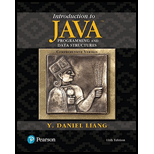
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 10, Problem 10.3PE
(The MyInteger class) Design a class named MyInteger. The class contains:
- An int data field named value that stores the int value represented by this object.
- A constructor that creates a MyInteger object for the specified int value.
- A getter method that returns the int value.
- The methods isEven(), isOdd(),and isPrime() that return true if the value in this object is even, odd, or prime, respectively.
- The static methods isEven(int), isOdd(int),and isPrime(int) that return true if the specified value is even, odd, or prime, respectively.
- The static methods isEven(MyInteger), isOdd(MyInteger), and isPrime(MyInteger) that return true if the specified value is even, odd, or prime, respectively.
- The methods equals(int) and equals(MyInteger) that return true if the value in this object is equal to the specified value.
- A static method parseInt (char[]) that converts an array of numeric characters to an int value.
- A static method parseInt(String) that converts a string into an int value.
Draw the UML diagram for the class then implement the class. Write a client
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8)
2. Read-And-Analyze: Given the code below, answer the following questions.
2
1 import java.util.Scanner;
3 public class Array2DPractice {
4
5
6
7
8
9
10
11
12
13
14
15
16
public static void main(String args[]) {
17 }
18
// Get an array from the user
int[][] m = getArray();
// Display array elements
System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m));
// Display array characteristics
int[] r = findCharacteristics(m);
System.out.println("The minimum value is: " + r[0]);
System.out.println("The maximum value is: " + r[1]);
System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length));
19 // Create an array from user input
public static int[][] getArray() {
20
21
PASSTR2222322222222222 222323 F F F F
44
// Create a Scanner to read user input
Scanner input = new Scanner(System.in);
// Ask user to input a number, and grab that number with the Scanner…
Given the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined
Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF
Consider the ER diagram of online sales system above. Based on the diagram answer the questions below,
1. Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key
2. Is there a direct relationship that exists between Store and Customer entities? AnswerYes/No?
Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 10.2 - Prob. 10.2.1CPCh. 10.3 - Is the BMI class defined in Listing 10.4...Ch. 10.4 - Prob. 10.4.1CPCh. 10.4 - Prob. 10.4.2CPCh. 10.4 - Prob. 10.4.3CPCh. 10.4 - Prob. 10.4.4CPCh. 10.7 - Prob. 10.7.1CPCh. 10.7 - Prob. 10.7.2CPCh. 10.7 - How do you convert an integer into a string? How...Ch. 10.7 - Show the output of the following code: public...
Ch. 10.7 - Prob. 10.7.5CPCh. 10.8 - What are autoboxing and autounboxing? Are the...Ch. 10.8 - Show the output of the following code. public...Ch. 10.9 - What is the output of the following code? public...Ch. 10.10 - Suppose s1, s2, s3, and s4 are four strings, given...Ch. 10.10 - To create the string Welcome to Java, you may use...Ch. 10.10 - What is the output of the following code? String...Ch. 10.10 - Let s1 be Welcome and s2 be welcome Write the...Ch. 10.10 - Prob. 10.10.5CPCh. 10.10 - Prob. 10.10.6CPCh. 10.10 - Prob. 10.10.7CPCh. 10.10 - Prob. 10.10.8CPCh. 10.10 - What is wrong in the following program? 1public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.11 - Prob. 10.11.1CPCh. 10.11 - Prob. 10.11.2CPCh. 10.11 - Prob. 10.11.3CPCh. 10.11 - Prob. 10.11.4CPCh. 10.11 - Prob. 10.11.5CPCh. 10.11 - Suppose s1 and s2 are given as fot tows:...Ch. 10.11 - Show the output of the following program: public...Ch. 10 - (The Time class) Design a class named Time. The...Ch. 10 - (The BMI class) Add the following new constructor...Ch. 10 - (The MyInteger class) Design a class named...Ch. 10 - Prob. 10.4PECh. 10 - (Display the prime factors) Write a program that...Ch. 10 - (Display the prime numbers) Write a program that...Ch. 10 - Prob. 10.7PECh. 10 - Prob. 10.8PECh. 10 - (The Course class) Revise the Course class as...Ch. 10 - Prob. 10.10PECh. 10 - Prob. 10.11PECh. 10 - (Geometry: the Triangle2D class) Define the...Ch. 10 - (Geometry: the MyRectangle 2D class) Define the...Ch. 10 - (The MyDate class) Design a class named MyDate....Ch. 10 - (Geometry: the bounding rectangle) A bounding...Ch. 10 - (Divisible by 2 or 3) Find the first 10 numbers...Ch. 10 - (Square numbers) Find the first 10 square numbers...Ch. 10 - (Mersenne prime)A prime number is called a...Ch. 10 - (Approximate e) Programming Exercise 5.26...Ch. 10 - (Divisible by 5 or 6) Find the first 10 numbers...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the Character class) The Character...Ch. 10 - (New string split method) The split method in the...Ch. 10 - (Implement the StringBuilder class) The...Ch. 10 - (Implement the StringBuilder class) The...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Figure 4-3212 shows a class list for Millennium College. Convert this user view to a set of 3NF relations using...
