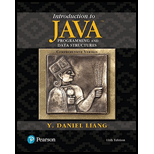
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 10, Problem 10.8PE
Program Plan Intro
Creation of a class named Tax to follow the required instance data fields
Program Plan:
- Define the required public class definition.
- Define the main function in the class using public static void main(String[] args).Define required constructor.
- Pass the required int variables.
- Pass the required double variables.
- Create the required object and pass the required arguments.
- Print the data field using System.out.println().
- Define for loop.
- Declare the required setter method.
- Print the data field using System.out.println().
- Close the main function.
- Define the main function in the class using public static void main(String[] args).Define required constructor.
- Close the public class definition.
- Define the required class definition.
- Declare the required static variables.
- Declare the required private int variables.
- Declare the required private double variables.
- Declare and define the required constructor.
- Declare the setter method.
- Declare the getter method.
- Declare the setter method.
- Return the required value.
- Declare the getter method.
- Return the required value.
- Declare the required constructor.
- Declare the getter method.
- Return the required value.
- Declare the setter method.
- Return the required value.
- Declare int varaibles.
- Declare if statement.
- Return the required value.
- Close the class definition
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ PROGRAMS:
Question#1:Define a class for rectangle objects defined by two points, the top-left and bottom-right corners of the rectangle. Include a constructor to copy a rectangle, a method to return a rectangle object that encloses the current object and the rectangle passed as an argument, and a method to display the defining points of a rectangle. Test the class by creating four rectangles and combining these cumulatively to end up with a rectangle enclosing them all. Output the defining points of all the rectangles you create.Question#2:Define a class, mcmLength, to represent a length measured in meters, centimeters, and millimeters, each stored as integers. Include methods to add and subtract objects, to multiply and divide an object by an integer value, to calculate an area resulting from the product of two objects, and to compare objects. Include constructors that accept three arguments—meters, centimeters, and millimeters; one integer argument in millimeters; one double…
[In c#]
Write a class with name Arrays . This class has an array which should be initialized by user.Write a method Sum that should sum even numbers in array and return sum. write a function with name numFind in this class with working logic as to find the mid number of an array. After finding this number calculate its factorial.Write function that should display sum and factorial.Don’t use divide operator
(After reading the instructions given in the pictures attached, read the following)
Class membersdoctorType Class must contain at least these functionsdoctorType(string first, string last, string spl); //First Name, Last Name, Specialty
void print() const; //Formatted Display First Name, Last Name, Specialtyvoid setSpeciality(string); //Set the doctor’s Specialtystring getSpeciality(); //Return the doctor’s SpecialtypatientType Class must contain at least these functionsvoid setInfo(string id, string fName, string lName,int bDay, int bMth, int bYear,string docFrName, string docLaName, string docSpl,int admDay, int admMth, int admYear,int disChDay, int disChMth, int disChYear);void setID(string);string getID();void setBirthDate(int dy, int mo, int yr);int getBirthDay();int getBirthMonth();int getBirthYear();void setDoctorName(string fName, string lName);void setDoctorSpl(string);string getDoctorFName();string getDoctorLName();string getDoctorSpl();void…
Chapter 10 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 10.2 - Prob. 10.2.1CPCh. 10.3 - Is the BMI class defined in Listing 10.4...Ch. 10.4 - Prob. 10.4.1CPCh. 10.4 - Prob. 10.4.2CPCh. 10.4 - Prob. 10.4.3CPCh. 10.4 - Prob. 10.4.4CPCh. 10.7 - Prob. 10.7.1CPCh. 10.7 - Prob. 10.7.2CPCh. 10.7 - How do you convert an integer into a string? How...Ch. 10.7 - Show the output of the following code: public...
Ch. 10.7 - Prob. 10.7.5CPCh. 10.8 - What are autoboxing and autounboxing? Are the...Ch. 10.8 - Show the output of the following code. public...Ch. 10.9 - What is the output of the following code? public...Ch. 10.10 - Suppose s1, s2, s3, and s4 are four strings, given...Ch. 10.10 - To create the string Welcome to Java, you may use...Ch. 10.10 - What is the output of the following code? String...Ch. 10.10 - Let s1 be Welcome and s2 be welcome Write the...Ch. 10.10 - Prob. 10.10.5CPCh. 10.10 - Prob. 10.10.6CPCh. 10.10 - Prob. 10.10.7CPCh. 10.10 - Prob. 10.10.8CPCh. 10.10 - What is wrong in the following program? 1public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.10 - Show the output of the following code: public...Ch. 10.11 - Prob. 10.11.1CPCh. 10.11 - Prob. 10.11.2CPCh. 10.11 - Prob. 10.11.3CPCh. 10.11 - Prob. 10.11.4CPCh. 10.11 - Prob. 10.11.5CPCh. 10.11 - Suppose s1 and s2 are given as fot tows:...Ch. 10.11 - Show the output of the following program: public...Ch. 10 - (The Time class) Design a class named Time. The...Ch. 10 - (The BMI class) Add the following new constructor...Ch. 10 - (The MyInteger class) Design a class named...Ch. 10 - Prob. 10.4PECh. 10 - (Display the prime factors) Write a program that...Ch. 10 - (Display the prime numbers) Write a program that...Ch. 10 - Prob. 10.7PECh. 10 - Prob. 10.8PECh. 10 - (The Course class) Revise the Course class as...Ch. 10 - Prob. 10.10PECh. 10 - Prob. 10.11PECh. 10 - (Geometry: the Triangle2D class) Define the...Ch. 10 - (Geometry: the MyRectangle 2D class) Define the...Ch. 10 - (The MyDate class) Design a class named MyDate....Ch. 10 - (Geometry: the bounding rectangle) A bounding...Ch. 10 - (Divisible by 2 or 3) Find the first 10 numbers...Ch. 10 - (Square numbers) Find the first 10 square numbers...Ch. 10 - (Mersenne prime)A prime number is called a...Ch. 10 - (Approximate e) Programming Exercise 5.26...Ch. 10 - (Divisible by 5 or 6) Find the first 10 numbers...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the String class) The String class is...Ch. 10 - (Implement the Character class) The Character...Ch. 10 - (New string split method) The split method in the...Ch. 10 - (Implement the StringBuilder class) The...Ch. 10 - (Implement the StringBuilder class) The...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Question 2: (TicTacToe Class) Create a class TicTacToe that will enable you to write a complete program to play the game of tic-tac-toe. The class contains as private data a 3-by-3 two-dimensional array of integers. The constructor should initialize the empty board to all zeros. Allow two human players. Wherever the first player moves, place a 1 in the specified square. Place a 2 wherever the second player moves. Each move must be to an empty square. After each move, determine whether the game has been won or is a draw. If you feel ambitious, modify your program so that the computer makes the moves for one of the players. Also, allow the player to specify whether he or she wants to go first or second.arrow_forwardC++ (Tic-Tac-Toe) Write a program that allows two players to play the tic- tac-toe game. Your program must contain the class ticTacToe to implement a ticTacToe objectarrow_forward- Create a struct called Complex for performing arithmetic with complex numbers. Write a driver program to test your struct. Complex numbers have the form: realPart + imaginaryPart * iwhere i is the square root of -1Use double variables to represent data of the struct. Provide a function that enables an object of this struct to be initialized when it is declared. The function should contain default values in case no initializers are provided. Also provide functions for each of the following:a) Addition of two Complex numbers: The real parts are added together and the imaginary parts are added together.b) Subtraction of two Complex numbers: The real part of the right operand is subtracted from the real part of the left operand and the imaginary part of the right operand is subtracted from the imaginary part of the left operand.c) Printing Complex numbers in the form (a, b) where a is the real part and b is the imaginary partSubmit one file which contains all code above: the structure…arrow_forward
