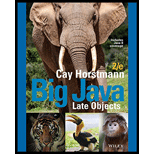
Concept explainers
Explanation of Solution
Program:
The earthquake program is as follows:
File name: “Earth.java”
//Create a class
public class Earth
{
//Declare the variable
private double mag;
//Define the constructor
public Earth(double magnit)
{
//Assign the magnitude value
mag = magnit;
}
//Define a method "getDesc()"
public String getDesc()
{
//Declare the variable for magnitude description
String desc;
/*Check whether the value of magnitude is greater than or equal to "8.0" */
if (mag >= 8.0)
{
//Assign the description
desc = "Most structures fall";
}
/*Check whether the value of magnitude is greater than or equal to "7.0" */
else if (mag >= 7.0)
{
//Assign the description
desc = "Many buildings destroyed";
}
/*Check whether the value of magnitude is greater than or equal to "6.0" */
else if (mag >= 6.0)
{
//Assign the description
desc = "Many buildings considerably damaged, some collapse";
}
/*Check whether the value of magnitude is greater than or equal to "4.5" */
else if (mag >= 4...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Big Java Late Objects
- Write a program that reads from the user a character (*q' or 'c'). If the character is 'c', the program reads from the user the radius r of a circle and prints its area. If the user enters 'q', the program asks the user to enter the length and width of a quadrilateral. We assume the quadrilateral is either a square or rectangle. You should print if the quadrilateral is square or rectangle. Otherwise, it prints "Wrong character" PS: Use the following formulas : area of circle=3.14*r? Iarrow_forwardAnswer in Java pleasearrow_forwardWelcome to the era of Aladdin and the great magic lamp. This is 1st of January and Aladdin will be in jail for one year. He was recently caught for helping forty thieves. Now he has nothing to do. But he knows that Jasmine is waiting for him. At some point he got an idea to give Jasmine a magical program that can find intervals of months from a year. This allows her to easily determine how long it will take for Aladdin to get out. Then Aladdin rubs the magic lamp and The Genie appears. Then he asked The Genie to make such a magical program. Unfortunately the Genie doesn't know programming and he wants your help to create that magical program. Genie will give you N as the day of the year and you have to print in which month that day is. Input Format Input will contain one line with a single integer N, day of the year. Constraints 1 <= N <= 365 Output Format Print a single line containing the name of month. Be careful about the newline ('\n') at the end. Sample Input 0 1 Sample…arrow_forward
- Write a program that simulates a simple calculator. It reads two integers and a character. If the character is a ‘+’, the sum is printed; if it is a ‘-’, the difference is printed; if it is a ‘*’, the product is printed; if it is a ‘/’, the quotient is printed; and if it is a ‘%’, the remainder is printed. (Refrance C++)arrow_forwardWrite a program that invites the user to enter two numbers: a dividend (the top number in a division) and a divisor (the bottom number). It then calculates quotient and remainder using / and % operator and displays the result. After one iteration it asks user whether he/she wants to do more (Y/N). (C++ show input and output as well)arrow_forwardWrite a program that reads the volume, V, as a floating-point number. It then computes and prints the radius of a sphere having volume V, and then computes and prints the height of a cylinder having the same radius and the same volume V. You may use math library functions if necessary.arrow_forward
- Must be in JAVA. Please show in simplest form and with comments.arrow_forwardTask 9 Write Python code of a program that reads an integer, and prints the integer if it is a multiple of NEITHER 2 NOR 5. For example, 1, 3, 7, 9, 11, 13, 17, 19, 21, 23, 27, 29, 31, 33, 37, 39 ... hint(1): use the modulus (%) operator for checking the divisibility hint(2): You can consider the number to be an integer !%3%===== Example01: Input: 3 Output: 3arrow_forwardCreate a program which transforms the number of days to years, weeks and days. For example: 375 days = 1 year 1 week 3 days. Use the remainder of the bigger unit. ( in C language)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
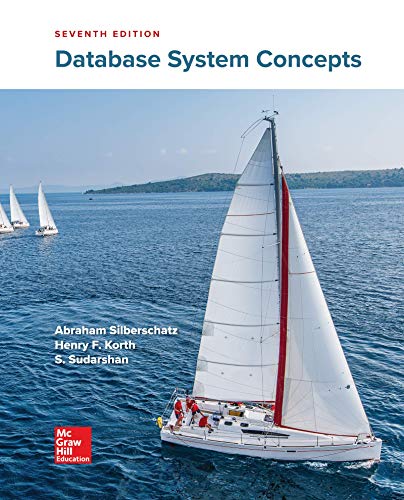
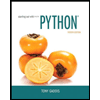
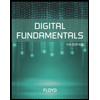
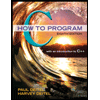
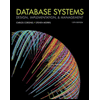
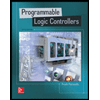