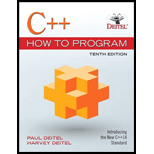
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 19, Problem 19.8E
Program Plan Intro
- Include required header files
- Create class list. Define structure , create its members intdata and node *next. Declare pointer to structure *start
- Define one constructor
- Define member functions input, display, addNode and addition
- Create a main function
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
My daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5.
Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?
Q4 For the network of Fig. 1.41:
a- Determine re
b- Find Aymid
=VolVi
=Vo/Vi
c- Calculate Zi.
d- Find Ay
smid
e-Determine fL, JLC, and fLE
f-Determine the low cutoff frequency.
g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e).
h-Sketch the low-frequency response for the amplifier using the results of part (f).
Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz.
14V
15.6ΚΩ
68kQ
0.47µF
Vo
0.82 ΚΩ
V₁
B-120
3.3kQ
0.47µF
10kQ
1.2k0
=20µF
Z₁
Fig. 1.41 Circuit for
a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table
shown below:
A
B
C
Y
0
0
0
1
0
0
1
0
0
1
0
0
0
1
1
0
1
0
0
1
1
0
1
0
1
1
1
1
0
1
1
0
a. [10 pts] Minimize the Boolean equation you obtained in (a).
b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of
the circuit in your report.
Chapter 19 Solutions
C++ How to Program (10th Edition)
Ch. 19 - Prob. 19.6ECh. 19 - Prob. 19.7ECh. 19 - Prob. 19.8ECh. 19 - Prob. 19.9ECh. 19 - Prob. 19.10ECh. 19 - Prob. 19.11ECh. 19 - (Infix-to-Post fix conversion) Stacks are used by...Ch. 19 - Prob. 19.13ECh. 19 - Prob. 19.14ECh. 19 - Prob. 19.15E
Ch. 19 - Prob. 19.16ECh. 19 - Prob. 19.17ECh. 19 - (Duplicate Elimination) In this chapter, we saw...Ch. 19 - Prob. 19.19ECh. 19 - Prob. 19.20ECh. 19 - Prob. 19.21ECh. 19 - Prob. 19.22ECh. 19 - Prob. 19.23ECh. 19 - Prob. 19.24ECh. 19 - Prob. 19.25ECh. 19 - Prob. 19.26ECh. 19 - Prob. 19.27ECh. 19 - Prob. 19.28E
Knowledge Booster
Similar questions
- Using XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forwardQ4) A thin ring of radius 5 cm is placed on plane z = 1 cm so that its center is at (0,0,1 cm). If the ring carries 50 mA along a^, find H at (0,0,a).arrow_forward4. [15 pts] A logic function F of four variables a; b; c; d is described by the following K-map. Derive the fully minimized SOP logic expression form of F. cd ab 00 01 11 10 00 0 0 0 1 01 1 0 0 1 11 1 0 1 1 10 0 0 1 1arrow_forward
- 2. [20 pts] Student A B will enjoy his picnic on sunny days that have no ants. He will also enjoy his picnic any day he sees a hummingbird, as well as on days where there are ants and ladybugs. a. Write a Boolean equation for his enjoyment (E) in terms of sun (S), ants (A), hummingbirds (H), and ladybugs (L). b. Implement in Logisim, the logic circuit of E function. Use the Circuit Analysis tool in Logisim to view the expression, include an image of the expression generated by Logisimarrow_forwardHow would I go about creating this computer database in MariaDB with sql? Create a database name "dbXXXXXX" Select the database using the "use [database name]" command. Now you are in the database. Based on the above schema from Enrolment System database, create all the tables with the last 6 digits of "123456", then the table name for table Lecturer should be "123456_Lecturer". Refer to basic SQL lecture note to create table that has primary keys and Foreign Keys. Provide the datatype of each attributes. Add a column called "Department" with datatype "VARCHAR(12)" to the table "Lecturer". Shows the metadata of the updated "Lecturer" table. (Use Describe command) Drop the "Department" column from the table "Lecturer", and show the metadata of the updated "Lecturer" table. Insert three (3) data to each of the table in the tables created. Note: If you have foreign key issues, please disable foreign key constraints before inserting the data, see below SET FOREIGN_KEY_CHECKS=0;…arrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 3. Consider the real number x = (3.395) 10 (a) (b) Convert the decimal number x into binary format up to 7 binary places (7 binary digits after decimal) Convert the calculated value into denormalized form and calculate fl(x) for m=4 Don't use any Al tool show answer in pen a nd paper then take pi ctures and sendarrow_forward
- Simplify the following expressions by means of a four-variable K-Map. AD+BD+ BC + ABDarrow_forwardCSE330 Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. 2. Let ẞ 2, m = 6, emin = -3 and emax = 3. Answer the following questions: Compute the minimum of |x| for General and Normalized form (a) Compute the Machine Epsilon value for the General and Denormalized form. If we change the value of emax to 6 then how will it affect the value of maximum scale invariant error for the case of Normalized form? Explain your answer. show answer in pen a Don't use any Al tool nd paper then take pi ctures and sendarrow_forwardCSE330: Discrete Mathematics 1. In the classes, we discussed three forms of floating number representations as given below, (1) Standard/General Form, (2) Normalized Form, (3) Denormalized Form. Now, let's take, ẞ = 2, m = 3, emin = -2 and emax = 3. Based on these, answer the following: (a) (b) (c) (d) What are the maximum/largest numbers that can be stored in the system by these three forms defined above? (express your answer in decimal values) What are the non-negative minimum/smallest numbers that can be stored in the system by the denormalized form? (express your answer in decimal values) How many numbers (both non-negative and negative) can be represented in the above mentioned system using the general form? Explain your answer. Find all the decimal numbers for e = 3 and e = 2 in denormalized form, plot them on a real line and prove that all the numbers are not equally spaced. Write the equally spaced sets for the number line you drew. show your answer in Don't use any Al tool pen…arrow_forward
- 3.[20 pts] Find the minimum equivalent circuit for the one shown below (show your work): DAB 0 f(A,B,C,D)arrow_forwardSuppose your computer is responding very slowly to information requests from the Internet. You observe that your network gateway shows high levels of network activity even though you have closed your e-mail client, Web browser, and all other programs that access the Internet. What types of malwares could cause such symptoms? What steps can you take to check whether malware has gained access to your system? What tools can you use at each step? If you identify malware, what ways might it have entered your system? How can you restore your PC to safe operation, including the special software tools you may use?arrow_forwardR languagearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
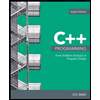
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
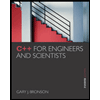
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
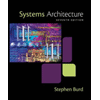
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
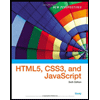
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
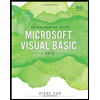
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning