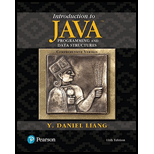
Show the output of the following code:
public class Test {
public static void main(String[] args) {
Graph<Character> graph = new UnweightedGraph<>();
graph.addVertex(‘U’);
graph.addVertex(‘V’);
int indexForU = graph.getIndex(‘U’);
int indexForV = graph.getlndex(‘V’);
System.out.println (“indexForU is ” + indexForU);
System.out.println(“indexForV is ” + indexForV);
graph.addEdge(indexForU, indexForV);
System.out.println(“Degree of U is ” +
graph.getDegree(indexForU));
System.out.println{“Degree of V is ” +
graph.getDegree(indexForV));
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Absolute Java (6th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Modern Database Management (12th Edition)
- Using the graph in the question: Write Java code to create an Adjacency Matrix M to represent the graph. Write Java code to create an Adjacency List L to represent the graph.arrow_forwardJavaarrow_forwardIn C++ QUESTION 14 class rectangleType { public: void setLengthWidth(double x, double y); //Sets the length = x; width = y; void print() const; //Output length and width double area(); //Calculate and return the area of the rectangle (length*width) double perimeter(); //Calculate and return the perimeter (length of outside boundary of the rectangle) private: double length; double width; }; Write the function definition for the function perimeterarrow_forward
- TranposeGraph import java.io.*; import java.util.*; // This class represents a directed graph using adjacency list // representation class ALGraph{ private int vertices; // No. of vertices // Array of lists for Adjacency List Representation private LinkedList adj[]; // Constructor ALGraph(int vertices) { this.vertices = vertices; adj = new LinkedList[this.vertices]; for (int i=0; i getAdjacentList(int v) { return adj[v]; } //Function to add an edge into the graph void addEdge(int v, int w) { adj[v].add(w); // Add w to v's list. } // TODO Transpose graph // If the graph includes zero vertices, return null // Create a new ALGraph // For every vertex, retrieve its adjacent list, make a pass over the list and rewrite each edge (u, v) to be (v, u) and add the u into the adjacent list of v public ALGraph transpose(){…arrow_forwardPlease help with this Java program, and include explanations and commentsarrow_forwardCourse: Data Structure and Algorithims Language: Java Kindly make the program in 2 hours. Task is well explained. You have to make the proogram properly in Java and attach output screen with program: Restriction: Prototype cannot be change you have to make program by using given prototype. TAsk: classBSTNode { Node left; Node right; int data; publicBSTNode(int _data);// assign data to_data and assign left and right node to null } class BST { BSTNoderoot; public BST();// assign root to null void insert(int data);// this function insert the data in tree which maintain property of BST boolean Search(int key); this function search the data in bst and return true if key is found else return false public void EvenPrint(Node n)// this function only print the data which are even, make this function resursive public void OddPrint(Node n)// this function only print the data which are odd, make this function resursive public void PrimePrint(Node n)// this function only…arrow_forward
- Explain the following code : public static void printLeafNodes(TreeNode node) { // base case if (node == null) { return; } if (node.left == null && node.right == null) { System.out.printf("%d ", node.value); } printLeafNodes(node.left); printLeafNodes(node.right); }arrow_forwardclass Node{int data;Node next;public Node (int d) {this(d,null);} public Node (int d,Node n) {data=d;next=n;}}public class List {private Node head; public List() {head=null;}public void addBegenning(int d) {Node n= new Node(d);if (head==null) {head=n;}else {n.next=head;head=n;}}public void addEnd(int d) {if (head==null) {head=new Node(d);}else {Node tmp=head;while(tmp.next!=null)tmp=tmp.next;tmp.next=new Node(d);} }public String toString() {Node tmp=head;String ans="";while(tmp!=null) {ans+=tmp.data+"-->";tmp=tmp.next;}return ans;}public void deleteBegging() {if (head!=null) {head=head.next;}}public void deleteEnd() {Node tmp=head;Node prev= null;while(tmp.next!=null) {prev=tmp;tmp=tmp.next;}prev.next=null;} public boolean contains(int d) {Node tmp=head;while (tmp!=null) {if (tmp.data==d)return true;tmp=tmp.next;}return false; }public void Remove(int d) {//if the d not exsist Node tmp=head;Node prev= null;while (tmp.data!=d) {// tmp!=null…arrow_forwardHi i need an implementation on the LinkListDriver and the LinkListOrdered for this output : Enter your choice: 1 Enter element: Mary List Menu Selections 1. add element 2_remove element 3.head element 4. display 5.Exit Enter your choice: 3 Element @ head: Mary List Menu Selections 1-add element 2-remove element 3_head element 4.display 5-Exit Enter your choice: 4 1. Mary List Menu Selections 1. add element 2. remove element 3.head element 4. display 5. Exit Enter your choice: LinkedListDriver package jsjf; import java.util.LinkedList; import java.util.Scanner; public class LinkedListDriver { public static void main(String [] args) { Scanner input = new Scanner(System.in); LinkedList list = new LinkedList(); int menu = 0; do { System.out.println("\nList menu selection\n1.Add element\n2.Remove element\n3.Head\n4.Display\n5.Exit"); System.out.println();…arrow_forward
- class Queue { private static int front, rear, capacity; private static int queue[]; Queue(int c) { front = rear = 0; capacity = c; queue = new int[capacity]; } static void queueEnqueue(int data) { if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } else { queue[rear] = data; rear++; } return; } static void queueDequeue() { if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } else { for (int i = 0; i < rear - 1; i++) { queue[i] = queue[i + 1]; } if (rear < capacity) queue[rear] = 0; rear--; } return; } static void queueDisplay() { int i; if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } for (i = front; i < rear; i++) { System.out.printf(" %d <-- ", queue[i]); } return; } static void queueFront() { if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } System.out.printf("\nFront Element is: %d", queue[front]);…arrow_forwarddata structures in javaarrow_forwardIn C++ language. Sample run should be exact as the one on image. Thanksarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
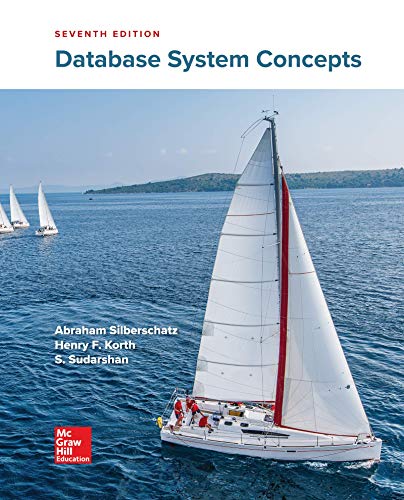
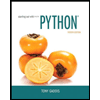
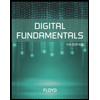
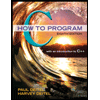
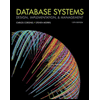
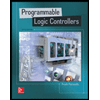