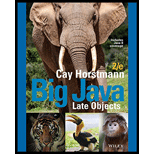
Concept explainers
Given Program:
The program given in the textbook is given here with comments for better understanding.
File Name: TaxReturn.java
// Class definition
public class TaxReturn
{
// Declare and initialize the required variables
public static final int SINGLE = 1;
public static final int MARRIED = 2;
private static final double RATE1 = 0.10;
private static final double RATE2 = 0.25;
private static final double RATE1_SINGLE_LIMIT = 32000;
private static final double RATE1_MARRIED_LIMIT = 64000;
private double income;
private int status;
/*Constructs a TaxReturn object for a given income and marital status.
@param anIncome the taxpayer income
@param aStatus either SINGLE or MARRIED */
// Method definition
public TaxReturn(double anIncome, int aStatus)
{
income = anIncome;
status = aStatus;
}
// Method definition
public double getTax()
{
// Declare and initialize the required variables
double tax1 = 0;
double tax2 = 0;
/* If the entered status is "Single", compute income tax based on their income */
if (status == SINGLE)
{
/* Check whether the income is less than or equal to $32000 */
if (income <= RATE1_SINGLE_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $32000, compute the tax */
else
{
tax1 = RATE1 * RATE1_SINGLE_LIMIT;
tax2 = RATE2 * (income - RATE1_SINGLE_LIMIT);
}
}
/* If the entered status is "Married", compute income tax based on their income */
else
{
/* Check whether the income is less than or equal to $64000 */
if (income <= RATE1_MARRIED_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $64000, compute the tax */
else
{
tax1 = RATE1 * RATE1_MARRIED_LIMIT;
tax2 = RATE2 * (income - RATE1_MARRIED_LIMIT);
}
}
// Return the tax to the main function
return tax1 + tax2;
}
}
File Name: TaxCalculator.java
// Import the required package
import java.util.Scanner;
/**
This program calculates a simple tax return.
*/
// Class definition
public class TaxCalculator
{
// Main class declaration
public static void main(String[] args)
{
// Create an object for scanner class
Scanner in = new Scanner(System.in);
// Prompt the user to enter the income
System.out.print("Please enter your income: ");
// Store the entered income in the variable
double income = in.nextDouble();
// Prompt the user to enter marital status
System.out.print("Are you married? (Y/N) ");
// Store the entered value in a variable
String input = in.next();
// Declare the variable
int status;
/* Check whether the user input value for marital status is "Y" */
if (input.equals("Y"))
{
/* If it is "Y", store the taxreturn value for a married person in the variable */
status = TaxReturn.MARRIED;
}
/* Check whether the user input value for marital status is "N" */
else
{
/* If it is "N", store the taxreturn value for a single person in the variable */
status = TaxReturn.SINGLE;
}
// Create an object for TaxReturn class
TaxReturn aTaxReturn = new TaxReturn(income, status);
// Display the tax return value based on the user input
System.out.println("Tax: "
+ aTaxReturn.getTax());
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Big Java Late Objects
- Finance do not use excel calculation sreen shotarrow_forwardThe number of different values that can be expressed using a single digit Your answerarrow_forwardin a shop ,there are 10 employee and 20 kinds of goods,goods id between 1-20 EMPLOYEE id first name last name gender 10001, 'Tom', 'Brown', 'F'10002, 'Elizabeth', 'Tremblay', 'F'10003, 'Gladys', 'Julie', 'F10004, 'John', 'Taylor', 'M10005, 'Amelia', 'Smith'10006, 'Logan', 'Katherine'10007, 'Leo', 'Brown'10007, 'Lem', 'Thompson'10009, 'Tom' 'Smith'10010, 'Emma', 'Campbell' ------------ and I want to add a name library in it ,like this how could i create a HTML file ,with will randomly create customers with these employee . there is a start button on the page . press "start "bottom ,and it will It will randomly match 10 items, customers, and goods, display goods id ,customername and gender ,employer name , id and gender . how to do such a page ?arrow_forward
- The following table shows the rate of commission that will be paid to the salesman according to the total sales performance of the month:Total Sales Performance (RM) CommissionLess than 10000 No commission10000 – 20000 1% of the total sales20001 – 30000 2% of the total sales30001 – 40000 3% of the total salesMore than 40000 4% of the total sales Lastly, display the salesmanName, totalSalesPerformance,totalCommission and totalSalary.arrow_forwardWhat is MINR minimum value across a range of value?arrow_forwardComputer sciencearrow_forward
- Section: FactThyroid function is measured using a factor called TSH, which stands for thyroid-stimulatinghormone. A TSH test is a blood test that measures this hormone. TSH normal values are 0.5 to 5.0mIU/L. If TSH is over 5.0 thyroid is underactive. On the other hand, if TSH is less than 0.5 thyroid isoveractive.arrow_forwardFind your group number• The remainder after division of second to last digit of your student id by 3 is a.• The last digit of your student id is b.• Your group number is ab, which is an integer between 0 and 29.• If your group number is equal to ab=00 then take it as ab=01.Examples:• Student Id = 290316027 → Group = 27• Student Id = 290315043 → Group = 13• Student Id = 280315061 → Group = 01• Student Id = 270316085 → Group = 25• Student Id = 290315027 → Group = 27 my student Id is 280316014 but i didnt calculate ab.Can you help me .Thank you.arrow_forwardRequirements List the needed requirements to estimate a population proportion List the needed requirements to estimate a population meanarrow_forward
- COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
- A Guide to SQLComputer ScienceISBN:9781111527273Author:Philip J. PrattPublisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks Cole
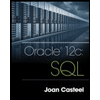
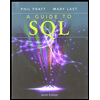
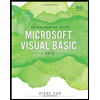
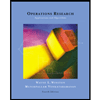