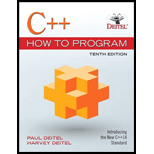
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 19, Problem 19.18E
(Duplicate Elimination) In this chapter, we saw that duplicate elimination is straightforward when creating a binary search tree. Describe how you’d perform duplicate elimination using only a one dimensional array. Compare the performance of array-based duplicate elimination with the performance of binary-search-tree-based duplicate elimination.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(Generic binary search) Implement the following method using binary search. public static > int binarySearch(E[] list, E key)
(Code in Java)
Using the binary search tree (BST) data structure, we can sort a sequence of n elements by first calling aninsertion procedure for n times to maintain a BST, and then performing an Inorder-Tree-Walk on theBST to output the elements in sorted order.The improved insertion procedure will make• 2 comparisons to insert a node with key = 1• 3 comparisons to insert a node with key = 3• 2 comparisons to insert a node with key = 8Program RequirementsIn this programming assignment, you will implement in Java this sorting algorithm using BST, by usingthe improved insertion procedure. Note that each node of a BST is an object containing four attributes:key (for the value of an element), lef t (for its left child), right (for its right child), and p (for its parent).Please add a static counter to track the number of key comparisons made by your algorithm. Your programwill output the following1. The size of the input array,2. The input array,3. The list of array elements after…
(Java) How can I implement a binary search tree in a program to store data, and use the delete method to trim the tree? The program should have a 'populate' button that obtains a string from the user, creates a sorted binary search tree of characters, and prints the tree using the DisplayTree class. It should also print the characters on one line in sorted order by traversing the tree in inorder fashion. The program should also have a 'Trim Tree' button that obtains a second line of input to delete characters from the tree, trimming the tree accordingly. It should ignore characters that are not in the tree, and only delete one character for each occurrence in the second line of input. When all characters from the second line have been deleted from the tree, the program should print the remaining characters in the tree using the DisplayTree class. The output should be labeled appropriately, and no spaces or commas should be used between tree elements in the inorder traversal.Here is the…
Chapter 19 Solutions
C++ How to Program (10th Edition)
Ch. 19 - Prob. 19.6ECh. 19 - Prob. 19.7ECh. 19 - Prob. 19.8ECh. 19 - Prob. 19.9ECh. 19 - Prob. 19.10ECh. 19 - Prob. 19.11ECh. 19 - (Infix-to-Post fix conversion) Stacks are used by...Ch. 19 - Prob. 19.13ECh. 19 - Prob. 19.14ECh. 19 - Prob. 19.15E
Ch. 19 - Prob. 19.16ECh. 19 - Prob. 19.17ECh. 19 - (Duplicate Elimination) In this chapter, we saw...Ch. 19 - Prob. 19.19ECh. 19 - Prob. 19.20ECh. 19 - Prob. 19.21ECh. 19 - Prob. 19.22ECh. 19 - Prob. 19.23ECh. 19 - Prob. 19.24ECh. 19 - Prob. 19.25ECh. 19 - Prob. 19.26ECh. 19 - Prob. 19.27ECh. 19 - Prob. 19.28E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (TRUE or FALSE) The minimum space complexity required to generate all possible subsets of a set with n elements is ALWAYS exponential.arrow_forward(data structor) This sorting algorithm is used for the “A”collection with n positive round members that are less than “k”. Would you please proof the correction of this algorithm using induction; and then say if it is stable or no and why?arrow_forward( Solve it by using Scanner ) Using Binary search tree write a Java program to Insert and print the element in (in-Order traversal ), then search the elements (a and z) in the tree.arrow_forward
- (Java) In this task, a skip list data structure should be implemented. You can follow the followinginstructions:- Implement the class NodeSkipList with two components, namely key node and arrayof successors. You can also add a constructor, but you do not need to add anymethod.- In the class SkipList implement a constructor SkipList(long maxNodes). The parameter maxNodes determines the maximal number of nodes that can be added to askip list. Using this parameter we can determine the maximal height of a node, thatis, the maximal length of the array of successors. As the maximal height of a nodeit is usually taken the logarithm of the parameter. Further, it is useful to constructboth sentinels of maximal height.- In the class SkipList it is useful to write a method which simulates coin flip andreturns the number of all tosses until the first head comes up. This number representsthe height of a node to be inserted.- In the class SkipList implement the following methods:(i) insert, which…arrow_forward3. Largest: a recursive function that computes the largest value for an integer array of positiveand negative values. For example, for the array below, the function largest should return 22,which is the largest value in the array. You can assume there are no more 20 integers in thearray. Think of how to formulate the recurrence relation in this problem yourself.arrow_forwardCan I get help with this question (Java language)arrow_forward
- (In java) In this task, a binomial heap should be implemented. A binomial heap is implemented byan array with its elements being binomial trees: on the first place there is the binomial treeB0 with one element, on the second place there is the binomial tree B1 with two elements,on the third place there is the binomial tree B2 with four elements,. . . Each binomial treeis implemented recursively using the class BinomialNode. In this class there is nothing toimplement. Methods in this class are needed for the implementation of the methods in theclass BinomialHeap. More precisely, in the class BinomialHeap you should implement thefollowing methods:-> delMin, which deletes the minimal key from the binomial heap. The method returnstrue, if the minimal key is successfully deleted and false otherwise (if the binomialheap is empty).-> resizeArray, which extends the array. The method is needed, for example, whenyou find out that you need an extra place in your array when inserting new…arrow_forward(In java) In this task, a binomial heap should be implemented. A binomial heap is implemented byan array with its elements being binomial trees: on the first place there is the binomial treeB0 with one element, on the second place there is the binomial tree B1 with two elements,on the third place there is the binomial tree B2 with four elements,. . . Each binomial treeis implemented recursively using the class BinomialNode. In this class there is nothing toimplement. Methods in this class are needed for the implementation of the methods in theclass BinomialHeap. More precisely, in the class BinomialHeap you should implement thefollowing methods:-> delMin, which deletes the minimal key from the binomial heap. The method returnstrue, if the minimal key is successfully deleted and false otherwise (if the binomialheap is empty).-> resizeArray, which extends the array. The method is needed, for example, whenyou find out that you need an extra place in your array when inserting new…arrow_forward(IN JAVA) In this task, a binomial heap should be implemented. A binomial heap is implemented byan array with its elements being binomial trees: on the first place there is the binomial treeB0 with one element, on the second place there is the binomial tree B1 with two elements,on the third place there is the binomial tree B2 with four elements,. . . Each binomial treeis implemented recursively using the class BinomialNode. In this class there is nothing toimplement. Methods in this class are needed for the implementation of the methods in theclass BinomialHeap. More precisely, in the class BinomialHeap you should implement thefollowing methods:(i) insert, which takes an integer (a key) and inserts it in the binomial heap. Themethod returns true, if the key is successfully inserted and false otherwise. In thecase, if the array should be resized, use the method resizeArray (see below).(ii) getMin, which returns the minimal key in binomial heap. The implementation willbe also efficient…arrow_forward
- (In java) In this task, a binomial heap should be implemented. A binomial heap is implemented byan array with its elements being binomial trees: on the first place there is the binomial treeB0 with one element, on the second place there is the binomial tree B1 with two elements,on the third place there is the binomial tree B2 with four elements,. . . Each binomial treeis implemented recursively using the class BinomialNode. In this class there is nothing toimplement. Methods in this class are needed for the implementation of the methods in theclass BinomialHeap. More precisely, in the class BinomialHeap you should implement thefollowing methods:(i) insert, which takes an integer (a key) and inserts it in the binomial heap. Themethod returns true, if the key is successfully inserted and false otherwise. In thecase, if the array should be resized, use the method resizeArray (see below).(ii) getMin, which returns the minimal key in binomial heap. The implementation willbe also efficient…arrow_forwardShort Answer Questions (3-4 sentences expected per question)1) Give a brief description of the three different loop types (for, while, do-while). When iseach loop type most useful? Are there certain problems that can only be solved withone type of loop?2) When we pass an integer into a function as an argument, the value gets copied into theparameter. When we pass an array of integers into a function, does every array elementalso get copied? Why or why not?3) There are several reasons we might choose to write a user-function in our program,instead of just using a the main() function. Give two benefits of writing our ownfunctions and explain each in a sentence or two.4) In C, we have a special character (NULL character, ‘\0’) for indicating the end of astring. Would it make sense to have a similar special integer value to indicate the end ofinteger arrays? Why or why not?arrow_forward(Sparse matrix–vector product) Recall from Section 3.4.2 that a matrix is said to be sparse if most of its entries are zero. More formally, assume a m × n matrix A has sparsity coefficient γ(A) ≪ 1, where γ(A) ≐ d(A)/s(A), d(A) is the number of nonzero elements in A, and s(A) is the size of A (in this case, s(A) = mn). 1. Evaluate the number of operations (multiplications and additions) that are required to form the matrix– vector product Ax, for any given vector x ∈ Rn and generic, non-sparse A. Show that this number is reduced by a factor γ(A), if A is sparse. 2. Now assume that A is not sparse, but is a rank-one modification of a sparse matrix. That is, A is of the form à + uv⊤, where à ∈ Rm,n is sparse, and u ∈ Rm, v ∈ Rm are given. Devise a method to compute the matrix–vector product Ax that exploits sparsity.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
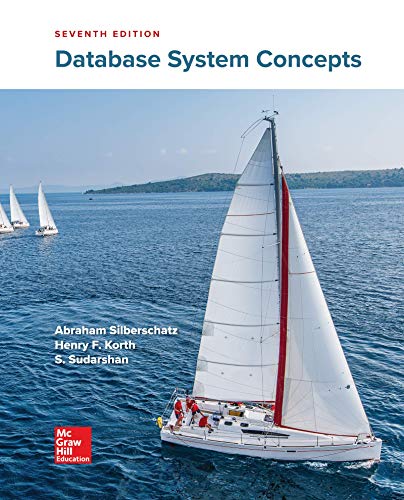
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
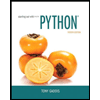
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
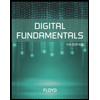
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
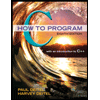
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
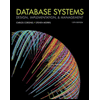
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
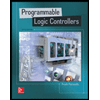
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation; Author: Jenny's lectures CS/IT NET&JRF;https://www.youtube.com/watch?v=AT14lCXuMKI;License: Standard YouTube License, CC-BY
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License