Modern Database Management
Why is the study of database technology important?
Database Concepts (8th Edition)
Assume a telephone signal travels through a cable at two-thirds the speed of light. How long does it take the s...
Electric Circuits. (11th Edition)
Why is aluminum oxide used more frequently than silicon carbide as an abrasive?
Degarmo's Materials And Processes In Manufacturing
What is the difference between the names defined in an ML let construct from the variables declared in a C bloc...
Concepts Of Programming Languages
What is an object?
Starting Out With Visual Basic (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Consider the ER diagram of online sales system above. Based on the diagram answer thequestions below, 1. Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M 2. Which one of the 4 Entities mention in the diagram can have a recursive relationship? 3. If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)? NO AI use pencil and paperarrow_forwardSTEP 1: The skeleton Let's start by creating a skeleton for some of the classes you will need. • Write a class called Tile. You can think of a tile as a square on the board on which the game will be played. We will come back to this class later. For the moment you can leave it empty while you work on creating classes that represents characters in the game. • Write an abstract class Fighter which has the following private fields: - A Tile field named position, representing the fighter's position in the game. A double field named health, representing the fighter's health points (HP). An int field named weaponType, representing the type of weapon the fighter is using. This value is used to rank different weapon types: higher values indicate higher weapon ranks. -An int field named attackDamage, representing the fighter's attack power. The class must also have the following public methods: 3 A constructor that takes as input a Tile indicating the position of the fighter, a double…arrow_forwardA company database needs to store information about employees (identified by SIN, with salary and phone as attributes), departments (identified by DID, with dname and budget as attributes), and children of employees (with name and age as attributes). Employees work in departments; each department is managed by an employee; a child must be identified uniquely by name when the parent (who is an employee; assume that only one parent works for the company) is known. We are not interested in information about a child once the parent leaves the company. Draw an ER diagram using Crows Foot notation that captures this information. Important: Must submit both ER Diagram and Relational Schema images in your solution here.arrow_forward
- Given the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined. Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NF]arrow_forward1. Using one of the method described in class and/or textbook (Section 9.1) convert the following regular expression into a state transition diagram: (0+ 10*1)* (01 + 10) Indicate in your answer how did you arrive at the result as follows: Write down all the state transition diagrams that you constructed for all the subexpressions and clearly indicate which diagram corresponds to which expression. Do not simplify any state transition diagram. 2. Consider the following state transition diagram over Σ = {a,b}: b A a a C b B a a b D За a Using the method described in class and in the textbook (Section 9.2) convert the diagram into an equivalent regular expression. Include all the intermediate steps in your answer. 3. Are the languages L1, L2, and L3 below over the alphabet Σ = {a, b, c} regular or non-regular? Justify your answer carefully. (a) L₁ = {a¹b2jc²i : i ≥ 0, j > 2} (b) L₂ = L₁n {akbm c³p: k,m,p≥ 0} (c) L3 = {a²ib²j+1 : i,j ≥ 0}^{akbm c³p : k,m,p ≥ 0}arrow_forward(1 point) By dragging statements from the left column to the right column below, give a proof by induction of the following statement: an = = 9" - 1 is a solution to the recurrence relation an = 9an-18 with ao = : 0. The correct proof will use 8 of the statements below. Statements to choose from: Note that a₁ = 9a0 + 8. Now assume that P(n) is true for all n ≥ 0. Your Proof: Put chosen statements in order in this column and press the Submit Answers button. Let P(n) be the predicate, "a = 9″ – 1". απ = 90 − 1 = Note that Let P(n) be the predicate, "an 9" - 1 is a solution to the recurrence relation an = 9an-1 +8 with ao = 0." - Now assume that P(k + 1) is true. Thus P(k) is true for all k. Thus P(k+1) is true. Then ak+1 = 9ak +8, so P(k + 1) is true. = 1 − 1 = 0, as required. Then = 9k — 1. ak Now assume that P(k) is true for an arbitrary integer k ≥ 1. By the recurrence relation, we have ak+1 = ak+1 = = 9ak + 8 = 9(9k − 1) + 8 This simplifies to 9k+19+8 = 9k+1 − 1 Then 9k+1 − 1 = 9(9*…arrow_forward
