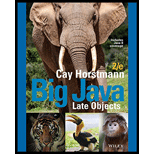
Given Program:
The program given in the textbook is given here with comments for better understanding.
File Name: TaxReturn.java
// Class definition
public class TaxReturn
{
// Declare and initialize the required variables
public static final int SINGLE = 1;
public static final int MARRIED = 2;
private static final double RATE1 = 0.10;
private static final double RATE2 = 0.25;
private static final double RATE1_SINGLE_LIMIT = 32000;
private static final double RATE1_MARRIED_LIMIT = 64000;
private double income;
private int status;
/*Constructs a TaxReturn object for a given income and marital status.
@param anIncome the taxpayer income
@param aStatus either SINGLE or MARRIED */
// Method definition
public TaxReturn(double anIncome, int aStatus)
{
income = anIncome;
status = aStatus;
}
// Method definition
public double getTax()
{
// Declare and initialize the required variables
double tax1 = 0;
double tax2 = 0;
/* If the entered status is "Single", compute income tax based on their income */
if (status == SINGLE)
{
/* Check whether the income is less than or equal to $32000 */
if (income <= RATE1_SINGLE_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $32000, compute the tax */
else
{
tax1 = RATE1 * RATE1_SINGLE_LIMIT;
tax2 = RATE2 * (income - RATE1_SINGLE_LIMIT);
}
}
/* If the entered status is "Married", compute income tax based on their income */
else
{
/* Check whether the income is less than or equal to $64000 */
if (income <= RATE1_MARRIED_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $64000, compute the tax */
else
{
tax1 = RATE1 * RATE1_MARRIED_LIMIT;
tax2 = RATE2 * (income - RATE1_MARRIED_LIMIT);
}
}
// Return the tax to the main function
return tax1 + tax2;
}
}
File Name: TaxCalculator.java
// Import the required package
import java.util.Scanner;
/**
This program calculates a simple tax return.
*/
// Class definition
public class TaxCalculator
{
// Main class declaration
public static void main(String[] args)
{
// Create an object for scanner class
Scanner in = new Scanner(System.in);
// Prompt the user to enter the income
System.out.print("Please enter your income: ");
// Store the entered income in the variable
double income = in.nextDouble();
// Prompt the user to enter marital status
System.out.print("Are you married? (Y/N) ");
// Store the entered value in a variable
String input = in.next();
// Declare the variable
int status;
/* Check whether the user input value for marital status is "Y" */
if (input.equals("Y"))
{
/* If it is "Y", store the taxreturn value for a married person in the variable */
status = TaxReturn.MARRIED;
}
/* Check whether the user input value for marital status is "N" */
else
{
/* If it is "N", store the taxreturn value for a single person in the variable */
status = TaxReturn.SINGLE;
}
// Create an object for TaxReturn class
TaxReturn aTaxReturn = new TaxReturn(income, status);
// Display the tax return value based on the user input
System.out.println("Tax: "
+ aTaxReturn.getTax());
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Big Java Late Objects
- Any help with this question would really help!! Write the following exercise using Java code. Add comments in the program to explain what your code is doing. Duke Shirts sells Java t-shirts for $24.95 each, but discounts are possible for quantities as follows: 1 or 2 shirts, no discount and total shipping is $10.003-5 shirts, discount is 10% and total shipping is $8.006-10 shirts, discount is 20% and total shipping is $5.0011 or more shirts, discount is 30% and shipping is free Write a Java program that prompts the user for the number of shirts required. The program should then print the extended price of the shirts, the shipping charges, and the total cost of the order. Use currency format where appropriate.arrow_forwardCan you help me with this program?In C++ The room contains n*n cubes (in rows and columns). Each cube is white or black. You can get out of a white cube by going in one of the four directions horizontally or vertically (but you can't go beyond the scope of the room). You can get out in a black cube, but you can't get out of it. There is a mouse and a piece of cheese in the room. The mouse is in a cube (0,0) - white, and the cheese in a cube (n-1, n-1) - black. The mouse can move from cube to cube according to the rules described above. Write a program that finds all the paths that can be moved to the cheese with the mouse and if it appears, display it with ‘*’. An example diagram of the task is shown in the figure: Operating instructions: Compose a recursive function path (int i, int j), receiving as parameters the coordinates of the current position of the mouse (initially 0.0, and then run with parameters (i-1, j), (i + 1, j), ( i, j-1) or (i, j + 1)).arrow_forwardI'm having trouble with this Java assignment.arrow_forward
