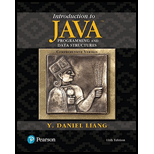
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 10.4PE
Program Plan Intro
Creation of MyPoint class with coordinates and display the distance between the points
Program Plan:
- Define the required public class definition.
- Define the main function in the class using public static void main(String[] args).
- Create the objects and pass the arguments.
- Print the data field using System.out.println().
- Close the main function.
- Define the main function in the class using public static void main(String[] args).
- Close the public class definition.
- Define the required class definition.
- Define private data fields.
- Declare the public method.
- Pass the parameters to the public method.
- Define the public method.
- Return the required value.
- Define the static method.
- Return the required value.
- Define for loop.
- Define if statement.
- Return the required value.
- Return the required value.
- Define the static method.
- Return the required value.
- Close the static method.
- Close the public class definition.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Suppose your computer is responding very slowly to information requests from the Internet. You observe that your network gateway shows high levels of network activity even though you have closed your e-mail client, Web browser, and all other programs that access the Internet.
What types of malwares could cause such symptoms? What steps can you take to check whether malware has gained access to your system? What tools can you use at each step? If you identify malware, what ways might it have entered your system? How can you restore your PC to safe operation, including the special software tools you may use?
R language
Using R language
Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 10.2 - Prob. 10.2.1CPCh. 10.3 - Is the BMI class defined in Listing 10.4...Ch. 10.4 - Prob. 10.4.1CPCh. 10.4 - Prob. 10.4.2CPCh. 10.4 - Prob. 10.4.3CPCh. 10.4 - Prob. 10.4.4CPCh. 10.7 - Prob. 10.7.1CPCh. 10.7 - Prob. 10.7.2CPCh. 10.7 - How do you convert an integer into a string? How...Ch. 10.7 - Show the output of the following code: public...
Ch. 10.7 - Prob. 10.7.5CPCh. 10.8 - What are autoboxing and autounboxing? Are the...Ch. 10.8 - Show the output of the following code. public...Ch. 10.9 - What is the output of the following code? public...Ch. 10.10 - Suppose s1, s2, s3, and s4 are four strings, given...Ch. 10.10 - To create the string Welcome to Java, you may use...Ch. 10.10 - What is the output of the following code? String...Ch. 10.10 - Let s1 be Welcome and s2 be welcome Write the...Ch. 10.10 - Prob. 10.10.5CPCh. 10.10 - Prob. 10.10.6CPCh. 10.10 - Prob. 10.10.7CPCh. 10.10 - Prob. 10.10.8CPCh. 10.10 - What is wrong in the following program? 1public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.11 - Prob. 10.11.1CPCh. 10.11 - Prob. 10.11.2CPCh. 10.11 - Prob. 10.11.3CPCh. 10.11 - Prob. 10.11.4CPCh. 10.11 - Prob. 10.11.5CPCh. 10.11 - Suppose s1 and s2 are given as fot tows:...Ch. 10.11 - Show the output of the following program: public...Ch. 10 - (The Time class) Design a class named Time. The...Ch. 10 - (The BMI class) Add the following new constructor...Ch. 10 - (The MyInteger class) Design a class named...Ch. 10 - Prob. 10.4PECh. 10 - (Display the prime factors) Write a program that...Ch. 10 - (Display the prime numbers) Write a program that...Ch. 10 - Prob. 10.7PECh. 10 - Prob. 10.8PECh. 10 - (The Course class) Revise the Course class as...Ch. 10 - Prob. 10.10PECh. 10 - Prob. 10.11PECh. 10 - (Geometry: the Triangle2D class) Define the...Ch. 10 - (Geometry: the MyRectangle 2D class) Define the...Ch. 10 - (The MyDate class) Design a class named MyDate....Ch. 10 - (Geometry: the bounding rectangle) A bounding...Ch. 10 - (Divisible by 2 or 3) Find the first 10 numbers...Ch. 10 - (Square numbers) Find the first 10 square numbers...Ch. 10 - (Mersenne prime)A prime number is called a...Ch. 10 - (Approximate e) Programming Exercise 5.26...Ch. 10 - (Divisible by 5 or 6) Find the first 10 numbers...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the Character class) The Character...Ch. 10 - (New string split method) The split method in the...Ch. 10 - (Implement the StringBuilder class) The...Ch. 10 - (Implement the StringBuilder class) The...
