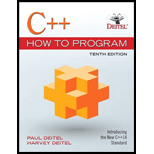
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please be thorough with explanation
(a) Consider implementing a stack as a class that relies on a singly linked list, maintaining pointers to both the start and end of the singly linked list in the main object. You consider two possibilities. The first is to push (insert) to the beginning and pop (remove) from the beginning of the linked list. The second is to push to the end and pop from the end of the linked list. Is one of these possibilities better than the other? Briefly explain your answer.
This in c++.
Question 2: Linked List Implementation
You are going to create and implement a new Linked List class. The Java Class name is
"StringLinkedList". The Linked List class only stores 'string' data type. You should not change the
name of your Java Class. Your program has to implement the following methods for the
StringLinked List Class:
1. push(String e) - adds a string to the beginning of the list (discussed in class)
2. printList() prints the linked list starting from the head (discussed in class)
3. deleteAfter(String e) - deletes the string present after the given string input 'e'.
4. updateToLower() - changes the stored string values in the list to lowercase.
5. concatStr(int p1, int p2) - Retrieves the two strings at given node positions p1 and p2. The
retrieved strings are joined as a single string. This new string is then pushed to the list
using push() method.
Chapter 19 Solutions
C++ How to Program (10th Edition)
Ch. 19 - Prob. 19.6ECh. 19 - Prob. 19.7ECh. 19 - Prob. 19.8ECh. 19 - Prob. 19.9ECh. 19 - Prob. 19.10ECh. 19 - Prob. 19.11ECh. 19 - (Infix-to-Post fix conversion) Stacks are used by...Ch. 19 - Prob. 19.13ECh. 19 - Prob. 19.14ECh. 19 - Prob. 19.15E
Ch. 19 - Prob. 19.16ECh. 19 - Prob. 19.17ECh. 19 - (Duplicate Elimination) In this chapter, we saw...Ch. 19 - Prob. 19.19ECh. 19 - Prob. 19.20ECh. 19 - Prob. 19.21ECh. 19 - Prob. 19.22ECh. 19 - Prob. 19.23ECh. 19 - Prob. 19.24ECh. 19 - Prob. 19.25ECh. 19 - Prob. 19.26ECh. 19 - Prob. 19.27ECh. 19 - Prob. 19.28E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Using java, write an easier version of a linked list with only a couple of the normal linked list functions and the ability to generate and utilize a list of ints. The data type of the connection to the following node can be just Node, and the data element can be just an int. You will need a reference like (Java) or either a reference to the first node, as well as one to the last node. (Answer the following questions) 1) Create a method or function that accepts an integer, constructs a node with that integer as its data value, and then includes the node to the end of the list. If the new node is the first one, this function will also need to update the reference to the first node. This function will need to update the reference to the final node. Consider how to insert the new node following the previous last node, and keep in mind that the next reference for the list's last node should be null. 2) Create a different method or function that iteratively explores the list, printing…arrow_forwardPlease use java code and create a program that completes the task below.arrow_forwardProgramming language: Java Topic: linked listarrow_forward
- This one in c++.arrow_forwardPlease help with this. I really needed help.arrow_forwardCreate a C++ linked list class for integers. Appending, inserting, and removing nodes should be class member functions. Add a list destructor. Drive the class. Add a print function to your linked list class. Display all linked list values. Start with an empty list, add elements, and print the list to test the class.arrow_forward
- You will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardDo the whole code in C++ and please share the full code.arrow_forwardUse Python for this question. Also please comment what each line of code means for this question as well: Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!) Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. push() – take an item as input and push it on the top of the stack pop() – remove and return the item at the top of the stack isEmpty() – returns True if the stack is empty,…arrow_forward
- Provide me complete and correct solution thanks 3arrow_forwardFill in the blanks in each of the following statements (CHOOSE ONLY TEN): 5 AP 1. An --- is created to store information of the method such as the parameters and (0- local variables specific to a given call of the method, and information about which command in the body of the method is currently executing. 21. One application of stack is 32. The type of linked list which is contain more memory space is 3. The --------- operation return the top item of stack. 54. The method used to removes beginning and ending spaces of string 6 5. stack is said underflow when 6. String objects are stored in a special memory area known as 7. Two dimensional array that have a different length is called ----------- A 8. One way to create string object is -------- 10 9. is known as expression. 110. Example of none linear data structures is 12 11. A is a method that contains a statement that makes a call to itself. 13 12. One application of queue isarrow_forwardPlease help me to finish this code in pythonarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
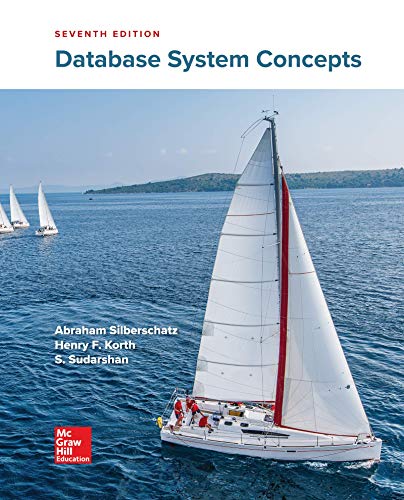
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
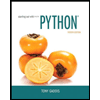
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
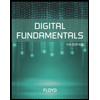
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
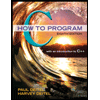
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
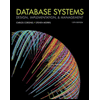
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
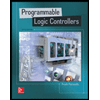
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education