- Can I please get help writing this in C++ Write a class called Word that stores a word from a product review in a data member called “word.” The class should also contain an integer variable representing the number of times (i.e. frequency) that the word was found in a product review document. The class should have a one-argument constructor that receives a pointer to a c-string (character array) containing the word as its one parameter. (Note that the output of the strtok_s function described above is a pointer to a c-string containing the word that was parsed. This is what you will pass in to the Word constructor.) The Word constructor should also set the frequency of this Word object to 1. Appropriate set and get functions should be included for both the word and frequency data members. Write a class called Review that contains a vector of objects of the Word class. The class should contain functions to add a new Word object to the vector and to print out all of the Words in…arrow_forwardstate the statement either true or false.arrow_forwardLanguage: C++ Create a class called publication that stores the title (char array) and price (float) of a publication. From this class derive two classes: book, which adds a page count (int) and tape, which adds a playing time in minutes (float). Each of the three classes should have a getdata() function to get its data from the user using input from the keyboard and a putdata() function to display the data.Write a main function that creates an array of pointers to publication. In a loop, ask the user for data about a particular type of book or tape to hold data. Put the pointer to the object in the array. When the user has finished entering data for all the books and tapes, display the resulting data for all the books and tapes entered, using a for loop and a single statement such as: pubarr[j] -> putdata(); To display the data from each object in the array.arrow_forward
- Q5: Correct the following code fragment and what will be the final results of the variable a and b class A {protected int x1,y1,z; public: A(a, b,c):x1(a+2),y1 (b-1),z(c+2) { for(i=0; i<5;i++) x1++;y1++;z++;}}; class B {protected: int x,y; public: B(a,b):x(a+1),y(b+2) { for(i=0; i<5;i++) x+=2; y+=1;}}; class D:public B, virtual public A { private: int a,b; public: D(k,m,n): a(k+n), B(k,m),b(n+2),A(k,m,n) { a=a+1;b=b+1;}); int main() {Dob(4,2,5);)arrow_forwardWrite in C++ Language. (Employee Record): Create a class named 'Staff' having the following members: Data members - Id – Name - Phone number – Address - AgeIt also has a function named 'printSalary' which prints the salary of the staff.Two classes 'Employee' and 'Officer' inherits the 'Staff' class. The 'Employee' and 'Officer' classes have data members 'Top Skill' and 'department' respectively. Now, assign name, age, phone number, address and salary to an employee and a officer by making an object of both of these classes and print the same.arrow_forwardC++ OOP * Use classes only.arrow_forward
- Research assistants provide support to professionals who are conducting experiments or gathering andanalyzing information and data. Suppose you are working as RA (research assistant) with a Professor who isworking on a project and evaluating complex mathematical equations. Your duty is to assist him, so in thisregard he has assigned a task to you. Your task is to write a oop c++ program to create a class named equation which will have the data members a, b and c which are the coefficients of the quadratic equation. The class will have two more data members namely proot and nroot which stand for the positive root and negative root of the equation. Suppose that variables a, b and c are integers. Where proot and nroot are floats. Input Function to get values of a, b and c Then design a friend function which will determine the proot and nroot of the equation. Create another friend function which will display the values of proot and nroot.arrow_forwardC) Write a Class Result that contains Name, Roll Number and Marks of five subjects. The marks are stored in an array of integers. The class contains the following member functions: input(), which is used to input the values in data members. show(), which is used to displays the values of data members. total(), which returns the total marks of a student. average(), which returns the average marks of a student. Define all member functions outside the class.arrow_forwardC++(OOP)Write a program that implements the following situation.A marketing organization ABC Ltd helps to promote the sales of other brands and products. ABCLtd has permanent employees and also hires employees from outside as well for different occasionson contract basis. A permanent employee gets monthly salary whereas contractual employee getspaid on daily wage rate along with basic salary.You need to create a class Employee with following data members.• String name• Int age• Int contact• Int CNIC• String educationYou will inherit the Employee class into Permanent_EMP and Contractual_EMP classes.In Permanent_EMP you are required to create following data members• Int basic_salary• Int allowancesIn addition, you are required to write down following class functions• A constructor that takes values of salary & allowances at the time of object declaration.• A method that calculates and returns the salary with following formulao Salary = basic_salary + allowances.In Contractual_EMP…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
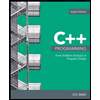
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning