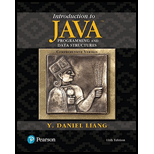
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 28, Problem 28.19PE
Program Plan Intro
Display a DFS/BFS tree in a graph
Program Plan:
Exercise.java:
- Import the required packages.
- Create a class “Exercise”:
- Create a text field.
- Create the required buttons.
- Create the label.
- City values vertices gets assigned.
- City value edges get assigned.
- New graph gets created.
- New view gets defined
- Define the start method:
- Create a new border pane.
- New hbox gets created.
- Add the buttons into the pane.
- Set the alignment of the pane.
- New scene gets created
- Set the title of the scene.
- Scene gets placed on the stage.
- The stage gets displayed.
- Action event for the button defined is set.
- Based on the action defined a new tree is defined.
- Define the main method
- Initialize the calls.
- Class “GraphView” gets defined
- Assign the graph.
- Assign the tree
- Define the method “GraphView”
- Assign the graph.
- Define the method “setTree”
- Assign the tree
- Define the method “repaint()”
- Clear the pane.
- Loop that iterates to draw the vertices
- Get the position of x and y coordinates.
- Condition to validate the edge gets defined.
- Loop that iterates to draw the line.
- Define the method “drawArrowLine”
- New lines get created.
- Add the line color.
- Calculate the angle.
- Set the angle.
- Condition to validate the arrow point gets defined.
- Draw the arrow at the required positions.
- Define the class “city”
- Declare the required variables.
- Assign the x and y value.
- Get the name.
- Validate the city name that is entered.
UnweightedGraph.java:
- Import the required packages.
- Create a class “UnweightedGraph”:
- New list for the vertices gets created.
- New list for the neighbor node gets created.
- Create an empty constructor.
- Method to create new graph gets created and adjacency list gets created.
- Method to create an adjacency list gets created.
- Method to return the size of the vertices.
- Method to return the index of the vertices gets defined.
- Method to gets the neighbor node gets defined.
- Method to return the degree of the vertices gets created.
- Method to print the Edges gets created.
- New to clear the graph gets created.
- Method to add vertex gets created.
- Method to add edge gets created.
- Method to perform the depth first search gets defined.
- Method to perform breadth first search gets defined.
- Search tree gets returned.
- Create a class “SearchTree”
- Define the method to return the root.
- Method to return the parent of the vertices
- Method to return the search order gets defined.
- Method to return the number of vertices found gets defined.
- Method to get the path of the vertices gets defined.
- Loop to validate the path gets defined.
- Path gets returned.
- Method to print the path gets defined.
- Method to print the tree gets defined.
- Display the edge.
- Display the root.
- Condition to validate the parent node to display the vertices gets created.
Graph.java:
- A graph interface gets created.
- Method to return the size gets defined.
- Method to return the vertices gets defined.
- Method to return the index gets created.
- Method to get the neighbor node gets created.
- Method to get the degree gets created.
- Method to print the edges.
- Method to clear the node gets created.
- Method to add the edges, add vertex gets created.
- Method to remove the vertices gets defined.
- Method for the depth first search gets defined.
- Method for the breadth first search gets defined.
Displayable.java
- Define the interface.
- Get the x value
- Get the y value.
- Get the name.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In C++, write a program that outputs the nodes of a graph in a breadth first traversal.
Data File: Please use this data file.
Text to copy:
100 1 3 -9991 4 -9992 5 -9993 2 -9994 -9995 7 8 -9996 4 7 -9997 -9998 -9999 7 8 -999
Diagram: Also, please take a look at the attached figure on and calculate the weights for the following edges:
0 -> 1 -> 4
0 -> 3 -> 2 -> 5 -> 7
0 -> 3 -> 2 -> 5 -> 8
6 -> 4
6 -> 7
9 -> 7
9 -> 8
To calculates these weights, please assume the following data:
0 -> 1 = 1
0 -> 3 = 2
1 -> 4 = 3
3 -> 2 = 4
2 -> 5 = 5
5 -> 7 = 6
5 -> 8 = 7
6 -> 4 = 8
6 -> 7 = 9
9 -> 7 = 10
9 -> 8 = 11
Programming language: Java
Topic: linked list
In C++, write a program that outputs the nodes of a graph in a breadth first traversal.
Data File: Please use this data file.