- Use JAVA to create a card game in which the dealer shuffles the deck and deals 13 cards out of the 52 to the player. The player sorts her hand and says whether it includes the King of Hearts. Repeat this process 4 times so that all the 52 cards are dealt. The player should return true or false without writing to the console. In response, the dealer should write on the console, "You won!" or "You loose again." Also create a unit test for the win and loose cases. Create an orignal code with Java card graphics. Please do not reshare a similar answer!arrow_forwarde Please read the whole document. Show the output screenshots with the code when done. You have to write it in Java Try and use methods and functions in the code.arrow_forwardHi, need help with the ques in Java programing, and will need the pseudo code as well, thanks in advanced.arrow_forward
- 4) For the following problem, write a program (in as close to Java code -but doesn’t need to be compilable/runnable code, ie no English explanation “vague” pseudocode and all helper methods must be defined). A few years ago, 2048 was a popular game to download and play on smartphone. You don't need to know anything about 2048, but here is a different game called 248. In 248, the game starts with a sequence of N integers where N is between 2 and 248 (inclusive) and each integer in the sequence is between 1 and 40 (inclusive). To play the game, the player must select two adjacent integers with equal values and replace them with a single integer of value one greater. For example, two adjacent 3's can be replaced with 4. After repeatedly doing combination operations there will eventually be no more equal adjacent numbers in the sequence and the game is then done. The score at the end of a game is whatever number is largest in the final sequence. Write a program to find the largest…arrow_forwardIn JAVA ASSIGNMENT DESCRIPTION: A half-life is the amount of time it takes for a substance or entity to fall to half its original value. Caffeine has a half-life of about 6 hours in humans. Given caffeine amount (in mg) as input, output the caffeine level after 6, 12, and 24 hours. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:System.out.printf("After 6 hours: %.2f mg\n", yourValue); Ex: If the input is: 100 the output is: After 6 hours: 50.00 mg After 12 hours: 25.00 mg After 24 hours: 6.25 mg Note: A cup of coffee has about 100 mg. A soda has about 40 mg. An "energy" drink (a misnomer) has between 100 mg and 200 mg. THE CODE I HAVE SO FAR: import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); double caffeineMg; // "double" supports floating-point like 75.5, versus int for integers like 75. caffeineMg = scnr.nextDouble(); System.out.printf("After…arrow_forwardNeed help with my intro to java assignment, would appreiciate it if the code is in simply terms. Thanks in advance, the oil txt is below.arrow_forward
- I need the answer quickly please. We need to use java programarrow_forward**This is programming with Java. Im a bit confused.. When answering if you could be kind enough to explain as well** Input When your program runs, it should have a dialog with the user. Ask the user: 1) the x and y location of the center of each asteroid as well as its size (allow the user to decide how many asteroids), 2) the current score, 3) the number of lives remaining, 4)the x and y location of the player’s spaceship, 5) the x and y location of at least 1 alien spaceship, and 6)the color of the player’s spaceship and the alien spaceship (use integers, e.g. 1=red, 2=blue, 3=green). Make sure the user enters values that will look good (e.g. each asteroid should display fully on the screen). Give the user a range of acceptable values in each prompt and make them repeat each input until they get it right. Important Tip: Instead of storing the asteroid locations and sizes, draw each asteroid as soon as the user has entered its x, y, and size values. Output When the stage appears, it…arrow_forwardFor the following problem, write a program (in as close to Java code - but doesn't need to be compilable/runnable code, ie no English explanation "vague" pseudocode and all helper methods must be defined) 248 A few years ago, 2048 was a popular game to download and play on the smartphone. You don't need to know anything about 2048, but here is a different game called 248. In 248, the game starts with a sequence of N integers where N is between 2 and 248 (inclusive) and each integer in the sequence is between 1 and 40 (inclusive). To play the game, the player must select two adjacent integers with equal values and replace them with a single integer of value one greater. For example, two adjacent 3's can be replaced with a 4. After repeatedly doing combination operations there will eventually be no more equal adjacent numbers in the sequence and the game is then done. The score at the end of a game is whatever number is largest in the final sequence. Write a program to find the largest…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
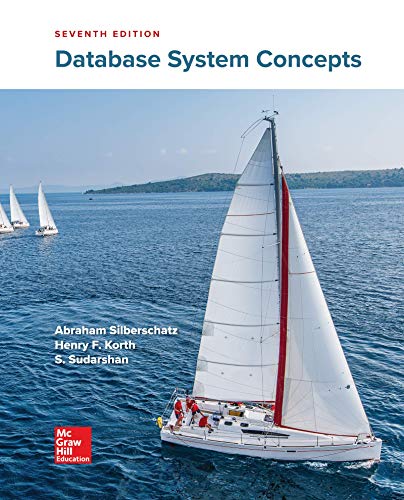
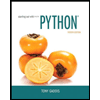
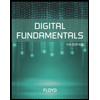
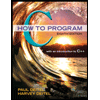
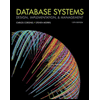
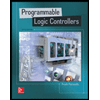