- Given the following request to an AI chatbot, which response is more accurate? (You should use Google to verify that the factual claims in each response are accurate.) Prompt Is this true? The first recorded use of the phrase "when pigs fly" in print is in a 1616 play by Ben Jonson called "The Devil is an Ass," in which a character says, "When pigs fly, good-night!!" Response Response A Response B Yes, that's true! The phrase "when pigs fly" indeed appears in Ben Jonson's 1616 play The Devil is an Ass and was said during the second act in a conversation between Fitzdottrel and Pug. The phrase was first used to express skepticism or disbelief, suggesting that something is as unlikely to happen as the fanciful idea of pigs flying. This usage is often cited as one of the earliest instances of the phrase in print. The claim that the phrase "when pigs fly" was first recorded in Ben Jonson's 1616 play "The Devil is an Ass" is not accurate. There is no evidence from the…arrow_forwardThis is for my Computer Organization & Assembly Language Classarrow_forwardPlease answer the homework scenario below and make a JAVA OOP code. You have been hired by GMU to create and manage their course registration portal. Your first task is to develop a program that will create and track different courses in the portal. Each course has the following properties: • a course number ex. IT 106, IT 206, • A course description, ex. Intro to Programming • Total credit hour ex. 3.0, and • current enrollment ex. 30 Each course must have at least a course number and credit hours. The maximum enrollment for each course is 40 students. The current enrollment should be no greater than the maximum enrollment. A course can have a maximum of 4 credit hour. The DDC should calculate the number of seats remaining for the course. Design an object-oriented solution to create a data definition class for the course object. The course class must define all the constructors, mutators with proper validation, accessors, and special purpose methods. The DDC should calculate the…arrow_forward
- For this case study, students will analyze the ethical considerations surrounding artificial intelligence and big data in healthcare, as explored in the case study found in the textbook (pages 34-36) and in the extended version available here There will also be additional articles in this weeks learning module to show both sides of the coin. https://www.delftdesignforvalues.nl/wp-content/uploads/2018/03/Saving-the-life-of-medical-ethics-in-the-age-of-AI-and-Big-Data.pdf Students should refer to the syllabus for specific guidelines regarding length, format, and content requirements. Reflection Questions to Consider: What are the key ethical dilemmas presented in the case? How does AI challenge traditional medical ethics principles such as autonomy, beneficence, and confidentiality? In what ways can responsible innovation help address moral overload in healthcare decision-making? What are the potential risks and benefits of integrating AI-driven decision-making into patient care?…arrow_forwardCan you please solve this. Thanksarrow_forwardcan you solve this pleasearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
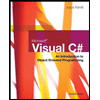
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
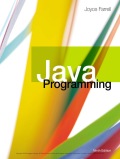
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
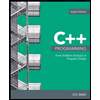
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
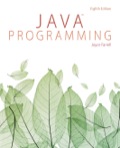
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
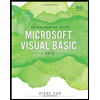
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Call By Value & Call By Reference in C; Author: Neso Academy;https://www.youtube.com/watch?v=HEiPxjVR8CU;License: Standard YouTube License, CC-BY