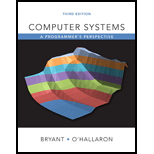
Computer Systems: A Programmer's Perspective (3rd Edition)
3rd Edition
ISBN: 9780134092669
Author: Bryant, Randal E. Bryant, David R. O'Hallaron, David R., Randal E.; O'Hallaron, Bryant/O'hallaron
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 4, Problem 4.45HW
A.
Program Plan Intro
Given Assembly code:
subq $8, %rsp
movq REG, (%rsp)
Data movement instructions:
- The different instructions are been grouped as “instruction classes”.
- The instructions in a class performs same operation but with different sizes of operand.
- The “Mov” class denotes data movement instructions that copy data from a source location to a destination.
- The class has 4 instructions that includes:
- movb:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 1 byte data size.
- movw:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 2 bytes data size.
- movl:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 4 bytes data size.
- movq:
- It copies data from a source location to a destination.
- It denotes an instruction that operates on 8 bytes data size.
- movb:
Unary and Binary Operations:
- The details of unary operations includes:
- The single operand functions as both source as well as destination.
- It can either be a memory location or a register.
- The instruction “incq” causes 8 byte element on stack top to be incremented.
- The instruction “decq” causes 8 byte element on stack top to be decremented.
- The details of binary operations includes:
- The first operand denotes the source.
- The second operand works as both source as well as destination.
- The first operand can either be an immediate value, memory location or register.
- The second operand can either be a register or a memory location.
Jump Instruction:
- The “jump” instruction causes execution to switch to an entirely new position in program.
- The “label” indicates jump destinations in assembly code.
- The “je” instruction denotes “jump if equal” or “jump if zero”.
- The comparison operation is performed.
- If result of comparison is either equal or zero, then jump operation takes place.
- The “ja” instruction denotes “jump if above”.
- The comparison operation is performed.
- If result of comparison is greater, then jump operation takes place.
- The “pop” instruction resumes execution of jump instruction.
- The “jmpq” instruction jumps to given address. It denotes a direct jump.
A.
Expert Solution

Explanation of Solution
Behavior of instruction pushq:
- No, the given code sequence is not correctly describes the behavior of given instruction.
- The instruction “subq $8, %rsp” subtracts 8 from value of “%rsp”.
- If “REG” denotes “%rsp” then it computes “%rsp-8”.
- The instruction “movq REG, (%rsp)” moves value of “REG” into location of “(%rsp)”.
- The value “%rsp-8” is been pushed into stack instead of “%rsp”.
- Hence, it does not describe behavior of instruction “pushq %rsp”.
B.
Program Plan Intro
Given Assembly code:
subq $8, %rsp
movq REG, (%rsp)
Processing stages:
- The processing of an instruction has number of operations.
- The operations are organized into particular sequence of stages.
- It attempts to follow a uniform sequence for all instructions.
- The description of stages are shown below:
- Fetch:
- It uses program counter “PC” as memory address to read instruction bytes from memory.
- The 4-bit portions “icode” and “ifun” of specifier byte is extracted from instruction.
- It fetches “valC” that denotes an 8-byte constant.
- It computes “valP” that denotes value of “PC” plus length of fetched instruction.
- Decode:
- The register file is been read with two operands.
- It gives values “valA” and “valB” for operands.
- It reads registers with instruction fields “rA” and “rB”.
- Execute:
- In this stage the ALU either performs required operation or increments and decrements stack pointer.
- The resulting value is termed as “valE”.
- The condition codes are evaluated and destination register is updated based on condition.
- It determines whether branch should be taken or not in a jump instruction.
- Memory:
- The data is been written to memory or read from memory in this stage.
- The value that is read is determined as “valM”.
- Write back:
- The results are been written to register file.
- It can write up to two results.
- PC update:
- The program counter “PC” denotes memory address to read bytes of instruction from memory.
- It is used to set next instruction’s address.
- Fetch:
B.
