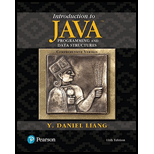
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 28, Problem 28.25PE
(Implement remove(V v)) Modify Listing 28.4, UnweightedGraph.java, to override the remove(V v) method defined in the Graph interface.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2.68♦♦
Write code for a function with the following prototype:
* Mask with least signficant n bits set to 1
* Examples: n = 6 -> 0x3F, n = 17-> 0x1FFFF
* Assume 1 <= n <= w
int lower_one_mask (int n);
Your function should follow the bit-level integer coding rules
Be careful of the case n = W.
Hi-Volt Components
You are the IT manager at Hi-Voltage Components, a medium-sized firm that makes specialized circuit
boards. Hi-Voltage's largest customer, Green Industries, recently installed a computerized purchasing sys-
tem. If Hi-Voltage connects to the purchasing system, Green Industries will be able to submit purchase
orders electronically. Although Hi-Voltage has a computerized accounting system, that system is not
capable of handling EDI.
Tasks
1. What options does Hi-Voltage have for developing a system to connect with Green Industries' pur-
chasing system?
2. What terms or concepts describe the proposed computer-to-computer relationship between
Hi-Voltage and Green Industries?
why not?
3. Would Hi-Voltage's proposed new system be a transaction processing system? Why or
4. Before Hi-Voltage makes a final decision, should the company consider an ERP system? Why or
why not?
Consider the following expression in C: a/b > 0 && b/a > 0.What will be the result of evaluating this expression when a is zero? What will be the result when b is zero? Would it make sense to try to design a language in which this expression is guaranteed to evaluate to false when either a or b (but not both) is zero? Explain your answer
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 28.2 - What is the famous Seven Bridges of Knigsberg...Ch. 28.2 - Prob. 28.2.2CPCh. 28.2 - Prob. 28.2.3CPCh. 28.2 - Prob. 28.2.4CPCh. 28.3 - Prob. 28.3.1CPCh. 28.3 - Prob. 28.3.2CPCh. 28.4 - Prob. 28.4.1CPCh. 28.4 - Prob. 28.4.2CPCh. 28.4 - Show the output of the following code: public...Ch. 28.4 - Prob. 28.4.4CP
Ch. 28.5 - Prob. 28.5.2CPCh. 28.6 - Prob. 28.6.1CPCh. 28.6 - Prob. 28.6.2CPCh. 28.7 - Prob. 28.7.1CPCh. 28.7 - Prob. 28.7.2CPCh. 28.7 - Prob. 28.7.3CPCh. 28.7 - Prob. 28.7.4CPCh. 28.7 - Prob. 28.7.5CPCh. 28.8 - Prob. 28.8.1CPCh. 28.8 - When you click the mouse inside a circle, does the...Ch. 28.8 - Prob. 28.8.3CPCh. 28.9 - Prob. 28.9.1CPCh. 28.9 - Prob. 28.9.2CPCh. 28.9 - Prob. 28.9.3CPCh. 28.9 - Prob. 28.9.4CPCh. 28.10 - Prob. 28.10.1CPCh. 28.10 - Prob. 28.10.2CPCh. 28.10 - Prob. 28.10.3CPCh. 28.10 - If lines 26 and 27 are swapped in Listing 28.13,...Ch. 28 - Prob. 28.1PECh. 28 - (Create a file for a graph) Modify Listing 28.2,...Ch. 28 - Prob. 28.3PECh. 28 - Prob. 28.4PECh. 28 - (Detect cycles) Define a new class named...Ch. 28 - Prob. 28.7PECh. 28 - Prob. 28.8PECh. 28 - Prob. 28.9PECh. 28 - Prob. 28.10PECh. 28 - (Revise Listing 28.14, NineTail.java) The program...Ch. 28 - (Variation of the nine tails problem) In the nine...Ch. 28 - (4 4 16 tails problem) Listing 28.14,...Ch. 28 - (4 4 16 tails analysis) The nine tails problem in...Ch. 28 - (4 4 16 tails GUI) Rewrite Programming Exercise...Ch. 28 - Prob. 28.16PECh. 28 - Prob. 28.17PECh. 28 - Prob. 28.19PECh. 28 - (Display a graph) Write a program that reads a...Ch. 28 - Prob. 28.21PECh. 28 - Prob. 28.22PECh. 28 - (Connected rectangles) Listing 28.10,...Ch. 28 - Prob. 28.24PECh. 28 - (Implement remove(V v)) Modify Listing 28.4,...Ch. 28 - (Implement remove(int u, int v)) Modify Listing...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
A(n) _____ is a module that automatically executes when a specific event occurs. a. event handler b. auto modul...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Big data Big data describes datasets with huge volumes that are beyond the ability of typical database manageme...
Management Information Systems: Managing The Digital Firm (16th Edition)
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Distance TraveLed Modification The distance a vehicle travels can be calculated as follows: Distance=SpeedTime ...
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
In what year was Plankalkl designed? In what year was that design published?
Concepts Of Programming Languages
How is the window manager related to the operating system?
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Consider the following expression in C: a/b > 0 && b/a > 0. What will be the result of evaluating this expression when a is zero? What will be the result when b is zero? Would it make sense to try to design a language in which this expression is guaranteed to evaluate to false when either a or b (but not both) is zero? Explain your answer.arrow_forwardWhat are the major threats of using the internet? How do you use it? How do children use it? How canwe secure it? Provide four references with your answer. Two of the refernces can be from an article and the other two from websites.arrow_forwardAssume that a string of name & surname is saved in S. The alphabetical characters in S can be in lowercase and/or uppercase letters. Name and surname are assumed to be separated by a space character and the string ends with a full stop "." character. Write an assembly language program that will copy the name to NAME in lowercase and the surname to SNAME in uppercase letters. Assume that name and/or surname cannot exceed 20 characters. The program should be general and work with every possible string with name & surname. However, you can consider the data segment definition given below in your program. .DATA S DB 'Mahmoud Obaid." NAME DB 20 DUP(?) SNAME DB 20 DUP(?) Hint: Uppercase characters are ordered between 'A' (41H) and 'Z' (5AH) and lowercase characters are ordered between 'a' (61H) and 'z' (7AH) in the in the ASCII Code table. For lowercase letters, bit 5 (d5) of the ASCII code is 1 where for uppercase letters it is 0. For example, Letter 'h' Binary ASCII 01101000 68H 'H'…arrow_forward
- What did you find most interesting or surprising about the scientist Lavoiser?arrow_forward1. Complete the routing table for R2 as per the table shown below when implementing RIP routing Protocol? (14 marks) 195.2.4.0 130.10.0.0 195.2.4.1 m1 130.10.0.2 mo R2 R3 130.10.0.1 195.2.5.1 195.2.5.0 195.2.5.2 195.2.6.1 195.2.6.0 m2 130.11.0.0 130.11.0.2 205.5.5.0 205.5.5.1 R4 130.11.0.1 205.5.6.1 205.5.6.0arrow_forwardAnalyze the charts and introduce each charts by describing each. Identify the patterns in the given data. And determine how are the data points are related. Refer to the raw data (table):arrow_forward
- 3A) Generate a hash table for the following values: 11, 9, 6, 28, 19, 46, 34, 14. Assume the table size is 9 and the primary hash function is h(k) = k % 9. i) Hash table using quadratic probing ii) Hash table with a secondary hash function of h2(k) = 7- (k%7) 3B) Demonstrate with a suitable example, any three possible ways to remove the keys and yet maintaining the properties of a B-Tree. 3C) Differentiate between Greedy and Dynamic Programming.arrow_forwardWhat are the charts (with their title name) that could be use to illustrate the data? Please give picture examples.arrow_forwardA design for a synchronous divide-by-six Gray counter isrequired which meets the following specification.The system has 2 inputs, PAUSE and SKIP:• While PAUSE and SKIP are not asserted (logic 0), thecounter continually loops through the Gray coded binarysequence {0002, 0012, 0112, 0102, 1102, 1112}.• If PAUSE is asserted (logic 1) when the counter is onnumber 0102, it stays here until it becomes unasserted (atwhich point it continues counting as before).• While SKIP is asserted (logic 1), the counter misses outodd numbers, i.e. it loops through the sequence {0002,0112, 1102}.The system has 4 outputs, BIT3, BIT2, BIT1, and WAITING:• BIT3, BIT2, and BIT1 are unconditional outputsrepresenting the current number, where BIT3 is the mostsignificant-bit and BIT1 is the least-significant-bit.• An active-high conditional output WAITING should beasserted (logic 1) whenever the counter is paused at 0102.(a) Draw an ASM chart for a synchronous system to providethe functionality described above.(b)…arrow_forward
- S A B D FL I C J E G H T K L Figure 1: Search tree 1. Uninformed search algorithms (6 points) Based on the search tree in Figure 1, provide the trace to find a path from the start node S to a goal node T for the following three uninformed search algorithms. When a node has multiple successors, use the left-to-right convention. a. Depth first search (2 points) b. Breadth first search (2 points) c. Iterative deepening search (2 points)arrow_forwardWe want to get an idea of how many tickets we have and what our issues are. Print the ticket ID number, ticket description, ticket priority, ticket status, and, if the information is available, employee first name assigned to it for our records. Include all tickets regardless of whether they have been assigned to an employee or not. Sort it alphabetically by ticket status, and then numerically by ticket ID, with the lower ticket IDs on top.arrow_forwardFigure 1 shows an ASM chart representing the operation of a controller. Stateassignments for each state are indicated in square brackets for [Q1, Q0].Using the ASM design technique:(a) Produce a State Transition Table from the ASM Chart in Figure 1.(b) Extract minimised Boolean expressions from your state transition tablefor Q1, Q0, DISPATCH and REJECT. Show all your working.(c) Implement your design using AND/OR/NOT logic gates and risingedgetriggered D-type Flip Flops. Your answer should include a circuitschematic.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
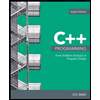
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
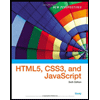
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
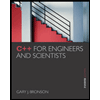
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Java Math Library; Author: Alex Lee;https://www.youtube.com/watch?v=ufegX5o8uc4;License: Standard YouTube License, CC-BY