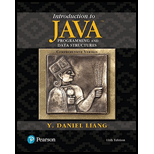
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 28, Problem 28.16PE
Program Plan Intro
Induced subgraph
Program Plan:
- Create a package “main”.
- Add a java class named “Edge” to the package which is used to get the edges from the graph.
- Add a java class named “Graph” to the package which is used to add and remove vertices, edges.
- Add a java class named “UnweightedGraph” to the package which is used to store vertices and neighbors.
- Add a java class named “WeightedGraph” to the package which is used to get the weighted edges and print the edges.
- Add a java class named “WeightedEdge” to the package which is used to compare edges.
- Add a java class named “E16” to the package.
- Import the required packages.
- Declare the main class.
- Give the “main ()” method.
- Declare the string array that contains the names of the city.
- Declare an integer array that contains the weight.
- Create an object for unweighted graph.
- Print the size of the graph, vertex of the graph, index of the vertex “Miami”, and the edges of the graph.
- Call the function “maxInducedSubgraph ()”.
- Print the size of the graph, vertex of the graph, index of the vertex “Miami”, and the edges of the graph.
- Give function definition for “maxInducedSubgraph ()”.
- Declare required variables
- Do until the condition “(!Isdone && g.getSize() > 0)” fails.
- Assign “true” to the variable.
- Loop from 0 through size.
- Check the condition “(g.getDegree(i) < k)”.
- Call the function “remove_Vertex ()”.
- Assign false to the variable.
- Break the loop.
- Check the condition “(g.getDegree(i) < k)”.
- Return the graph.
- Function definition for “UnweightedGraphInducedSubgraph ()”.
- Construct the empty graph.
- Construct a graph from vertices and edges stored in arrays.
- Get the vertices and edges.
- Construct a graph from vertices and edges stored in List.
- Get the vertices and edges.
- Construct a graph for integer vertices 0, 1, 2 and edge list.
- Get the vertices and edges.
- Construct a graph from integer vertices 0, 1, and edge array.
- Get the vertices and edges.
- Give function definition for “remove_Vertex ()”.
- Check the condition “(vertices.contains(v))”.
- Get the index.
- Call the functions “vertices.remove ()”, and “neighbors.remove ()”.
- Loop to remove the edges.
- Loop from 0 through size.
- Check the condition “(list.get(i).v == index)”. If it is true then remove the edge.
- Else, increment the variable
- Loop from 0 through size.
- Loop to reassign the labels.
- Loop from 0 through size.
- Check the condition “(list.get(i).u >= index)”. If the condition is true then get the edge
- Check the condtion “(list.get(i).v >= index)”. If the condition is true then get the edge
- Loop from 0 through size.
- Return “true”.
- Else,
- Return “false”.
- Check the condition “(vertices.contains(v))”.
- Give the “main ()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
answer shoul avoid using AI and should be basic and please explain
Node A is connected to node B by a 2000km fiber link having a bandwidth of 100Mbps.
What is the total latency time (transmit + propagation) required to transmit a 4000 byte file using packets that include
1000 Bytes of data plus 40 Bytes of header.
answer should avoid using AI and should be basic and explain please
Chapter 28 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 28.2 - What is the famous Seven Bridges of Knigsberg...Ch. 28.2 - Prob. 28.2.2CPCh. 28.2 - Prob. 28.2.3CPCh. 28.2 - Prob. 28.2.4CPCh. 28.3 - Prob. 28.3.1CPCh. 28.3 - Prob. 28.3.2CPCh. 28.4 - Prob. 28.4.1CPCh. 28.4 - Prob. 28.4.2CPCh. 28.4 - Show the output of the following code: public...Ch. 28.4 - Prob. 28.4.4CP
Ch. 28.5 - Prob. 28.5.2CPCh. 28.6 - Prob. 28.6.1CPCh. 28.6 - Prob. 28.6.2CPCh. 28.7 - Prob. 28.7.1CPCh. 28.7 - Prob. 28.7.2CPCh. 28.7 - Prob. 28.7.3CPCh. 28.7 - Prob. 28.7.4CPCh. 28.7 - Prob. 28.7.5CPCh. 28.8 - Prob. 28.8.1CPCh. 28.8 - When you click the mouse inside a circle, does the...Ch. 28.8 - Prob. 28.8.3CPCh. 28.9 - Prob. 28.9.1CPCh. 28.9 - Prob. 28.9.2CPCh. 28.9 - Prob. 28.9.3CPCh. 28.9 - Prob. 28.9.4CPCh. 28.10 - Prob. 28.10.1CPCh. 28.10 - Prob. 28.10.2CPCh. 28.10 - Prob. 28.10.3CPCh. 28.10 - If lines 26 and 27 are swapped in Listing 28.13,...Ch. 28 - Prob. 28.1PECh. 28 - (Create a file for a graph) Modify Listing 28.2,...Ch. 28 - Prob. 28.3PECh. 28 - Prob. 28.4PECh. 28 - (Detect cycles) Define a new class named...Ch. 28 - Prob. 28.7PECh. 28 - Prob. 28.8PECh. 28 - Prob. 28.9PECh. 28 - Prob. 28.10PECh. 28 - (Revise Listing 28.14, NineTail.java) The program...Ch. 28 - (Variation of the nine tails problem) In the nine...Ch. 28 - (4 4 16 tails problem) Listing 28.14,...Ch. 28 - (4 4 16 tails analysis) The nine tails problem in...Ch. 28 - (4 4 16 tails GUI) Rewrite Programming Exercise...Ch. 28 - Prob. 28.16PECh. 28 - Prob. 28.17PECh. 28 - Prob. 28.19PECh. 28 - (Display a graph) Write a program that reads a...Ch. 28 - Prob. 28.21PECh. 28 - Prob. 28.22PECh. 28 - (Connected rectangles) Listing 28.10,...Ch. 28 - Prob. 28.24PECh. 28 - (Implement remove(V v)) Modify Listing 28.4,...Ch. 28 - (Implement remove(int u, int v)) Modify Listing...
Knowledge Booster
Similar questions
- answer should avoid using AI (such as ChatGPT), do not any answer directly copied from AI would and explain codearrow_forwardanswer should avoid using AI (such as ChatGPT), do not any answer directly copied from AI would and explain codearrow_forwardWrite a c++ program that will count from 1 to 10 by 1. The default output should be: 1, 2, 3, 4, 5, 6 , 7, 8, 9, 10 There should be only a newline after the last number. Each number except the last should be followed by a comma and a space. To make your program more functional, you should parse command line arguments and change behavior based on their values. Argument Parameter Action -f, --first yes, an integer Change place you start counting -l, --last yes, an integer Change place you end counting -s, --skip optional, an integer, 1 if not specified Change the amount you add to the counter each iteration -h, —help none Print a help message including these instructions. -j, --joke none Tell a number based joke. So, if your program is called counter, counter -f 10 --last 4 --skip 2 should produce 10, 8, 6, 4 Please use the last supplied argument. If your code is called counter, counter -f 4 -f 5 -f 6 should count from 6. You should…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningLINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.Computer ScienceISBN:9781337569798Author:ECKERTPublisher:CENGAGE LEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
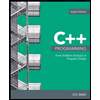
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
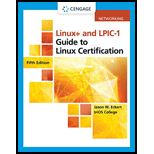
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:9781337569798
Author:ECKERT
Publisher:CENGAGE L
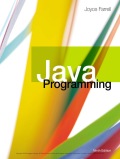
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
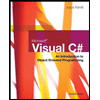
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
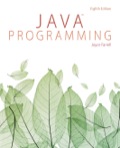
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
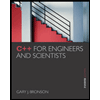
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr