSecond());
//Create object to the class and assign the arguments
MyTime time3 = new MyTime(5, 23, 55);
//Print the data field
System.out.println(time3.getHour() + ":" +
time3.getMinute() + ":" + time3.getSecond());
}
}
//Class definition
class MyTime {
//Define the integer
private int hour;
private int minute;
private int second;
//Declare public function
public MyTime() {
this(System.currentTimeMillis());
}
//Declare public method
public MyTime(long elapsedTime) {
//Define setter method
setTime(elapsedTime);
}
//Pass the arguments to the public function
public MyTime(int hour, int minute, int second) {
this.hour = hour;
this.minute = minute;
this.second = second;
}
//Define getter method
public int getHour() {
return hour;
}
//Define getter method
public int getMinute() {
return minute;
}
//Define getter method
public int getSecond() {
return second;
}
//Define void function
public void setTime(long elapsedTime) {
// Obtain the total seconds since the midnight, Jan 1, 1970
long totalSeconds = elapsedTime / 1000;
// Compute the current second in the minute in the hour
second = (int)(totalSeconds % 60);
// Obtain the total minutes
long totalMinutes = totalSeconds / 60;
// Compute the current minute in the hour
minute = (int)(totalMinutes % 60);
// Obtain the total hours
int totalHours = (int)(totalMinutes / 60);
// Compute the current hour
hour = (int)(totalHours % 24);
}
}
17:57:23
10:19:10
5:23:55
Want to see more full solutions like this?
Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Management Information Systems: Managing The Digital Firm (16th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Introduction To Programming Using Visual Basic (11th Edition)
Database Concepts (8th Edition)
- My daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forwarda. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 1 1 0 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report.arrow_forward
- Using XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forwardQ4) A thin ring of radius 5 cm is placed on plane z = 1 cm so that its center is at (0,0,1 cm). If the ring carries 50 mA along a^, find H at (0,0,a).arrow_forward4. [15 pts] A logic function F of four variables a; b; c; d is described by the following K-map. Derive the fully minimized SOP logic expression form of F. cd ab 00 01 11 10 00 0 0 0 1 01 1 0 0 1 11 1 0 1 1 10 0 0 1 1arrow_forward
- 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisimarrow_forwardHow would I go about creating this computer database in MariaDB with sql? Create a database name "dbXXXXXX" Select the database using the "use [database name]" command. Now you are in the database. Based on the above schema from Enrolment System database, create all the tables with the last 6 digits of "123456", then the table name for table Lecturer should be "123456_Lecturer". Refer to basic SQL lecture note to create table that has primary keys and Foreign Keys. Provide the datatype of each attributes. Add a column called "Department" with datatype "VARCHAR(12)" to the table "Lecturer". Shows the metadata of the updated "Lecturer" table. (Use Describe command) Drop the "Department" column from the table "Lecturer", and show the metadata of the updated "Lecturer" table. Insert three (3) data to each of the table in the tables created. Note: If you have foreign key issues, please disable foreign key constraints before inserting the data, see below SET FOREIGN_KEY_CHECKS=0;…arrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 3. Consider the real number x = (3.395) 10 (a) (b) Convert the decimal number x into binary format up to 7 binary places (7 binary digits after decimal) Convert the calculated value into denormalized form and calculate fl(x) for m=4 Don't use any Al tool show answer in pen a nd paper then take pi ctures and sendarrow_forward
- Simplify the following expressions by means of a four-variable K-Map. AD+BD+ BC + ABDarrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 2. Let ẞ 2, m = 6, emin = -3 and emax = 3. Answer the following questions: Compute the minimum of |x| for General and Normalized form (a) Compute the Machine Epsilon value for the General and Denormalized form. If we change the value of emax to 6 then how will it affect the value of maximum scale invariant error for the case of Normalized form? Explain your answer. show answer in pen a Don't use any Al tool nd paper then take pi ctures and sendarrow_forwardCSE330: Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. Now, let's take, ẞ = 2, m = 3, emin = -2 and emax = 3. Based on these, answer the following: (a) (b) (c) (d) What are the maximum/largest numbers that can be stored in the system by these three forms defined above? (express your answer in decimal values) What are the non-negative minimum/smallest numbers that can be stored in the system by the denormalized form? (express your answer in decimal values) How many numbers (both non-negative and negative) can be represented in the above mentioned system using the general form? Explain your answer. Find all the decimal numbers for e = 3 and e = 2 in denormalized form, plot them on a real line and prove that all the numbers are not equally spaced. Write the equally spaced sets for the number line you drew. show your answer in Don't use any Al tool pen…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
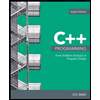
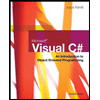
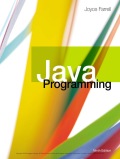
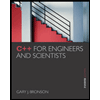
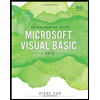