Expert Solution

Explanation of Solution
Modified code:
movq REG, -8(%rsp)
subq $8, %rsp
Explanation:
- The instruction “movq REG, -8(%rsp)” moves value of “REG” into location of “(%rsp)-8”.
- The instruction “subq $8, %rsp” subtracts 8 from value of “%rsp”.
- If “REG” denotes “%rsp” then it computes “%rsp”.
- The value “%rsp” is now been pushed into stack.
- Hence, it describes behavior of instruction “pushq %rsp”.
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Students have asked these similar questions
I want to solve 13.2 using matlab please help
a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.
using r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.
Chapter 4 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Ch. 4.1 - Prob. 4.1PPCh. 4.1 - Prob. 4.2PPCh. 4.1 - Prob. 4.3PPCh. 4.1 - Prob. 4.4PPCh. 4.1 - Prob. 4.5PPCh. 4.1 - Prob. 4.6PPCh. 4.1 - Prob. 4.7PPCh. 4.1 - Prob. 4.8PPCh. 4.2 - Practice Problem 4.9 (solution page 484) Write an...Ch. 4.2 - Prob. 4.10PP
Ch. 4.2 - Prob. 4.11PPCh. 4.2 - Prob. 4.12PPCh. 4.3 - Prob. 4.13PPCh. 4.3 - Prob. 4.14PPCh. 4.3 - Prob. 4.15PPCh. 4.3 - Prob. 4.16PPCh. 4.3 - Prob. 4.17PPCh. 4.3 - Prob. 4.18PPCh. 4.3 - Prob. 4.19PPCh. 4.3 - Prob. 4.20PPCh. 4.3 - Prob. 4.21PPCh. 4.3 - Prob. 4.22PPCh. 4.3 - Prob. 4.23PPCh. 4.3 - Prob. 4.24PPCh. 4.3 - Prob. 4.25PPCh. 4.3 - Prob. 4.26PPCh. 4.3 - Prob. 4.27PPCh. 4.4 - Prob. 4.28PPCh. 4.4 - Prob. 4.29PPCh. 4.5 - Prob. 4.30PPCh. 4.5 - Prob. 4.31PPCh. 4.5 - Prob. 4.32PPCh. 4.5 - Prob. 4.33PPCh. 4.5 - Prob. 4.34PPCh. 4.5 - Prob. 4.35PPCh. 4.5 - Prob. 4.36PPCh. 4.5 - Prob. 4.37PPCh. 4.5 - Prob. 4.38PPCh. 4.5 - Prob. 4.39PPCh. 4.5 - Prob. 4.40PPCh. 4.5 - Prob. 4.41PPCh. 4.5 - Prob. 4.42PPCh. 4.5 - Prob. 4.43PPCh. 4.5 - Prob. 4.44PPCh. 4 - Prob. 4.45HWCh. 4 - Prob. 4.46HWCh. 4 - Prob. 4.47HWCh. 4 - Prob. 4.48HWCh. 4 - Modify the code you wrote for Problem 4.47 to...Ch. 4 - In Section 3.6.8, we saw that a common way to...Ch. 4 - Prob. 4.51HWCh. 4 - The file seq-full.hcl contains the HCL description...Ch. 4 - Prob. 4.53HWCh. 4 - The file pie=full. hcl contains a copy of the PIPE...Ch. 4 - Prob. 4.55HWCh. 4 - Prob. 4.56HWCh. 4 - Prob. 4.57HWCh. 4 - Our pipelined design is a bit unrealistic in that...Ch. 4 - Prob. 4.59HW
Knowledge Booster
Similar questions
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- Principles of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
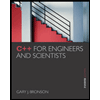
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
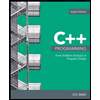
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
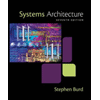
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
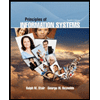
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
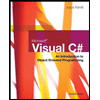
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
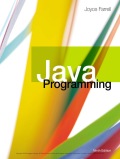
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT