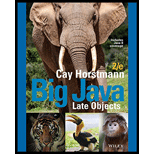
Given Program:
The program given in the textbook is given here with comments for better understanding.
File Name: TaxReturn.java
// Class definition
public class TaxReturn
{
// Declare and initialize the required variables
public static final int SINGLE = 1;
public static final int MARRIED = 2;
private static final double RATE1 = 0.10;
private static final double RATE2 = 0.25;
private static final double RATE1_SINGLE_LIMIT = 32000;
private static final double RATE1_MARRIED_LIMIT = 64000;
private double income;
private int status;
/*Constructs a TaxReturn object for a given income and marital status.
@param anIncome the taxpayer income
@param aStatus either SINGLE or MARRIED */
// Method definition
public TaxReturn(double anIncome, int aStatus)
{
income = anIncome;
status = aStatus;
}
// Method definition
public double getTax()
{
// Declare and initialize the required variables
double tax1 = 0;
double tax2 = 0;
/* If the entered status is "Single", compute income tax based on their income */
if (status == SINGLE)
{
/* Check whether the income is less than or equal to $32000 */
if (income <= RATE1_SINGLE_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $32000, compute the tax */
else
{
tax1 = RATE1 * RATE1_SINGLE_LIMIT;
tax2 = RATE2 * (income - RATE1_SINGLE_LIMIT);
}
}
/* If the entered status is "Married", compute income tax based on their income */
else
{
/* Check whether the income is less than or equal to $64000 */
if (income <= RATE1_MARRIED_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $64000, compute the tax */
else
{
tax1 = RATE1 * RATE1_MARRIED_LIMIT;
tax2 = RATE2 * (income - RATE1_MARRIED_LIMIT);
}
}
// Return the tax to the main function
return tax1 + tax2;
}
}
File Name: TaxCalculator.java
// Import the required package
import java.util.Scanner;
/**
This program calculates a simple tax return.
*/
// Class definition
public class TaxCalculator
{
// Main class declaration
public static void main(String[] args)
{
// Create an object for scanner class
Scanner in = new Scanner(System.in);
// Prompt the user to enter the income
System.out.print("Please enter your income: ");
// Store the entered income in the variable
double income = in.nextDouble();
// Prompt the user to enter marital status
System.out.print("Are you married? (Y/N) ");
// Store the entered value in a variable
String input = in.next();
// Declare the variable
int status;
/* Check whether the user input value for marital status is "Y" */
if (input.equals("Y"))
{
/* If it is "Y", store the taxreturn value for a married person in the variable */
status = TaxReturn.MARRIED;
}
/* Check whether the user input value for marital status is "N" */
else
{
/* If it is "N", store the taxreturn value for a single person in the variable */
status = TaxReturn.SINGLE;
}
// Create an object for TaxReturn class
TaxReturn aTaxReturn = new TaxReturn(income, status);
// Display the tax return value based on the user input
System.out.println("Tax: "
+ aTaxReturn.getTax());
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Big Java Late Objects
- Mr. and Mrs. John Smith have signed a purchase agreement for a 4 bedroom house with separate garage. The purchase price is $500,000. They plan on putting a down payment of 20 percent. They are with their lender who shows the couple how much their monthly mortgage payment would be if they took out a 30-year mortgage, a 20-year mortgage, or if they took out a 15-year mortgage. The interest rate is 2.5% to take out the loan. Write a JAVA program that will help the Smiths and any other customer of the lender determine their monthly mortgage payment based on the following input: - Purchase Price - Down Payment - Loan Amount (purchase price - down payment) - Interest rate in decimal form - Number of years for mortgage The program will also include entry of the name(s) for the mortgagee (person taking out the loan for the mortgage) and the date the calculations were made. The program will also ask if the client wants to run the program again [that way the Smiths' will be able to…arrow_forwardYou are in the process of getting a new car but are not sure if you should buy or lease. The price of the car you want is $18,000, but you do not want to spend more than $250 a month on car payments. If you lease the car, the terms of the lease will be 48 months at an annual interest rate of 5%. The residual value of the car will be set at $9,000. If you buy the car, your bank will offer you a 7-year loan at an annual interest rate of 6%. You are not required to make a down payment with either the lease or loan options, and payments are made at the end of the month for both options. Instructions Should you lease or buy the car given your budget limit of $250 a month? Create a new workbook and design a worksheet that shows the difference between leasing and buying the car in terms of monthly payments. Use proper formatting so your worksheet is easy to read. Remember to use column and row headings, add a title to your worksheet, and rename the worksheet tab with an appropriate label.…arrow_forwardA farmer in Georgia has a 100-acre farm on which to plant watermelons and cantaloupes. Every acre planted with watermelons requires 50 gallons of water per day and must be prepared for planting with 20 pounds of fertilizer. Every acre planted with cantaloupes requires 75 gallons of water per day and must be prepared for planting with 15 pounds of fertilizer. The farmer estimates that it will take 2 hours of labor to harvest each acre planted with watermelons and 2.5 hours to harvest each acre planted with cantaloupes. He believes that watermelons will sell for about $3 each, and cantaloupes will sell for about $1 each. Every acre planted with watermelons is expected to yield 90 salable units. Every acre planted with cantaloupes is expected to yield 300 salable units. The farmer can pump about 6,000 gallons of water per day for irrigation purposes from a shallow well. He can buy as much fertilizer as he needs at a cost of $10 per 50-pound bag. Finally, the farmer can hire laborers to…arrow_forward
- Here I am again with my special Omicron Juice! Here goes the story: I have three kids- Alpha, Beta and Gamma. Every morning I need to prepare them for school, serve them breakfast and say them bye. You might think that I don't like all these. But to be honest I do like it, specially I have fun serving them breakfast. Every morning I serve them Omicron Juice. But I can't always pour the juice in equal amounts. If they don't get equal amounts of juice then they start fighting. I use two other glasses to make the juice amount equal. To be more specific... There are three glasses with juice of amount A, B and C. I have two other glasses which can hold exactly K amount of drinks each and initially empty. Let's call these two extra glasses as cups to avoid ambiguity. In one operation, I pour K amount of drinks from one of the three glasses to my first cup, and from one of the two remaining glasses to my second cup. Next I pour the drink from my first cup to one of the three glasses and from…arrow_forwardSuppose you have to select one project partner from a set of four classmates, who have different GPAs. Assume you do not know any student’s GPA in advance but can get to know it after you have picked a student from that group (a) Suppose you pick one of the four students at random and accept that student as your project partner. What is the probability that your partner is the one with the highest GPA? (b) Suppose you decide to reject the first student and to then accept the next student if and only if that student has a higher GPA. Note that you MUST have a partner, so if the first three are rejected by you, then you have to accept the fourth student. What is the probability that your partner will be the one with the highest GPA.arrow_forwardYou found an exciting summer job for five weeks. It pays, say, $15.50 per hour. Suppose thatthe total tax you pay on your summer job income is 14%. After paying the taxes, you spend10% of your net income to buy new clothes and other accessories for the next school yearand 1% to buy school supplies. After buying clothes and school supplies, you use 25% of theremaining money to buy savings bonds. For each dollar you spend to buy savings bonds,your parents spend $0.50 to buy additional savings bonds for you. Write a flowchart that prompts the user to enter the number of hours you worked each week. The program thenoutputs the following:a. Your income before and after taxes from your summer job.b. The money you spend on clothes and other accessories.c. The money you spend on school supplies.d. The money you spend to buy savings bonds.e. The money your parents spend to buy additional savings bonds for you.arrow_forward
- What is the simple interest of an investment amounting to P 10,000.00, if it yields 5% per year within 10 years?arrow_forwardDo you agree with the assumption that the technologies of the Fourth Industrial Revolution will eventually take over practically all of the world's jobs?arrow_forwardHow long till self-driving cars?arrow_forward
- Operations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
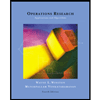