Knowledge Booster
Similar questions
- Compare the security services provided by a digital signature (DS) with those of a message authentication code (MAC). Assume that Oscar can observe all messages sent between Rina and Naseem. Oscar has no knowledge of any keys but the public one, in the case of DS. State whether DS and MAC protect against each attack and, if they do, how. The value auth(x) is computed with a DS or a MAC algorithm. In each scenario, assume the message M = x#####auth(x). (Message integrity) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message M, and then sends M in cleartext to Naseem. Oscar intercepts the message and replaces “Mark” with “Oscar.” Will Naseem detect this in the case of either DS or MAC? If yes, how will Naseem detect it? If not, why? (Replay) Rina has the textual data x = “Transfer $1000 to Mark” to send to Naseem. To ensure the integrity of the data, Rina generates auth(x), forms a message…arrow_forwardI need to resolve the following....You are trying to convince your boss that your company needs to invest in a license for MS-Project (project management software from Microsoft) before beginning a systems project. What arguments would you give her?arrow_forwardWhat are the four types of feasibility? what is the issues addressed by each feasibility component.arrow_forward
- I would like to get ab example of a situation where Agile Methods might be preferable versus the traditional SDLC? What are the characteristics of this situation that give Agile Methods an advantage?arrow_forwardWhat is a functional decomposition diagram? what is a good example of a high level task being broken down into tasks in at least two lower levels (three levels in all).arrow_forwardWhat are the advantages to using a Sytems Analysis and Design model like the SDLC vs. other approaches?arrow_forward
- 3. Problem Description: Define the Circle2D class that contains: Two double data fields named x and y that specify the center of the circle with get methods. • A data field radius with a get method. • A no-arg constructor that creates a default circle with (0, 0) for (x, y) and 1 for radius. • A constructor that creates a circle with the specified x, y, and radius. • A method getArea() that returns the area of the circle. • A method getPerimeter() that returns the perimeter of the circle. • • • A method contains(double x, double y) that returns true if the specified point (x, y) is inside this circle. See Figure (a). A method contains(Circle2D circle) that returns true if the specified circle is inside this circle. See Figure (b). A method overlaps (Circle2D circle) that returns true if the specified circle overlaps with this circle. See the figure below. р O со (a) (b) (c)< Figure (a) A point is inside the circle. (b) A circle is inside another circle. (c) A circle overlaps another…arrow_forward1. Explain in detail with examples each of the following fundamental security design principles: economy of mechanism, fail-safe default, complete mediation, open design, separation of privilege, least privilege, least common mechanism, psychological acceptability, isolation, encapsulation, modularity, layering, and least astonishment.arrow_forwardSecurity in general means the protection of an asset. In the context of computer and network security, explore and explain what assets must be protected within an online university. What the threats are to the security of these assets, and what countermeasures are available to mitigate and protect the organization from such threats. For each of the assets you identify, assign an impact level (low, moderate, or high) for the loss of confidentiality, availability, and integrity. Justify your answers.arrow_forward
- Please include comments and docs comments on the program. The two other classes are Attraction and Entertainment.arrow_forwardObject-Oriented Programming In this separate files. ent, you'll need to build and run a small Zoo in Lennoxville. All classes must be created in Animal (5) First, start by building a class that describes an Animal at a Zoo. It should have one private instance variable for the name of the animal, and one for its hunger status (fed or hungry). Add methods for setting and getting the hunger satus variable, along with a getter for the name. Consider how these should be named for code clarity. For instance, using a method called hungry () to make the animal hungry could be used as a setter for the hunger field. The same logic could be applied to when it's being fed: public void feed () { this.fed = true; Furthermore, the getter for the fed variable could be named is Fed as it is more descriptive about what it answers when compared to get Fed. Keep this technique in mind for future class designs. Zoo (10) Now we have the animals designed and ready for building a little Zoo! Build a class…arrow_forward1.[30 pts] Answer the following questions: a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 0 1 1 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report. 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisim in your report. 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB C…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
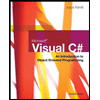
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
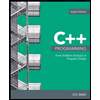
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
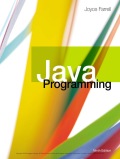
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
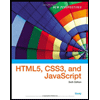
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
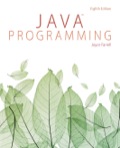
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT