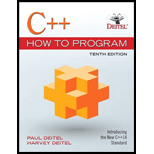
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 19.26E
Program Plan Intro
Program Plan:
This Program defines following structure to represent a List Node in Linked List
structlist_node {
int data;
structlist_node *next;
}node;
We have defined functions to add element at beginning and last of the list.
And to remove element from beginning and last.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
cout<<"List after isolation: ";
display(head);.
Topic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. (See attached photo for reference)
void isEmpty()
This method will return true if the linked list is empty, otherwise return false.
void clear()
This method will empty your linked list. Effectively, this should and already has been called in your destructor (i.e., the ~LinkedList() method) so that it will deallocate the nodes created first before deallocating the linked list itself.
Use C++ Programming language:
Design and implement your own linked list class to hold a sorted list of integers in ascending order. The class should have member functions for inserting an item in the list (in ascending order), deleting an item from the list, and searching the list for an item. Note: the search function should return the position of the item in the list (first item at position 0) and -1 if not found.
In addition, it should have member functions to display the list, check if the list is empty, and return the length of the list. Be sure to have a class constructor a class destructor, and a class copy constructor for deep copy. Demonstrate your class with a driver program (be sure to include the following cases: insertion at the beginning, end (note that the list should alway insert in ascending order. However, in your test include a case where the inserted item goes at the beginning of the list), and inside the list, deletion of first item, last item, and an item…
Chapter 19 Solutions
C++ How to Program (10th Edition)
Ch. 19 - Prob. 19.6ECh. 19 - Prob. 19.7ECh. 19 - Prob. 19.8ECh. 19 - Prob. 19.9ECh. 19 - Prob. 19.10ECh. 19 - Prob. 19.11ECh. 19 - (Infix-to-Post fix conversion) Stacks are used by...Ch. 19 - Prob. 19.13ECh. 19 - Prob. 19.14ECh. 19 - Prob. 19.15E
Ch. 19 - Prob. 19.16ECh. 19 - Prob. 19.17ECh. 19 - (Duplicate Elimination) In this chapter, we saw...Ch. 19 - Prob. 19.19ECh. 19 - Prob. 19.20ECh. 19 - Prob. 19.21ECh. 19 - Prob. 19.22ECh. 19 - Prob. 19.23ECh. 19 - Prob. 19.24ECh. 19 - Prob. 19.25ECh. 19 - Prob. 19.26ECh. 19 - Prob. 19.27ECh. 19 - Prob. 19.28E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Question #7. SORTED LIST NOT UNSORTED C++ The specifications for the Sorted List ADT state that the item to be deleted is in the list. • Rewrite the specification for Deleteltem so that the list is unchanged if the item to be deleted is not in the list. • Implement Deleteltem as specified in (a) using an array-based. Implement Deleteltem as specified in (a) using a linked implementation. • Rewrite the specification for Deleteltem so that all copies of the item to be deleted are removed if they exist. • Implement Deleteltem as specified in (d) using an array-based. • Implement Deleteltem as specified in (d) using a linked implementation.arrow_forwardTopic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. (See attached photo for reference) int count(int num) This will return the count of the instances of the element num in the list. In the linked list in removeAll method, having the method count(10) will return 3 as there are three 10's in the linked list. Additionally, you are to implement these methods: bool move(int pos1, int pos2) This method will move the element at position pos1 to the position specified as pos2 and will return true if the move is successful. This will return false if either the specified positions is invalid. In the example stated, operating the method move(1, 3) will move the 1st element and shall now become the 3rd element. The linked list shall now look like: 30 -> 40 -> 10 -> 50 int removeTail() This method will remove the last element in your linked list and return the said element. If the list is empty, return 0. You can use the…arrow_forwardQUESTION: NOTE: This assignment is needed to be done in OOP(c++/java), the assignment is a part of course named data structures and algorithm. A singly linked circular list is a linked list where the last node in the list points to the first node in the list. A circular list does not contain NULL pointers. A good example of an application where circular linked list should be used is a items in the shopping cart In online shopping cart, the system must maintain a list of items and must calculate total bill by adding amount of all the items in the cart, Implement the above scenario using Circular Link List. Do Following: First create a class Item having id, name, price and quantity provide appropriate methods and then Create Cart/List class which holds an items object to represent total items in cart and next pointer Implement the method to add items in the array, remove an item and display all items. Now in the main do the following Insert Items in list Display all items. Traverse…arrow_forward
- Do it in C++ template (STL)arrow_forwardThe code below is for: (Programming language is C) a. Create a sorted linked list using tenStudent array (copy from array into the linked list will be done). b. Append an element to the end of a list c. Delete the last element from a list. d. Delete the nth element from a list. >>>>>>>>>>>>>>>>>>>>>>>>>> I need to complete the code to do : 1. Using the linked list which is populated at step a, Create a binary tree. The new tree will be created during the deletion of the linked list. The rest of question details in picture.. (Programming language is C) .. thank you The Code: #include<stdio.h>#include<stdlib.h> struct student{int TC;char F_name[12];char L_name[12];int age;char gender[2];};struct student tenStudent[10] =…arrow_forwarddata structurearrow_forward
- Question 6 Linked Lists lend themselves easily to recursive solutions. and it is common to traverse a list by processing a single element and then recursively calling the function on the remaining sub-list. Il does this by accepting a pointer to the current position in the list es a parameter. In the answers below. this has been referred to as the list pointer. what criteria is oten used as the base case that ends the recursion and allos the function to return to he original cal? The data element of the nade pointed to by the list pointer is storing-1. The list pointer points to the beginning of the ist. The list pointer is NULL None of the above. Question 7 when designing the Node structure for our linked list which attributes should we include? Select all that apply. A deta element to store whatever the list is supposed to store. A Node pointer to serve as the head of the list. © A Node pointer to the next element in the list. None of the above.arrow_forwardC++arrow_forward7) Consider a Linked List class called LL, that has a Node pointer (Node*) called head. A Node is a struct composed of an integer element called value and a Node pointer to the next Node called next. The last Node's next is nullptr. Fill in functions A and B below. Note: You do NOT need to implement other functions of LL. struct Node { int value; Node* next; } class LL { private: Node* head; public: void insertAtFront(int num); void deleteRear(int num); }; // Function A: insert a node with value num at the front of the list. void LL:insertAtFront(int num) { //provide your code here } /* Function B: delete the last node in the list. If the list is empty when this is called, print an error message (çcout) stating so instead of deleting anything. Your function must not cause a memory leak! */ void LL::deleteRear(int num) // insert a node with value num at the front of the list. { //provide your code herearrow_forward
- 6. Write a function DOT-PRODUCT that takes two lists, each list has the same number of elements, and produces the dot product of the vectors that they represent. For example, (DOT-PRODUCT '(1.2 2.0 -0.2) '(0.0 2.3 5.0)) -> 3.6 Hint: The key for designing a lisp function is using recursion.arrow_forwardUse C++arrow_forwardkindly don't copy the code from other websites because it's incorrectarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
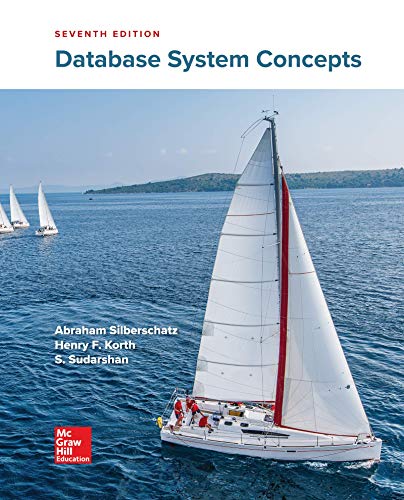
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
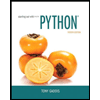
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
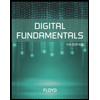
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
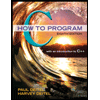
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
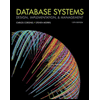
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
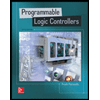
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education