Text to copy:
100 1 3 -9991 4 -9992 5 -9993 2 -9994 -9995 7 8 -9996 4 7 -9997 -9998 -9999 7 8 -999
Diagram: Also, please take a look at figure 20-6 on page 1414 and calculate the weights for the following edges:
0 -> 1 -> 4
0 -> 3 -> 2 -> 5 -> 7
0 -> 3 -> 2 -> 5 -> 8
6 -> 4
6 -> 7
9 -> 7
9 -> 8
To calculates these weights, please assume the following data:
0 -> 1 = 1
0 -> 3 = 2
1 -> 4 = 3
3 -> 2 = 4
2 -> 5 = 5
5 -> 7 = 6
5 -> 8 = 7
6 -> 4 = 8
6 -> 7 = 9
9 -> 7 = 10
9 -> 8 = 11
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 28.2 - What is the famous Seven Bridges of Knigsberg...Ch. 28.2 - Prob. 28.2.2CPCh. 28.2 - Prob. 28.2.3CPCh. 28.2 - Prob. 28.2.4CPCh. 28.3 - Prob. 28.3.1CPCh. 28.3 - Prob. 28.3.2CPCh. 28.4 - Prob. 28.4.1CPCh. 28.4 - Prob. 28.4.2CPCh. 28.4 - Show the output of the following code: public...Ch. 28.4 - Prob. 28.4.4CP
Ch. 28.5 - Prob. 28.5.2CPCh. 28.6 - Prob. 28.6.1CPCh. 28.6 - Prob. 28.6.2CPCh. 28.7 - Prob. 28.7.1CPCh. 28.7 - Prob. 28.7.2CPCh. 28.7 - Prob. 28.7.3CPCh. 28.7 - Prob. 28.7.4CPCh. 28.7 - Prob. 28.7.5CPCh. 28.8 - Prob. 28.8.1CPCh. 28.8 - When you click the mouse inside a circle, does the...Ch. 28.8 - Prob. 28.8.3CPCh. 28.9 - Prob. 28.9.1CPCh. 28.9 - Prob. 28.9.2CPCh. 28.9 - Prob. 28.9.3CPCh. 28.9 - Prob. 28.9.4CPCh. 28.10 - Prob. 28.10.1CPCh. 28.10 - Prob. 28.10.2CPCh. 28.10 - Prob. 28.10.3CPCh. 28.10 - If lines 26 and 27 are swapped in Listing 28.13,...Ch. 28 - Prob. 28.1PECh. 28 - (Create a file for a graph) Modify Listing 28.2,...Ch. 28 - Prob. 28.3PECh. 28 - Prob. 28.4PECh. 28 - (Detect cycles) Define a new class named...Ch. 28 - Prob. 28.7PECh. 28 - Prob. 28.8PECh. 28 - Prob. 28.9PECh. 28 - Prob. 28.10PECh. 28 - (Revise Listing 28.14, NineTail.java) The program...Ch. 28 - (Variation of the nine tails problem) In the nine...Ch. 28 - (4 4 16 tails problem) Listing 28.14,...Ch. 28 - (4 4 16 tails analysis) The nine tails problem in...Ch. 28 - (4 4 16 tails GUI) Rewrite Programming Exercise...Ch. 28 - Prob. 28.16PECh. 28 - Prob. 28.17PECh. 28 - Prob. 28.19PECh. 28 - (Display a graph) Write a program that reads a...Ch. 28 - Prob. 28.21PECh. 28 - Prob. 28.22PECh. 28 - (Connected rectangles) Listing 28.10,...Ch. 28 - Prob. 28.24PECh. 28 - (Implement remove(V v)) Modify Listing 28.4,...Ch. 28 - (Implement remove(int u, int v)) Modify Listing...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In C++ please make a program that calculates the sum of two polynomials using LINKED LINEAR LISTS similar working interface that shows in the given image. (I have posted the question but the working interface isn't suitable please help) (DataStructure)arrow_forwardAll codes should be in c++ or carrow_forwardMissing Brackets Checking: Write a technique to find on the off chance that an articulation has missing sections or not utilizing StackLinkedList. The technique takes a String articulation as a boundary and return True on the off chance that the articulation's sections are right and Falsearrow_forward
- ] ] is_bipartite In the cell below, you are to write a function "is_bipartite (graph)" that takes in a graph as its input, and then determines whether or not the graph is bipartite. In other words, it returns True if it is, and False if it is not. After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (is_bipartite({"A" : ["B", "C"], "B" : ["A"], "C" : ["A"]}), is_bipartite({"A" : ["B", "C"], "B" : ["A", "C"], "C" : ["A", "B"]})) This should return True False Python Pythonarrow_forwardwrite this code using c++ or python plzarrow_forwardUse c++ 1. A Red-Black tree is said to be beautiful if all nodes in the alternate levels of the tree are of the same color. Write a program to check whether a Red-Black tree created from the given input is beautiful or not. Your program should include the following functions. • INSERTREDBLACK (struct node* root, key): Inserts a new node with the 'key' into the tree. • CHECKBEAUTIFUL (struct node* root): Checks whether the given tree is beautiful or not. Input format: Output format: • The output will be 1 if the Red-Black tree is beautiful else -1. Sample Input 1: 9 12 • The first line of input will be an integer 'n' which is the number of nodes in the tree. • After which 'n' integer inputs will be given subsequently which will be the keys of nodes of the tree. The keys are unique and values are in the range [1,1000]. 8 25 3 5 27 22 32 9 Sample Output 1: 1arrow_forward
- Problem 3: In classroom, we implemented MyStack by including an ArrayList as private data field of the class (using composition). In this problem, we will use another way to implement the stack class. Define a new MyStack class that extends ArrayList. Draw the UML diagram for the classes and then implement MyStack. Write a test program that prompts the user to enter five strings and displays them in reverse order. (1) Your UML diagram: (3)arrow_forwardCan someone help me with this? C++ programming please! Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the…arrow_forwardnot sure how to do this problem output doesnt matterarrow_forward
- This is a pyhton , Coding the screenshot better explains. It talks about listarrow_forward8-Write a procedure that takes a tree (represented as a list) and returns a list whose elements are all the leaves of the tree arranged in right to left order or left to right. For example, ( leaves '((1 2) ((3 4) 5 )) returns (1 2 3 4 5) or (5 4 3 2 1). Then, Manually trace your procedure with the provided example.arrow_forwardComplete using Standard C programming. Implement a singly linked list that performs the following: Displays the maximum value in the linked list using recursion. Displays the linked list in reverse order using recursion. Merge two single linked lists and display.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
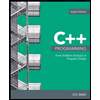
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning