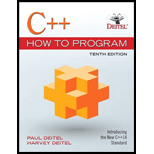
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 19, Problem 19.9E
Program Plan Intro
- Include required header files
- Create a class list, declare structure node with char data and node * next. Create structure variable *start.
- Create main class
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Use c++
Write a program that uses the STL list container to create a linked list of integers. The program will ask the user to provide a list of integers. When the user is finished, the program will list the integers entered by the user and show the average of those integers. Additionally, you need to add the following code:
Display your name (first and last) before you ask the user to enter the integers.
You need to do one of the followings: (do not do all three)
if the first letter of your last name is between and including A through G, compute the maximum value entered by the user
Please code using C++ and only use the headers <iostream> and <limits.h>. The use of any other headers will not be accepted. Thank you!
Task 3: (Advanced operations) in python
Write a function that rotates the elements of the list k times. [You are not allowed to create any other list]. (use linked lists)
Sample Input
Sample Output
3 -> 2 -> 5 -> 1-> 8, left, 2
5 -> 1 -> 8 -> 3 -> 2
3 -> 2 -> 5 -> 1-> 8, right, 2
1 -> 8 -> 3 -> 2 -> 5
Chapter 19 Solutions
C++ How to Program (10th Edition)
Ch. 19 - Prob. 19.6ECh. 19 - Prob. 19.7ECh. 19 - Prob. 19.8ECh. 19 - Prob. 19.9ECh. 19 - Prob. 19.10ECh. 19 - Prob. 19.11ECh. 19 - (Infix-to-Post fix conversion) Stacks are used by...Ch. 19 - Prob. 19.13ECh. 19 - Prob. 19.14ECh. 19 - Prob. 19.15E
Ch. 19 - Prob. 19.16ECh. 19 - Prob. 19.17ECh. 19 - (Duplicate Elimination) In this chapter, we saw...Ch. 19 - Prob. 19.19ECh. 19 - Prob. 19.20ECh. 19 - Prob. 19.21ECh. 19 - Prob. 19.22ECh. 19 - Prob. 19.23ECh. 19 - Prob. 19.24ECh. 19 - Prob. 19.25ECh. 19 - Prob. 19.26ECh. 19 - Prob. 19.27ECh. 19 - Prob. 19.28E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 4arrow_forward1. Write a program to reverse a singly or doubly linked list. (take data as int)2. Write a program to remove duplicates from a doubly linked list. (1,2,3,4,5,2) (you will remove the 2nd duplicate).3. Write a program to swap two data items in a doubly linked list. swap number 2 and number 4 data with each other. course--data structure in c++ perform all three parts in the one cpp file.arrow_forward45- Which of the following statements about lists in Python is true? a. Items within a list are unordered. b. A list can grow in size as items are added to it. c. All items in a list must have the same type. d. Items within a list must be unique. e. Items can only be added to a list by creating a new list object. f. Lists can only contain items which have a primitive type.arrow_forward
- Happy weekend:) I will upvotearrow_forwardAnswer explanation-.arrow_forwardpython help Q9: Sub All Write sub-all, which takes a list s, a list of old words, and a list of new words; the last two lists must be the same length. It returns a list with the elements of s, but with each word that occurs in the second argument replaced by the corresponding word of the third argument. You may use substitute in your solution. Assume that olds and news have no elements in common. (define (sub-all s olds news) 'YOUR-CODE-HERE )arrow_forward
- python: def character_gryffindor(character_list):"""Question 1You are given a list of characters in Harry Potter.Imagine you are Minerva McGonagall, and you need to pick the students from yourown house, which is Gryffindor, from the list.To do so ...- THIS MUST BE DONE IN ONE LINE- First, remove the duplicate names in the list provided- Then, remove the students that are not in Gryffindor- Finally, sort the list of students by their first name- Don't forget to return the resulting list of names!Args:character_list (list)Returns:list>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter, Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "Luna Lovegood, Ravenclaw"])['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger, Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "James Potter, Gryffindor"])['Hermione Granger, Gryffindor',…arrow_forwardpython: def character_gryffindor(character_list):"""Question 1You are given a list of characters in Harry Potter.Imagine you are Minerva McGonagall, and you need to pick the students from yourown house, which is Gryffindor, from the list.To do so ...- THIS MUST BE DONE IN ONE LINE- First, remove the duplicate names in the list provided- Then, remove the students that are not in Gryffindor- Finally, sort the list of students by their first name- Don't forget to return the resulting list of names!Args:character_list (list)Returns:list>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter,Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "LunaLovegood, Ravenclaw"])['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger,Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "JamesPotter, Gryffindor"])['Hermione Granger, Gryffindor', 'James…arrow_forwardWhich of the following statements are correct (assuming O notation)? 1.Deleting an element is slower for a linked list than for an array 2.Random access of elements in an array is O(1) 3.Linked lists are more memory-efficient than arraysarrow_forward
- .arrow_forwardAssume a singly linked list of characters contains the following elements B, C, D, E, F. (Don't write any line of code or algorithm!) 4. (2 marks) Draw the steps of inserting a new element A into first of the above linked list. Draw the steps of removing F from the above linked list.arrow_forwardNeed done in pythonarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
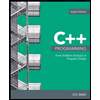
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
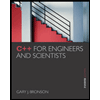
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Introduction to Linked List; Author: Neso Academy;https://www.youtube.com/watch?v=R9PTBwOzceo;License: Standard YouTube License, CC-BY
Linked list | Single, Double & Circular | Data Structures | Lec-23 | Bhanu Priya; Author: Education 4u;https://www.youtube.com/watch?v=IiL_wwFIuaA;License: Standard Youtube License